In today’s digital age, social sharing is an integral part of online content consumption. Websites and applications are constantly seeking ways to engage users and expand their reach through the power of social media. One effective strategy is to implement a JavaScript social sharing widget that allows users to easily share content with their networks. In this article, we’ll explore two approaches to creating a JavaScript-based social sharing widget: the traditional method and using the Navigator API.
The Importance of Social Sharing
Before diving into the technical aspects of creating a social sharing widget, let’s briefly discuss why it is important for web developers and businesses to incorporate such functionality into their online platforms.
Enhanced Visibility
Social media platforms are a powerful way to increase the visibility of your content. When users share your content on their social networks, it can reach a wider audience, potentially attracting new visitors and customers.
User Engagement
Social sharing encourages user interaction with your content. It allows users to express their appreciation for your content by sharing it with their followers, thereby increasing engagement and building a sense of community around your brand.
SEO Benefits
Social signals, such as the number of shares, likes, and comments, can indirectly impact your website’s search engine rankings. A well-shared piece of content can improve its search visibility.
Traffic Generation
Social sharing can drive additional traffic to your website, leading to more opportunities for conversions, whether it’s signing up for a newsletter, making a purchase, or any other desired action.
Traditional Approach
The traditional approach to creating a social sharing widget involves manually constructing sharing URLs for various social media platforms. Developers create buttons for each platform, and when users click on a button, the corresponding URL is opened in a new window or tab. Here’s an example implementation for sharing a webpage on Facebook, LinkedIn, Twitter, Email and SMS:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Creating a JavaScript Social Sharing Widget</title>
<style>
/* styles.css */
.social-sharing-widget {
display: flex;
gap: 10px;
}
.share-button {
padding: 10px 20px;
background-color: #0073e6;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
.share-button:hover {
background-color: #005bbf;
}
</style>
</head>
<body>
<div class="social-sharing-widget">
<button class="share-button" data-social="facebook">Share on Facebook</button>
<button class="share-button" data-social="twitter">Share on Twitter</button>
<button class="share-button" data-social="linkedin">Share on LinkedIn</button>
<button class="share-button" data-social="email">Share on Email</button>
<button class="share-button" data-social="sms">Share on SMS</button>
</div>
<script>
document.addEventListener("DOMContentLoaded", function () {
const shareButtons = document.querySelectorAll(".share-button");
shareButtons.forEach(function (button) {
button.addEventListener("click", function () {
const socialPlatform = button.getAttribute("data-social");
// You can replace this with the URL you want to share.
const urlToShare = window.location.href;
// Define social media share URLs
const shareUrls = {
facebook: `https://www.facebook.com/sharer/sharer.php?u=${urlToShare}`,
linkedin: `https://www.linkedin.com/shareArticle?url=${urlToShare}`,
twitter: `https://twitter.com/intent/tweet?url=${urlToShare}`,
email: `mailto:?subject=${encodeURIComponent(document.title)}&body=${urlToShare}`,
sms: `sms:?body=${urlToShare}`,
};
// Open a new window to share the content
window.open(shareUrls[socialPlatform], "_blank");
});
});
});
</script>
</body>
</html>
In this example, we create five (5) buttons: one for Facebook, one for LinkedIn, one for Twitter, one for Email, and one for SMS. When a user clicks on one of the buttons, JavaScript constructs the sharing URL using the current webpage’s URL and opens it in a new window or tab, allowing the user to share the content on the chosen platform or through email and SMS.
When a user clicks on the “Share on SMS” button, it will trigger their device’s SMS application, and the body of the SMS will contain the URL. However, it’s important to note that the behavior of such links may vary depending on the user’s device and SMS application, and not all devices and apps may support this method of sharing links.
While this approach works, it can become cumbersome as you add more social networks. It also requires manual updates when social networks change their sharing URL structures.
Using the Navigator API
A more modern and efficient approach involves using the Navigator API, which provides access to the user’s available sharing services. This method simplifies the process of creating a social sharing widget and ensures compatibility with various platforms. Here’s an example implementation:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Creating a JavaScript Social Sharing Widget</title>
<style>
/* styles.css */
.share-button {
padding: 10px 20px;
background-color: #0073e6;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
.share-button:hover {
background-color: #005bbf;
}
</style>
</head>
<body>
<div class="social-sharing-widget">
<button class="share-button">Share</button>
</div>
<script>
document.addEventListener("DOMContentLoaded", function () {
const shareButtons = document.querySelectorAll(".share-button");
shareButtons.forEach(function (button) {
button.addEventListener('click', () => {
// Check if the navigator.share method is available
if (navigator.share) {
navigator.share({
title: document.title,
text: "Check out this article!",
url: window.location.href,
})
.then(() => {
console.log('Shared successfully');
})
.catch((error) => {
console.error('Error sharing:', error);
});
} else {
// Fallback for browsers that do not support navigator.share
alert("Navigator API not supported in this browser.");
}
});
});
});
</script>
</body>
</html>
In this example, we use the navigator.share method provided by the Navigator API. This method opens the native sharing dialog if supported by the user’s browser. Users can then choose from a variety of installed apps and services to share the content. If the Navigator API is not supported, we display an alert message.
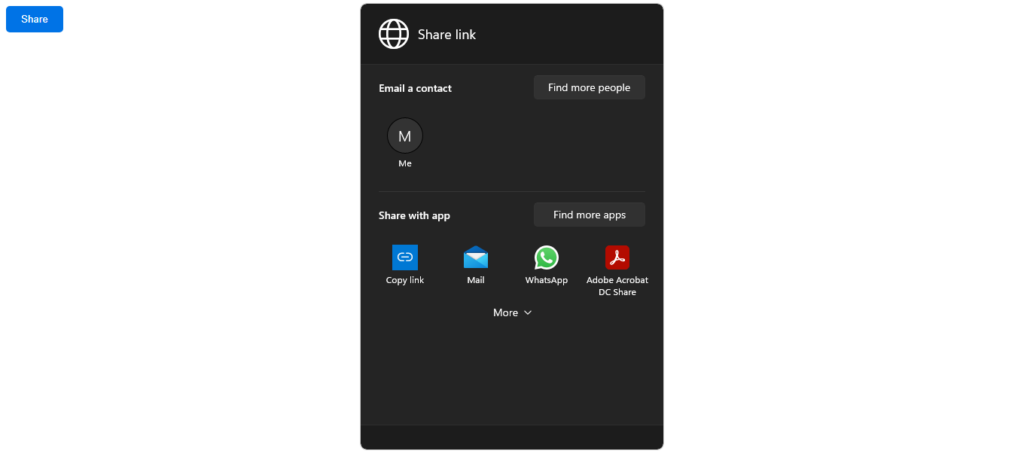
Keep in mind that not all browsers support the navigator.share method, so it’s essential to provide a graceful fallback to ensure a consistent user experience across various platforms.
Conclusion
Both the traditional approach and using the Navigator API have their merits. The traditional approach offers fine-grained control but requires more code and maintenance. On the other hand, the Navigator API simplifies the process, ensures consistency across platforms, and leverages native sharing dialogs, but may not be available in all browsers.
Consider your project’s requirements and your target audience when choosing the method that best suits your needs. Regardless of your choice, implementing a social sharing widget can greatly enhance user engagement and extend the reach of your content.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.