In the world of modern software development, creating user interfaces that respond seamlessly to data changes is paramount. JavaFX, with its robust toolkit for building graphical user interfaces, offers a powerful feature known as data binding. Data binding simplifies the synchronization of data between user interface components and the underlying data model, resulting in more efficient, maintainable, and responsive applications.
User interfaces (UIs) in Java applications are inherently dynamic, often displaying data that changes over time. Manually updating the UI to reflect changes in the data model can be tedious, error-prone, and lead to complex code. JavaFX’s data binding feature addresses these challenges by providing a declarative way to establish a connection between UI components and data objects. In this article, we will explore the fundamentals of data binding in JavaFX and demonstrate how it can be a game-changer for JavaFX developers.
Understanding Data Binding
At its core, data binding is about creating a link between UI components and data values, ensuring that changes in one are automatically reflected in the other. This simplifies UI development by reducing the manual effort required to synchronize data between the view and the model.
In JavaFX, data binding relies on the concepts of properties and observable values. A property is a value that can change over time, while an observable value is a property that can notify its observers when its value changes. JavaFX provides a range of property types, such as IntegerProperty, StringProperty, and BooleanProperty, to accommodate different data types.
Creating Binding Expressions
In JavaFX, you have a variety of mechanisms at your disposal to create binding expressions, which facilitate automatic synchronization of data between properties. These mechanisms include utilizing the Bindings class, employing lambda expressions, or using the bind and unbind methods to establish these bindings.
For example, you can create a binding expression for calculating the area of a rectangle as follows:
import javafx.application.Application;
import javafx.beans.binding.DoubleBinding;
import javafx.beans.property.DoubleProperty;
import javafx.beans.property.SimpleDoubleProperty;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.control.TextFormatter;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import java.util.function.UnaryOperator;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create text fields for width and height
DoublePropertyTextField txtWidth = new DoublePropertyTextField("Width");
DoublePropertyTextField txtHeight = new DoublePropertyTextField("Height");
// Bind the area label to the product of width and height
DoubleBinding area = txtWidth.doubleProperty().multiply(txtHeight.doubleProperty());
Label results = new Label();
// Bind the label to the calculated area
results.textProperty().bind(area.asString());
// Create a container for the UI components
VBox container = new VBox(
15, // Spacing between components
txtWidth, txtHeight,
new VBox(results)
);
container.setAlignment(Pos.CENTER);
container.setMaxWidth(300.0);
// Add the container to the parent StackPane
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Data Binding in JavaFX: Simplifying UI-Data Synchronization");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
// Custom VBox with a DoubleProperty for numeric input
static class DoublePropertyTextField extends VBox {
private final DoubleProperty value = new SimpleDoubleProperty();
public DoublePropertyTextField(String label) {
super(5); // Spacing between label and text field
// Create a text field with a NumericFormatter to allow only numeric input
TextField textField = new TextField();
textField.setTextFormatter(new NumericFormatter());
// Listen for changes in the text field and update the DoubleProperty accordingly
textField.textProperty().addListener((observable, oldValue, newValue) -> {
try {
double newValueAsDouble = Double.parseDouble(newValue);
value.set(newValueAsDouble);
} catch (NumberFormatException nfe) {
value.set(0.0);
}
});
// Add the label and text field to the VBox
getChildren().addAll(new Label(label), textField);
}
public DoubleProperty doubleProperty() {
return value;
}
// Custom TextFormatter to enforce numeric input
static class NumericFormatter extends TextFormatter<Double> {
public NumericFormatter() {
// Initialize the NumericFormatter with appropriate settings
this(change -> {
String newText = change.getControlNewText();
if (newText.matches("[0-9]*\\.?[0-9]*")) {
// Accept the change if it's a valid numeric input
return change;
}
// Reject the change if it's not a valid numeric input
return null;
});
}
private NumericFormatter(UnaryOperator<Change> unaryOperator) {
super(unaryOperator);
}
}
}
}
In this example, we have created a JavaFX application that seamlessly updates the displayed area whenever changes are made to the width and height input fields. This synchronization is achieved through the use of data binding, allowing for real-time calculations and immediate updates in the user interface.
