When creating applications with large amounts of content, such as images, text, or tables, it becomes essential to provide a way for users to navigate through the content easily. JavaFX ScrollPane comes to the rescue in such scenarios by allowing us to add scrollbars to our application, enabling smooth scrolling through the content. In this article, we will explore JavaFX ScrollPane in detail and provide code examples to demonstrate its usage.
What is a ScrollPane?
A ScrollPane is a JavaFX container that provides a scrollable view of its content. It is particularly useful when the content inside it exceeds the visible area, and you want to allow users to navigate through the content using scrollbars. The ScrollPane automatically adds horizontal and vertical scrollbars when needed, making it a handy component for displaying large datasets or images.
Benefits of using ScrollPane
Using ScrollPane in your JavaFX applications offers several benefits:
- Efficient Content Handling: ScrollPane handles large content efficiently, enabling smooth navigation through the content without overwhelming the user interface.
- User-Friendly: It provides a familiar and intuitive way for users to view and interact with extensive content in a confined space.
- Flexible Configuration: ScrollPane can be customized to suit your application’s specific needs, including scroll policies, scrollbar visibility, and more.
- Versatile Content Support: You can place various JavaFX nodes inside a ScrollPane, such as images, text, tables, or even complex layouts.
- Responsive Design: ScrollPane automatically adjusts to changes in the content size, making it compatible with various screen sizes and resolutions.
Creating a Basic ScrollPane
Let’s start by creating a basic JavaFX application with a ScrollPane containing some content.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
VBox content = new VBox(10);
for (int i = 1; i <= 50; i++) {
content.getChildren().add(new Label("Item " + i));
}
// Add scrolling capability to the content
ScrollPane scrollPane = new ScrollPane(content);
// Add the scroll pane to the BorderPane layout manager
this.parent.setCenter(scrollPane);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ScrollPane");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we create a VBox container with 50 Label items and place it inside a ScrollPane. When the number of items exceeds the visible area of the ScrollPane, the scrollbars automatically appear, allowing you to scroll through the content.
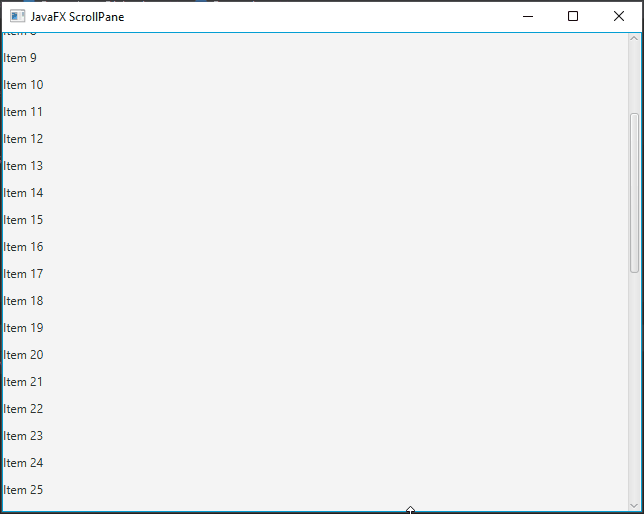
Customizing ScrollPane Behavior
JavaFX ScrollPane provides various options to customize its behavior. For example, you can adjust the scroll position, disable one of the scrollbars, or control how the content is displayed within the viewport.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
VBox content = new VBox(10);
for (int i = 1; i <= 50; i++) {
content.getChildren().add(new Label("Item " + i));
}
// Add scrolling capability to the content
ScrollPane scrollPane = new ScrollPane(content);
// Customize ScrollPane behavior
// Disable horizontal scrollbar
scrollPane.setHbarPolicy(ScrollPane.ScrollBarPolicy.NEVER);
// Always show vertical scrollbar
scrollPane.setVbarPolicy(ScrollPane.ScrollBarPolicy.ALWAYS);
// Set initial vertical scroll position to the middle
scrollPane.setVvalue(0.5);
// Add the scroll pane to the BorderPane layout manager
this.parent.setCenter(scrollPane);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ScrollPane");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we customize the ScrollPane by disabling the horizontal scrollbar, always showing the vertical scrollbar, and setting the initial vertical scroll position to the middle.
Working with ScrollBars
ScrollPane also provides access to its horizontal and vertical ScrollBars, allowing you to control them programmatically. You can add event listeners to ScrollBars and respond to changes in their values. To ensure you can reliably access the scroll bars of a ScrollPane, it’s essential to handle the layout and rendering process properly. Since JavaFX’s layout and rendering might not have completed when attempting to access the scroll bars, a dependable approach is to add a listener to the skinProperty of the ScrollPane.
By adding a listener to the skinProperty, you ensure that you get notified when the ScrollPane is added to the scene and laid out, resulting in the skin property being set. When the listener is triggered, you can retrieve the skin and check if it’s not null. Once the skin is available, you can safely access the horizontal and vertical scroll bars from the skin and work with them as needed.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.control.skin.ScrollPaneSkin;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
VBox content = new VBox(10);
for (int i = 1; i <= 50; i++) {
content.getChildren().add(new Label("Item " + i));
}
// Add scrolling capability to the content
ScrollPane scrollPane = new ScrollPane(content);
// Add listener to the skin property of the ScrollPane
scrollPane.skinProperty().addListener((skinObservable, oldSkin, newSkin) -> {
if(newSkin != null) {
ScrollBar hScrollBar = ((ScrollPaneSkin) newSkin).getHorizontalScrollBar();
ScrollBar vScrollBar = ((ScrollPaneSkin) newSkin).getVerticalScrollBar();
// Add event listeners to ScrollBars
hScrollBar.valueProperty().addListener((observable, oldValue, newValue) -> {
System.out.println("Horizontal Scroll Value: " + newValue);
});
vScrollBar.valueProperty().addListener((observable, oldValue, newValue) -> {
System.out.println("Vertical Scroll Value: " + newValue);
});
}
});
// Add the scroll pane to the BorderPane layout manager
this.parent.setCenter(scrollPane);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ScrollPane");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we access the horizontal and vertical ScrollBars of the ScrollPane and add event listeners to them to print their current values when they change.
