In the world of modern software development, data is king. Whether you’re managing business records, analyzing financial data, or keeping track of your personal expenses, Excel files have become a staple for storing and organizing information. What if you could take the power of Excel and seamlessly integrate it into your JavaFX application? Well, the good news is that you can! In this article, we’ll delve into the exciting world of displaying Excel files in JavaFX.
Why Display Excel Files in JavaFX Tables?
Before diving into the technical details, let’s understand why you might want to display Excel data in JavaFX tables. Excel is a ubiquitous tool for data storage and analysis. When you want to present this data in a more user-friendly and interactive way, JavaFX tables come to the rescue. By displaying Excel data in a table, you can provide users with an organized, searchable, and visually appealing interface for data exploration.
What is Apache POI?
Apache POI (Poor Obfuscation Implementation) is an open-source Java library that provides a comprehensive set of classes and methods for working with Microsoft Office documents, including Word, PowerPoint, and Excel. In this article, we will focus on using Apache POI to handle Excel files, making it easier to display Excel data within a JavaFX application.
Installation
Before we dive into integrating Excel files with JavaFX, let’s start by installing Apache POI. You can download the library directly from the official Apache POI website, which can be found at Apache POI – the Java API for Microsoft Documents.
To streamline the installation process, you can also use Maven, a popular build automation tool, by adding the following dependencies to your project’s pom.xml:
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.2.4</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.4</version>
</dependency>
These dependencies will ensure you have the necessary Apache POI libraries available in your JavaFX project. With Apache POI successfully integrated, let’s explore how to display Excel files in your JavaFX application.
Creating the UserExcelFileReader Class
With the Apache POI library integrated into your project, you can proceed to create a UserExcelFileReader class. This class will be the core component responsible for reading and processing Excel files. You will utilize various classes and methods provided by Apache POI to parse the Excel files and extract the data.
The UserExcelFileReader class acts as the bridge between your JavaFX application and the Excel data. It will handle tasks such as opening Excel files, reading their content, and structuring the data for display. This class is where you can apply Excel-specific operations and ensure that the data becomes readily available for presentation in your JavaFX interface.
import java.io.FileInputStream;
import java.io.IOException;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
public class UserExcelFileReader {
private Workbook workbook;
public UserExcelFileReader(String excelFilePath) throws IOException {
// Open the Excel file
try (FileInputStream file = new FileInputStream(excelFilePath)) {
// Create a Workbook object based on the file extension
if (excelFilePath.endsWith(".xlsx")) {
workbook = new XSSFWorkbook(file);
} else if (excelFilePath.endsWith(".xls")) {
workbook = new HSSFWorkbook(file);
}
}
}
public ObservableList<User> getUsers() {
// Get users from the first sheet
return this.getData(0);
}
public ObservableList<User> getUsers(int sheetIndex) {
// Get users from the specified sheet
return this.getData(sheetIndex);
}
private ObservableList<User> getData(int sheetIndex) {
ObservableList<User> users = FXCollections.observableArrayList();
// Get the specific sheet
Sheet sheet = this.workbook.getSheetAt(sheetIndex);
// Use a flag to skip the first row (header)
boolean isFirstRow = true;
for (Row row : sheet) {
if (!isEmptyRow(row)) {
// Skip the first row
if (isFirstRow) {
isFirstRow = false;
continue;
}
// Extract and create a User object from the row's data
User user = new User(
((int) row.getCell(0).getNumericCellValue()), // ID
row.getCell(1).getStringCellValue(), // First Name
row.getCell(2).getStringCellValue(), // Last Name
row.getCell(3).getStringCellValue(), // Gender
row.getCell(4).getStringCellValue(), // Country
((int) row.getCell(5).getNumericCellValue()), // Age
row.getCell(6).getStringCellValue() // Date
);
users.add(user);
}
}
return users;
}
private boolean isEmptyRow(Row row) {
boolean emptyRow = true;
for (Cell cell : row) {
// Check if the cell is empty or null
if (cell != null && cell.getCellType() != CellType.BLANK) {
emptyRow = false;
break; // No need to continue checking if any cell is not empty
}
}
return emptyRow;
}
public int getNumberOfSheets() {
// Return the number of sheets in the workbook
return workbook.getNumberOfSheets();
}
// Define the User record with attributes
public record User(int id, String firstName, String lastName, String gender, String country, int age, String date) {
public int getId() {
return id;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getGender() {
return gender;
}
public String getCountry() {
return country;
}
public int getAge() {
return age;
}
public String getDate() {
return date;
}
}
}
The UserExcelFileReader class encapsulates the functionality to open and read Excel files. It provides methods to retrieve user data from the Excel sheets, skip header rows, and determine whether a row is empty. This class also offers a getNumberOfSheets method to return the number of sheets in the workbook.
To structure the data, we’ve created a User record that defines the attributes of each user. This record simplifies the process of representing and working with user data extracted from the Excel sheets.