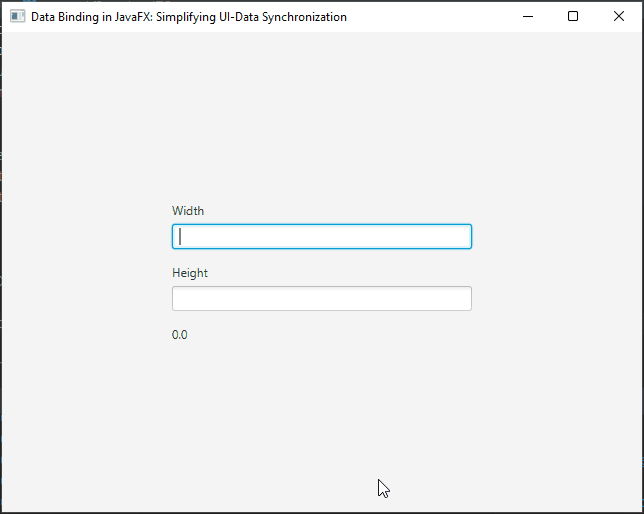
Automatic Updates for Effortless UI Synchronization
One of the fundamental benefits of data binding in JavaFX is its automatic update mechanism. For example, when you bind a label’s text property to a TextField’s textProperty(), you establish a dynamic connection between the two properties. As a result, the label seamlessly and instantly updates its content whenever the value of the TextField’s textProperty() changes. This automatic synchronization simplifies UI-Data interactions, ensuring that the displayed information always reflects the most current user input, without the need for manual updates or event handling.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a TextField for user input
TextField textField = new TextField();
// Create a Label to display the content of the TextField
Label label = new Label();
// Bind the Label's text property to the TextField's text property
label.textProperty().bind(textField.textProperty());
// Create a container to arrange the UI components
VBox container = new VBox(
// Arrange the TextField and Label vertically with spacing
new VBox(15, textField, label)
);
// Center align the container within its parent
container.setAlignment(Pos.CENTER);
// Set a maximum width for the container
container.setMaxWidth(300.0);
// Add the container to the parent StackPane
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Data Binding in JavaFX: Simplifying UI-Data Synchronization");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
This code demonstrates the power of data binding in JavaFX, enabling automatic synchronization between the TextField and Label without the need for explicit event handling. The TextField’s textProperty and the Label’s textProperty are bound together, resulting in real-time updates in the displayed text as the user interacts with the TextField.
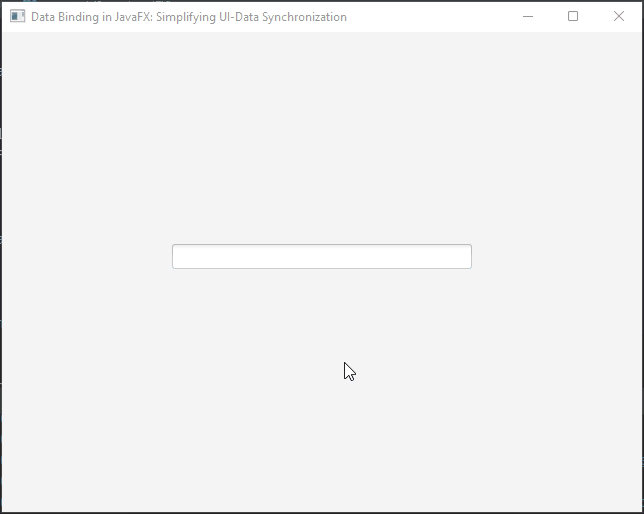
Bidirectional Binding for Two-Way Data Synchronization
JavaFX provides a powerful feature known as bidirectional binding, which facilitates effortless two-way data synchronization between source and target properties. This functionality automatically ensures that changes made to either the source or target property are instantly reflected in the other, creating a seamless and real-time exchange of data.