ScrollPane with Different Content
ScrollPane is not limited to containing only text or labels; you can use it to display various types of content, such as images, tables, or custom nodes.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Load an image
Image image = new Image("https://via.placeholder.com/640");
// Use an ImageView to display the image
ImageView imageView = new ImageView(image);
ScrollPane scrollPane = new ScrollPane(imageView);
// Add the scroll pane to the BorderPane layout manager
this.parent.setCenter(scrollPane);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ScrollPane");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we load an image from a URL and display it inside a ScrollPane using an ImageView.
Handling Scroll Events
You can also handle scroll events on the ScrollPane to perform custom actions when the user scrolls.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.input.ScrollEvent;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
VBox content = new VBox();
for (int i = 1; i <= 100; i++) {
content.getChildren().add(new Label("Item " + i));
}
ScrollPane scrollPane = new ScrollPane(content);
// Handle scroll events on the ScrollPane
scrollPane.addEventFilter(ScrollEvent.SCROLL, event -> {
double deltaY = event.getDeltaY();
if (deltaY > 0) {
System.out.println("Scrolling Up");
} else {
System.out.println("Scrolling Down");
}
});
// Add the scroll pane to the BorderPane layout manager
this.parent.setCenter(scrollPane);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ScrollPane");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we add a scroll event handler that prints the text “Scrolling Up” to the console when the user scrolls up and “Scrolling Down” when scrolling down.
ScrollPane with Zooming Functionality
In some cases, you might want to provide the ability to zoom in and out of the content within the ScrollPane. Here’s an example of how you can achieve this:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.input.ScrollEvent;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private double scaleValue = 1.0;
private static final double ZOOM_FACTOR = 1.5;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Load an image
Image image = new Image("https://via.placeholder.com/640");
// Use an ImageView to display the image
ImageView imageView = new ImageView(image);
ScrollPane scrollPane = new ScrollPane(imageView);
// Allow panning
scrollPane.setPannable(true);
// Handle scroll events on the ScrollPane
scrollPane.addEventFilter(ScrollEvent.SCROLL, event -> {
if (event.isControlDown()) {
double deltaY = event.getDeltaY();
if (deltaY < 0) {
scaleValue /= ZOOM_FACTOR;
} else {
scaleValue *= ZOOM_FACTOR;
}
// Limit the zoom level
scaleValue = Math.min(2.0, Math.max(0.1, scaleValue));
// Apply the scale transformation to the content
scrollPane.getContent().setScaleX(scaleValue);
scrollPane.getContent().setScaleY(scaleValue);
event.consume();
}
});
// Add the scroll pane to the BorderPane layout manager
this.parent.setCenter(scrollPane);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ScrollPane");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we load an image into an ImageView, and the ScrollPane is configured to allow panning. When the user scrolls while holding down the “Control” key, the content inside the ScrollPane is zoomed in and out by adjusting the scaleValue. We ensure that the zoom level remains within a reasonable range to avoid extreme scaling. Panning allows users to pan within the ScrollPane by clicking and dragging with the mouse. You can enable or disable this feature using the setPannable method.
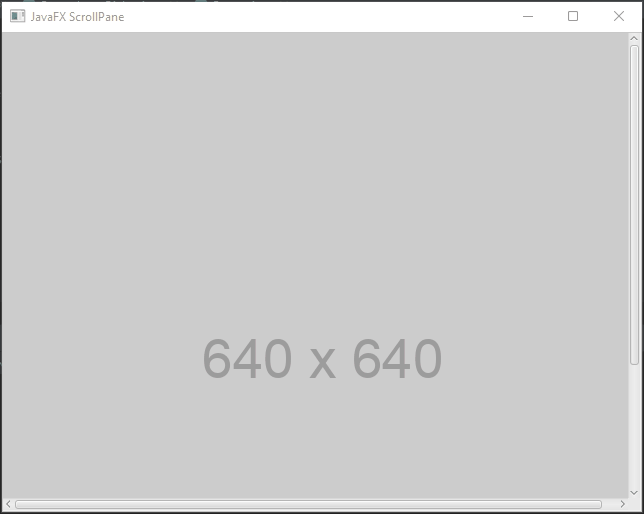
Conclusion
In this article, we explored the ScrollPane in JavaFX, learning how to create a basic ScrollPane, customize its behavior, and handle scroll events. The ScrollPane is an essential component for building applications that involve large amounts of content or data. By using the code examples provided, you can easily integrate the ScrollPane into your JavaFX applications and enhance the user experience.
Remember that this is just the beginning, and the ScrollPane offers even more options and functionalities for you to explore. As you continue to develop JavaFX applications, make sure to check the official JavaFX documentation for more details and examples.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!