With the UserExcelFileReader class in place, you can now effectively read and manage Excel data within your JavaFX application. This enables you to create dynamic and data-driven user interfaces with ease.
Displaying Data in JavaFX
Having successfully implemented the UserExcelFileReader class, the final step involves seamlessly presenting the extracted Excel data within your JavaFX application. JavaFX offers a wide array of user interface components, such as TableView, ListView, and the flexibility to create custom controls. These components are pivotal in crafting a user-friendly, interactive interface for your application. You can efficiently bind the data obtained from the UserExcelFileReader class to these JavaFX components, ensuring that your Excel data is exhibited in a visually pleasing and organized manner. By doing so, your users can effortlessly navigate and interact with the Excel data within your JavaFX application, enhancing their overall experience.
Using TableView to Display Excel Data
One of the most effective ways to display Excel data in a structured and organized manner is by using a TableView. Here’s how you can integrate the UserExcelFileReader class with a TableView to display the data:
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.scene.Scene;
import java.io.IOException;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() throws IOException {
// Create a UserExcelFileReader to read data from the Excel file
UserExcelFileReader reader = new UserExcelFileReader("sample.xlsx");
ObservableList<UserExcelFileReader.User> users = reader.getUsers();
// Create a UserTableView to display the data
UserTableView userTableView = new UserTableView(users);
// Add the TableView to the parent layout
this.parent.getChildren().add(userTableView);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Displaying Excel Data in JavaFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
class UserTableView extends TableView<UserExcelFileReader.User> {
public UserTableView(ObservableList<UserExcelFileReader.User> users) {
super(users);
// Create TableColumn instances for each attribute
TableColumn<UserExcelFileReader.User, Integer> idColumn = this.createColumn("ID", "id");
TableColumn<UserExcelFileReader.User, String> firstNameColumn = this.createColumn("First Name", "firstName");
TableColumn<UserExcelFileReader.User, String> lastNameColumn = this.createColumn("Last Name", "lastName");
TableColumn<UserExcelFileReader.User, String> genderColumn = this.createColumn("Gender", "gender");
TableColumn<UserExcelFileReader.User, String> countryColumn = this.createColumn("Country", "country");
TableColumn<UserExcelFileReader.User, Integer> ageColumn = this.createColumn("Age", "age");
TableColumn<UserExcelFileReader.User, String> dateColumn = this.createColumn("Date", "date");
// Add the TableColumn instances to the TableView
this.getColumns().addAll(idColumn, firstNameColumn, lastNameColumn, genderColumn, countryColumn, ageColumn, dateColumn);
}
private <T> TableColumn<UserExcelFileReader.User, T> createColumn(String title, String property) {
TableColumn<UserExcelFileReader.User, T> column = new TableColumn<>(title);
column.setCellValueFactory(new PropertyValueFactory<>(property));
return column;
}
}
In this code, we create an instance of UserExcelFileReader, extract user data, and bind it to a TableView. You can define columns for the TableView to represent different user attributes. These columns are populated with the data from the Excel file. The result is a user-friendly interface where users can view and interact with the Excel data efficiently.
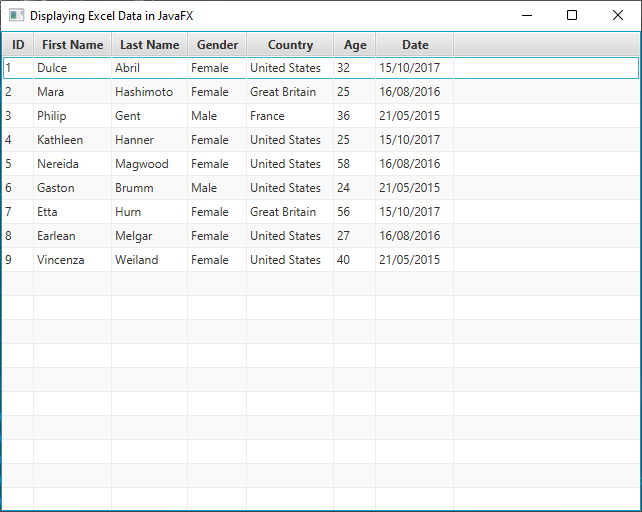
Real-World Examples
Let’s look at a couple of real-world examples of applications that benefit from displaying Excel files in JavaFX.
Financial Management Application
Imagine a financial management application where users can import their financial data from Excel files. They can view their income, expenses, and investment portfolios in interactive JavaFX charts and tables. With real-time updates, users can instantly see the impact of their financial decisions.
Inventory Management System
In an inventory management system, businesses can import their product lists and inventory data from Excel files. The JavaFX application can display real-time stock levels, generate reports, and provide alerts when stock is running low. This integration simplifies inventory management and reduces errors.
Conclusion
Integrating Excel files into your JavaFX application is a powerful feature that can greatly enhance the functionality and usability of your software. Apache POI makes working with Excel files in JavaFX a straightforward process, allowing you to create dynamic and data-driven applications.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.
Oh my god, thanks
You are welcome! 😊