Bidirectional binding is especially valuable in scenarios where maintaining consistency between two properties is crucial. For instance, consider a situation where you need to keep two input fields synchronized, allowing users to input data in one field and see the same data instantly mirrored in another. Bidirectional binding simplifies such tasks, enhancing the user experience and streamlining data management.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a TextField for user input
TextField textField1 = new TextField();
// Create another TextField for user input
TextField textField2 = new TextField();
// Bind both text properties bidirectionally, making them mirror each other
textField1.textProperty().bindBidirectional(textField2.textProperty());
// Create a container to arrange the UI components
VBox container = new VBox(
// Arrange the TextFields vertically with spacing
new VBox(15, textField1, textField2)
);
// Center align the container within its parent
container.setAlignment(Pos.CENTER);
// Set a maximum width for the container
container.setMaxWidth(300.0);
// Add the container to the parent StackPane
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Data Binding in JavaFX: Simplifying UI-Data Synchronization");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this case, bidirectional binding establishes a seamless two-way interaction between the textField1 and textField2. Any changes made to textField1 will instantly update textField2, and conversely, modifications to textField2 will be promptly reflected in textField1. This bidirectional synchronization simplifies data interaction, ensuring that both text fields consistently mirror each other’s content. As a result, users can effortlessly work with the two text fields, experiencing real-time synchronization.
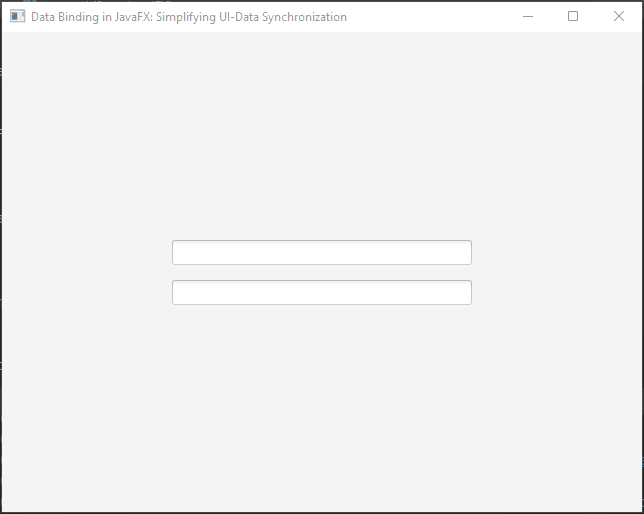
Custom Binding Expressions
Binding expressions in JavaFX are a way to establish relationships between properties, ensuring that when one property changes, others are updated accordingly. While JavaFX provides some built-in bindings for common scenarios, custom binding expressions give you the flexibility to define your own complex relationships.
In our Celsius to Fahrenheit converter application, we’ll create a custom binding expression that automatically calculates the Fahrenheit temperature whenever the user inputs a value in Celsius. This allows for an intuitive and responsive user interface without the need for manual calculations or button presses.
import javafx.application.Application;
import javafx.beans.binding.Bindings;
import javafx.beans.binding.DoubleBinding;
import javafx.beans.property.DoubleProperty;
import javafx.beans.property.SimpleDoubleProperty;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.control.TextFormatter;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import java.util.function.UnaryOperator;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a text field for Celsius input
DoublePropertyTextField txtCelsius = new DoublePropertyTextField("Celsius");
// Create a text field for Fahrenheit input
DoublePropertyTextField txtFahrenheit = new DoublePropertyTextField("Fahrenheit");
// Bindings
DoubleBinding celsiusFahrenheitBinding = Bindings.createDoubleBinding(
() -> (txtCelsius.doubleProperty().get() * 9 / 5) + 32,
txtCelsius.doubleProperty()
);
DoubleBinding fahrenheitCelsiusBinding = Bindings.createDoubleBinding(
() -> (txtFahrenheit.doubleProperty().get() - 32) * 5 / 9,
txtFahrenheit.doubleProperty()
);
// Labels to display converted temperature
Label lblCelsiusToFahrenheit = new Label();
Label lblFahrenheitToCelsius = new Label();
// Bind the label to display the Celsius to Fahrenheit conversion
lblCelsiusToFahrenheit.textProperty().bind(Bindings.format("%.2f °F", celsiusFahrenheitBinding));
// Bind the label to display the Fahrenheit to Celsius conversion
lblFahrenheitToCelsius.textProperty().bind(Bindings.format("%.2f °C", fahrenheitCelsiusBinding));
