JavaFX is a versatile and powerful framework for building user interfaces in Java applications. Among its many features, the JavaFX TableView stands out as a highly useful tool for displaying data in a tabular format with added interactivity. In this article, we’ll explore the basics of JavaFX TableView, from setting up the basic structure to populating it with data and handling user interactions.
Setting Up the JavaFX Application
To get started, ensure you have JavaFX installed and set up in your development environment. Create a new JavaFX project, and make sure to include the necessary libraries.
Creating the TableView
The first step is to create a TableView instance. A TableView consists of columns, where each column represents a specific property of the data displayed. To start, let’s create a simple TableView with two columns: “Name” and “Age.” We’ll populate it with some sample data to illustrate the concept.
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Sample data
ObservableList<Person> data = FXCollections.observableArrayList(
new Person("Abel", 3),
new Person("Benard", 6),
new Person("Carl", 9),
new Person("Denis", 12),
new Person("Edward", 15),
new Person("Jane", 18),
new Person("Sam", 21)
);
// TableView and columns
TableView<Person> tableView = new TableView<>();
TableColumn<Person, String> nameColumn = new TableColumn<>("Name");
TableColumn<Person, Integer> ageColumn = new TableColumn<>("Age");
// Define how to get the values from the Person object
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
ageColumn.setCellValueFactory(new PropertyValueFactory<>("age"));
// Add columns to the TableView
tableView.getColumns().addAll(nameColumn, ageColumn);
// Add data to the TableView
tableView.setItems(data);
this.parent.setCenter(tableView);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX TableView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Defining the Data Model
In the example above, we referenced a Person class as the data model for the TableView. Ensure you have a class like this defined, with properties that match the column types:
// Sample Person class
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return this.name;
}
public int getAge() {
return this.age;
}
}
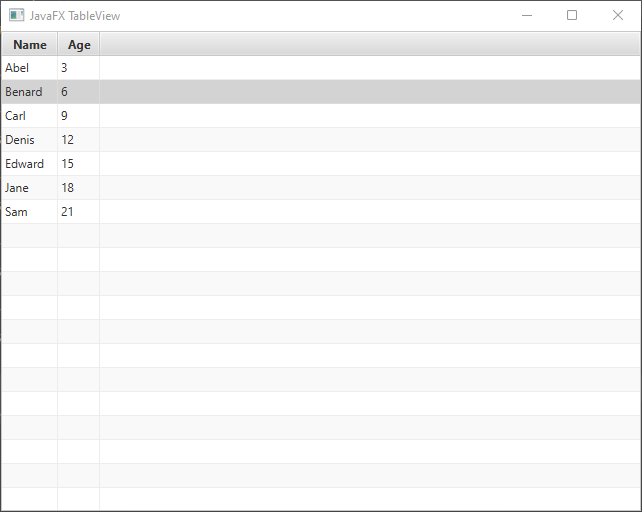
Customizing TableView
JavaFX TableView provides extensive customization options. You can add event handlers, modify cell rendering, and handle user interactions. Let’s explore some of these customization options.
Handling Cell Editing
Enabling cell editing in JavaFX TableView allows users to modify the data directly within the table, making it more interactive and user-friendly. To enable cell editing, you need to set the editable property of the TableView to true. Additionally, you can define event handlers to handle the editing and updating of the data.
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.control.cell.TextFieldTableCell;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import javafx.util.converter.IntegerStringConverter;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Sample data
ObservableList<Person> data = FXCollections.observableArrayList(
new Person("Abel", 3),
new Person("Benard", 6),
new Person("Carl", 9),
new Person("Denis", 12),
new Person("Edward", 15),
new Person("Jane", 18),
new Person("Sam", 21)
);
// TableView and columns
TableView<Person> tableView = new TableView<>();
// Make TableView editable
tableView.setEditable(true);
TableColumn<Person, String> nameColumn = new TableColumn<>("Name");
TableColumn<Person, Integer> ageColumn = new TableColumn<>("Age");
// Define how to get the values from the Person object
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
ageColumn.setCellValueFactory(new PropertyValueFactory<>("age"));
// Enable cell editing for the name column
nameColumn.setCellFactory(TextFieldTableCell.forTableColumn());
// Add an event handler to the name column to update the data when editing is finished
nameColumn.setOnEditCommit(event -> {
// Get selected person
Person person = event.getRowValue();
// Update Person name
person.setName(event.getNewValue());
});
// Enable cell editing for the age column
ageColumn.setCellFactory(TextFieldTableCell.forTableColumn(new IntegerStringConverter() {
@Override
public Integer fromString(String s) {
try {
return super.fromString(s);
} catch(NumberFormatException numberFormatException) {
return 0;
}
}
}));
// Add an event handler to the age column to update the data when editing is finished
ageColumn.setOnEditCommit(event -> {
// Get selected person
Person person = event.getRowValue();
// Update Person age
person.setAge(event.getNewValue());
});
// Add columns to the TableView
tableView.getColumns().addAll(nameColumn, ageColumn);
// Add data to the TableView
tableView.setItems(data);
this.parent.setCenter(tableView);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX TableView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
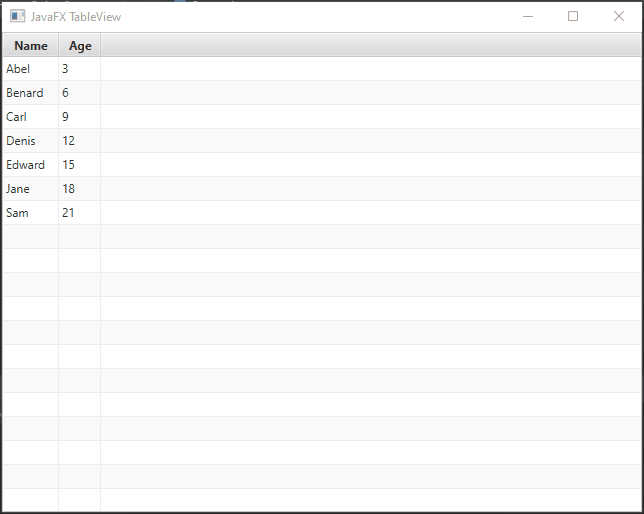
Handling Selection
Listening for selection changes in JavaFX TableView allows you to perform actions based on the user’s selection, which can be useful for displaying additional information, updating other parts of the UI, or triggering specific actions in response to the selected item.
