One essential aspect of GUI design is the application icon, which represents the identity and branding of your software. JavaFX provides a straightforward way to customize the icon of a stage, enabling developers to add a personal touch and create a lasting impression on users. In this article, we will explore how to set up custom stage icons in JavaFX, along with some code examples to demonstrate the process.
Understanding the Stage Icon
Before diving into the code, let’s understand what a stage icon is. In a JavaFX application, the stage icon is the image that appears in the title bar of the application window and also in the taskbar or dock when the application is minimized. By default, JavaFX uses the default Java coffee cup icon as the stage icon. However, you can easily change this to a custom image that suits your application’s brand or purpose.
Adding Custom Stage Icons
To set a custom stage icon in JavaFX, you’ll need an image file of the icon you want to use. JavaFX supports various image formats, including PNG, JPEG, GIF, and BMP. Use the Image class to load the image from a file or URL. Here’s an example of how to load the image from the local file system:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Load the custom icon image from the local file system
Image iconImage = new Image("icon.png");
// Set the stage icon
stage.getIcons().add(iconImage);
// Set the stage title
stage.setTitle("Customizing JavaFX Stage Icons for Your Applications");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Once you have loaded the image using the Image class, use the getIcons() method of the Stage class to obtain a list of icons associated with the stage. Then, add your custom icon to this list using the add() method.
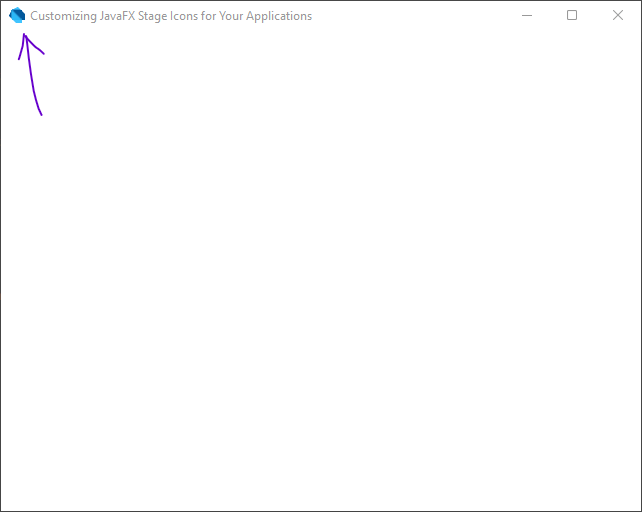
Using Multiple Icons (Optional)
JavaFX allows you to add multiple icons to support different sizes and resolutions. When you add multiple icons, the platform will select the most appropriate icon for the target environment. It’s a good practice to include multiple sizes of your custom icon to ensure proper rendering on different systems.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Load multiple icon images of different sizes
Image icon16x16 = new Image("icon_16x16.png");
Image icon32x32 = new Image("icon_32x32.png");
Image icon64x64 = new Image("icon_64x64.png");
// Set multiple icons for the stage
stage.getIcons().addAll(icon16x16, icon32x32, icon64x64);
// Set the stage title
stage.setTitle("Customizing JavaFX Stage Icons for Your Applications");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Conclusion
Customizing the stage icon of your JavaFX application is a simple yet impactful way to create a unique identity for your software. By following the steps and code examples provided in this article, you can easily set up your custom icon and make your application visually stand out. Remember to include multiple icon sizes for better compatibility across different platforms, and let your creativity shine through your stage icon!
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!