// Create a container for the UI components
VBox container = new VBox(
15, // Spacing between components
txtCelsius,
txtFahrenheit,
new VBox(10, lblCelsiusToFahrenheit, lblFahrenheitToCelsius)
);
container.setAlignment(Pos.CENTER);
container.setMaxWidth(300.0);
// Add the container to the parent StackPane
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Data Binding in JavaFX: Simplifying UI-Data Synchronization");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
// Custom VBox with a DoubleProperty for numeric input
static class DoublePropertyTextField extends VBox {
private final DoubleProperty value = new SimpleDoubleProperty();
public DoublePropertyTextField(String label) {
super(5); // Spacing between label and text field
// Create a text field with a NumericFormatter to allow only numeric input
TextField textField = new TextField();
textField.setTextFormatter(new NumericFormatter());
// Listen for changes in the text field and update the DoubleProperty accordingly
textField.textProperty().addListener((observable, oldValue, newValue) -> {
try {
double newValueAsDouble = Double.parseDouble(newValue);
value.set(newValueAsDouble);
} catch (NumberFormatException nfe) {
value.set(0.0);
}
});
// Add the label and text field to the VBox
getChildren().addAll(new Label(label), textField);
}
public DoubleProperty doubleProperty() {
return value;
}
// Custom TextFormatter to enforce numeric input
static class NumericFormatter extends TextFormatter<Double> {
public NumericFormatter() {
// Initialize the NumericFormatter with appropriate settings
this(change -> {
String newText = change.getControlNewText();
if (newText.matches("[0-9]*\\.?[0-9]*")) {
// Accept the change if it's a valid numeric input
return change;
}
// Reject the change if it's not a valid numeric input
return null;
});
}
private NumericFormatter(UnaryOperator<Change> unaryOperator) {
super(unaryOperator);
}
}
}
}
This elegant binding expression eliminates the need for manual event handling and updates. As the user types a temperature in Celsius, the converted Fahrenheit temperature is updated instantly, and vice versa.
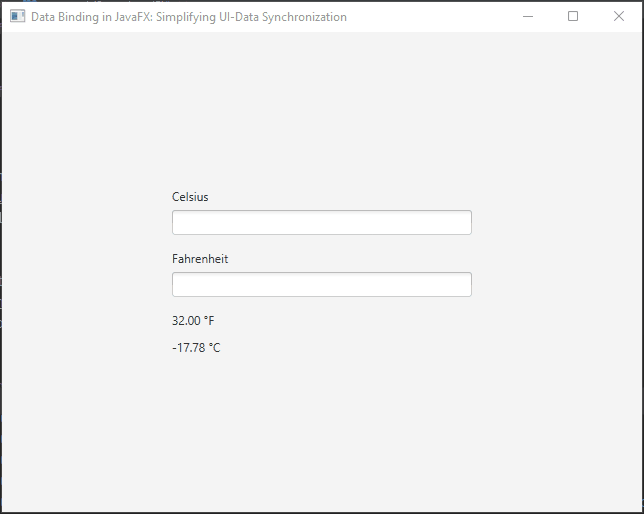
Collections and Listeners for Dynamic UI Elements
Data binding is not limited to scalar values; it extends to collections as well. You can bind UI controls like ListView or TableView to observable lists, and changes to the list will automatically update the UI. Moreover, you can listen for changes using listeners and observers, providing a dynamic and responsive user experience.
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create an observable list of names
ObservableList<String> names = FXCollections.observableArrayList("Name 1");
// Create a ListView bound to the names list
ListView<String> listView = new ListView<>();
listView.setItems(names);
// Create a text field for entering new names
TextField textField = new TextField();
// Create a button to add a new name to the list
Button submit = new Button("Add Name");
// Define an action to execute when the button is clicked
submit.setOnAction(actionEvent -> {
// Add the text from the text field to the names list
names.add(textField.getText());
// Clear the text field for the next entry
textField.clear();
});
// Create a container for the UI components
VBox container = new VBox(
15, // Spacing between components
listView, // Display the list of names
new HBox(10, textField, submit) // Input field and the "Add Name" button
);
container.setAlignment(Pos.CENTER);
container.setMaxWidth(300.0);
// Add the container to the parent StackPane
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Data Binding in JavaFX: Simplifying UI-Data Synchronization");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we create an ObservableList named names, which is bound to a ListView. When we add a new name to the names list, the ListView automatically updates to display the new item. This dynamic binding is particularly useful when dealing with dynamic data in UI components.