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.control.cell.TextFieldTableCell;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import javafx.util.converter.IntegerStringConverter;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Sample data
ObservableList<Person> data = FXCollections.observableArrayList(
new Person("Abel", 3),
new Person("Benard", 6),
new Person("Carl", 9),
new Person("Denis", 12),
new Person("Edward", 15),
new Person("Jane", 18),
new Person("Sam", 21)
);
// TableView and columns
TableView<Person> tableView = new TableView<>();
// Make TableView editable
tableView.setEditable(true);
TableColumn<Person, String> nameColumn = new TableColumn<>("Name");
TableColumn<Person, Integer> ageColumn = new TableColumn<>("Age");
// Define how to get the values from the Person object
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
ageColumn.setCellValueFactory(new PropertyValueFactory<>("age"));
// Add a selection listener to the TableView
tableView.getSelectionModel().selectedItemProperty().addListener((obs, oldSelection, newSelection) -> {
if (newSelection != null) {
// Print selected Person name and age to the console
System.out.printf("{name: %s, age: %d}%n", newSelection.getName(), newSelection.getAge());
}
});
// Add columns to the TableView
tableView.getColumns().addAll(nameColumn, ageColumn);
// Add data to the TableView
tableView.setItems(data);
this.parent.setCenter(tableView);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX TableView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
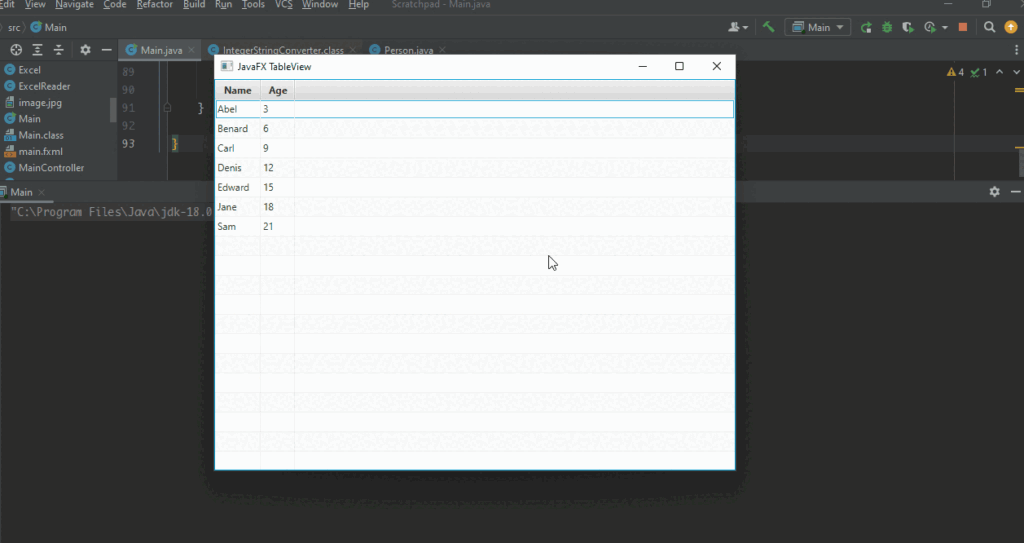
Conclusion
JavaFX TableView is a powerful and flexible component for displaying data in a tabular format within Java applications. With its ability to handle cell editing, selection, and numerous customization options, it empowers developers to build interactive data tables that enhance the user experience. As you delve deeper into JavaFX, you’ll discover even more possibilities to create intuitive and feature-rich user interfaces.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!