In the world of visual design, blending is a fundamental concept. It allows you to combine two or more graphical elements to create a new and visually appealing composition. In JavaFX, the Blend Effect is a powerful tool that lets you blend two input effects together using a variety of predefined Blend Modes. In this article, we will explore the Blend Effect in JavaFX, understand its core concepts, and create a sample application to demonstrate its usage.
Understanding the JavaFX Blend Effect
The JavaFX Blend Effect is a powerful tool that enables you to blend two different visual elements together, creating unique and compelling compositions. It is a part of the JavaFX library and is used to enhance the visual aesthetics of your user interface. The Blend Effect is particularly useful when you want to overlay or merge multiple graphical components, such as shapes, images, or text, to produce a harmonious and visually striking result.
One of the key features of the Blend Effect is the ability to apply different blending modes to the inputs. A blending mode defines how the colors of the two inputs are combined. JavaFX provides a set of predefined Blend Modes, each with its unique characteristics and applications. To explore the available Blend Modes and their descriptions, you can refer to the official JavaFX BlendMode documentation here.
Creating a Blend Effect Example Application
Now, let’s get hands-on with a practical example of how to use the JavaFX Blend Effect. In this example, we’ll create a simple JavaFX application that demonstrates the Blend Effect with different Blend Modes.
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.effect.*;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class BlendEffect extends Application {
// Application properties
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
// Create the main application scene
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the title for the application window
stage.setTitle("Blend Effect");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
// Create Rectangle Nodes
Rectangle rectangle = new Rectangle(50, 50, 300, 300);
rectangle.setFill(Color.CORNFLOWERBLUE); // Set the Rectangle Fill color
Blend blend = new Blend();
blend.setOpacity(0.8); // Set the Blend Opacity to 0.8
// Create the ColorInput Effect
ColorInput colorInput = new ColorInput(0, 0, 160, 150, Color.MEDIUMPURPLE);
blend.setTopInput(colorInput); // Set the ColorInput as the Blend Top Input
// Create the SepiaTone Effect
SepiaTone sepiaTone = new SepiaTone();
// Set the SepiaTone as the Blend Bottom Input
blend.setBottomInput(sepiaTone);
// Apply the Blend Effect to the Rectangle
rectangle.setEffect(blend);
// Add the Rectangle to the center region of the BorderPane
this.parent.setCenter(rectangle);
// Add the BlendModePanel to the left region of the BorderPane
this.parent.setLeft(new BlendModePanel(blend));
}
static class BlendModePanel extends HBox {
public BlendModePanel(Blend blend) {
super(5);
// Create BlendModes Collection
ObservableList<BlendMode> blendModes = FXCollections.observableArrayList(
BlendMode.values()
);
// Create BlendMode ComboBox and provide the available BlendModes
ComboBox<BlendMode> blendModeComboBox = new ComboBox<>(blendModes);
blendModeComboBox.getSelectionModel().selectFirst(); // Select the first BlendMode
// Bind the Blend modeProperty to the ComboBox valueProperty
blend.modeProperty().bind(blendModeComboBox.valueProperty());
// Create the HBox to arrange the Label and BlendMode ComboBox
this.getChildren().addAll(
new Label(String.format("%-13s", "Blend Mode")),
blendModeComboBox
);
this.setPadding(new Insets(20));
this.setAlignment(Pos.CENTER_LEFT);
}
}
}
In this code, we create a JavaFX application that displays a blue rectangle with a Blend Effect applied to it. The Blend Effect blends a ColorInput effect and a SepiaTone effect. We also provide a user interface to select different Blend Modes using a ComboBox.
The Blend Mode ComboBox allows you to choose from various Blend Modes, each of which will change the way the input effects blend together. This interactive feature demonstrates the flexibility and power of the JavaFX Blend Effect.
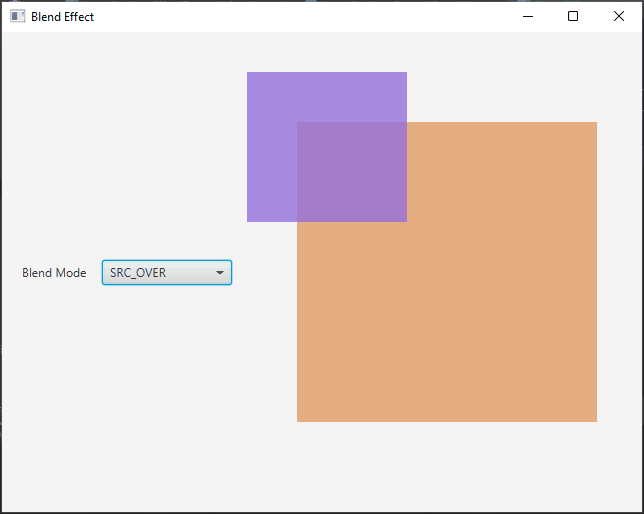
Conclusion
The JavaFX Blend Effect is a powerful tool for creating visually stunning effects in your Java applications. By blending two inputs with different BlendModes, you can achieve a wide range of results, from simple color adjustments to complex visual transformations. This article has provided a practical example of using the Blend Effect, and with a little creativity, you can leverage this feature to enhance the visual appeal of your Java applications.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.