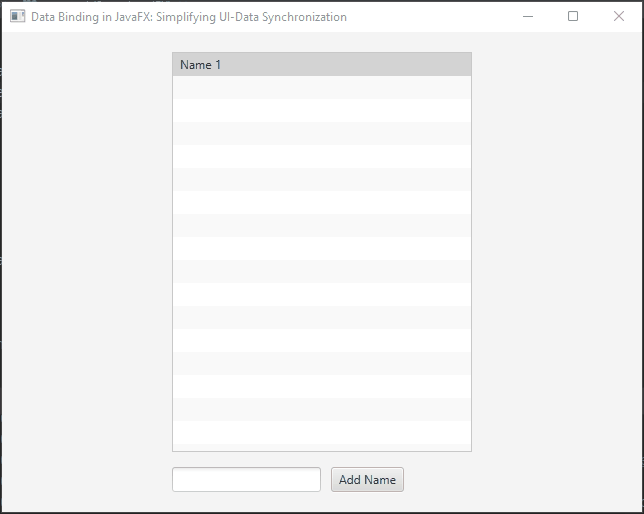
Unbinding for Manual Control
To break a binding relationship, you can use the unbind() method. This is useful when you want to manually control updates or when you no longer need the binding. Here’s how to unbind a property:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a text field for user input
TextField textField = new TextField();
// Create a label to display the text from the text field
Label label = new Label();
// Bind the label's text to the text property of the text field
label.textProperty().bind(textField.textProperty());
// Create a button to bind the label to the text field
Button bind = new Button("Bind");
bind.setOnAction(actionEvent -> label.textProperty().bind(textField.textProperty()));
// Create a button to unbind the label from the text field
Button unbind = new Button("Unbind");
unbind.setOnAction(actionEvent -> label.textProperty().unbind());
// Create a container for the UI components
VBox container = new VBox(
15, // Spacing between components
new VBox(10, textField, label), // Text field and label
new HBox(10, bind, unbind) // Bind and unbind buttons
);
container.setAlignment(Pos.CENTER);
container.setMaxWidth(300.0);
// Add the container to the parent StackPane
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Data Binding in JavaFX: Simplifying UI-Data Synchronization");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we start by binding the text of the label to the text property of the textField, which means any changes in the text field will automatically update the label. However, we also provide the option to “Unbind” by clicking the “Unbind” button. When this button is clicked, the label and text field are unbound, so changes in the text field no longer affect the label’s text.
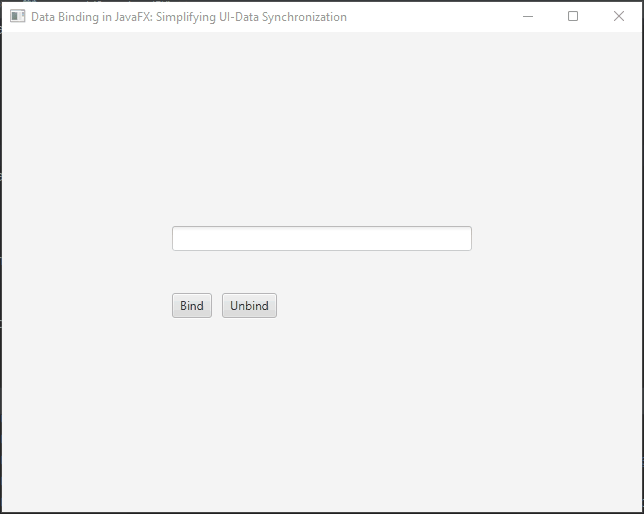
Conclusion
Data binding in JavaFX is a game-changer for developers seeking to create responsive and maintainable user interfaces. By establishing connections between UI components and data objects, JavaFX simplifies the synchronization process, resulting in more efficient and error-resistant code. Whether you’re building a small desktop application or a complex enterprise system, mastering data binding is essential for delivering polished, dynamic user interfaces in JavaFX.
In conclusion, data binding empowers JavaFX developers to build user interfaces that effortlessly reflect changes in the data model, leading to more responsive and maintainable applications. Incorporating data binding into your JavaFX projects can streamline your development process and enhance the user experience, making it a valuable skill for any JavaFX developer.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.