JavaFX provides a powerful canvas for creating rich graphical user interfaces and visual elements in your applications. When it comes to drawing basic shapes, such as rectangles, JavaFX offers several methods to achieve this on a Canvas. In this article, we’ll explore how to draw four different types of rectangles on a JavaFX Canvas: filled rectangles, filled round rectangles, stroked round rectangles, and stroked rectangles. We’ll provide full code examples for each type of rectangle.
Drawing Filled Rectangles
The fillRect method of the GraphicsContext class is used to draw a filled rectangle on the canvas. It takes four parameters: x and y represent the top-left corner of the rectangle, and w and h represent its width and height, respectively. Here’s an example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the fill color
gc.setFill(Color.BLUE);
// Draw a filled rectangle
gc.fillRect(50, 50, 200, 100);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Rectangles in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
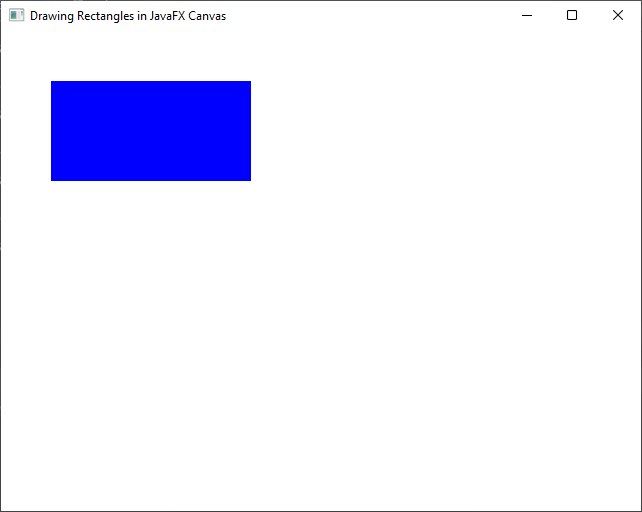
In this example, we created a canvas, obtain its GraphicsContext, set the fill color to blue, and then use fillRect to draw a filled rectangle with a top-left corner at (50, 50) and dimensions of 200×100.
Drawing Filled Round Rectangles
The fillRoundRect method of the GraphicsContext class is similar to fillRect but allows you to draw rounded rectangles with specified arc widths and heights for the corners. Here’s an example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the fill color
gc.setFill(Color.GREEN);
// Draw a filled round rectangle
gc.fillRoundRect(50, 50, 200, 100, 20, 20);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Rectangles in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
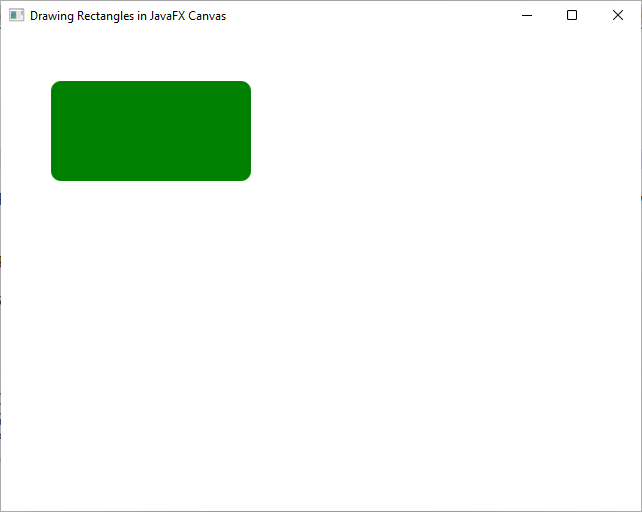
In this example, we use fillRoundRect to draw a filled rounded rectangle with specified arc widths (20) and heights (20) at the corners.
Drawing Stroked Round Rectangles
The strokeRoundRect method is used to draw the outline of a rounded rectangle with specified arc widths and heights for the corners. Here’s an example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set a stroke color, and width
gc.setStroke(Color.PURPLE);
gc.setLineWidth(5.0);
// Draw a stroked round rectangle
gc.strokeRoundRect(50, 50, 200, 100, 20, 20);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Rectangles in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
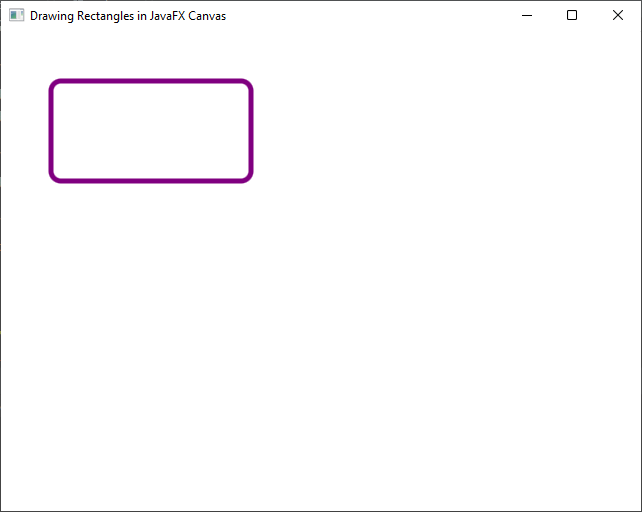
In this example, we use strokeRoundRect to draw the outline of a rounded rectangle with specified arc widths (20) and heights (20) at the corners. We also set the stroke color to purple and the line width to 5.0.
Drawing Stroked Rectangles
The strokeRect method is used to draw the outline of a rectangle without rounded corners. Here’s an example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set a stroke color, and width
gc.setStroke(Color.ORANGE);
gc.setLineWidth(3.0);
// Draw a stroked rectangle
gc.strokeRect(50, 50, 200, 100);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Rectangles in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
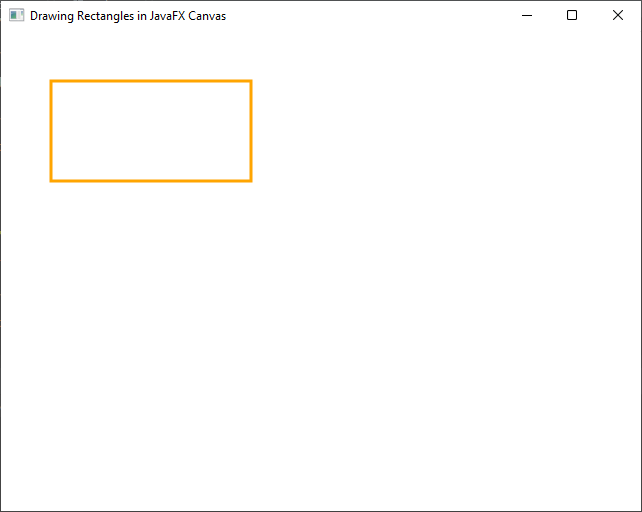
In this example, we use strokeRect to draw the outline of a rectangle without rounded corners. We set the stroke color to orange and the line width to 3.0.
Conclusion
Drawing rectangles in JavaFX Canvas is a fundamental skill for creating graphical user interfaces and custom graphics. In this article, we’ve covered four essential methods for drawing rectangles, each with its unique characteristics. By using these methods and understanding their parameters, you can create a wide range of visual elements in your JavaFX applications.
Remember that these methods can be combined and customized to create complex shapes and visuals. Experiment with different colors, sizes, and coordinates to achieve the desired appearance for your graphics. Don’t forget to consult the JavaFX Canvas Documentation for further information.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!