Buttons are a fundamental component of any graphical user interface, allowing users to interact with your application. In JavaFX, creating and handling buttons is straightforward. The code snippet below demonstrates how to use buttons and handle click events in JavaFX, empowering you to create interactive and responsive applications.
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private Scene scene;
private Label label;
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// create the main content pane
VBox mainContent = new VBox(10);
mainContent.setAlignment(Pos.CENTER);
// create button
Button button = new Button("Button");
this.label = new Label("I dare you to click on the button!");
// setting a button handler
button.setOnAction(this::buttonHandler);
// add label and button to the main content pane
mainContent.getChildren().addAll(this.label, button);
// Create the layout manager using BorderPane
BorderPane layoutManager = new BorderPane(mainContent);
// create the scene with specified dimensions
this.scene = new Scene(layoutManager, 640, 480);
}
// button handler
private void buttonHandler(ActionEvent actionEvent) {
this.label.setText("Oh, you just did. I am offended!");
}
@Override
public void start(Stage stage) throws Exception {
// set the scene for the stage
stage.setScene(this.scene);
stage.setTitle("Getting Started with JavaFX Buttons");
stage.centerOnScreen();
stage.show();
}
}
When executed, the above program creates a simple UI with a button and a label, as depicted in the images below. When the button is clicked, the label’s text is updated:
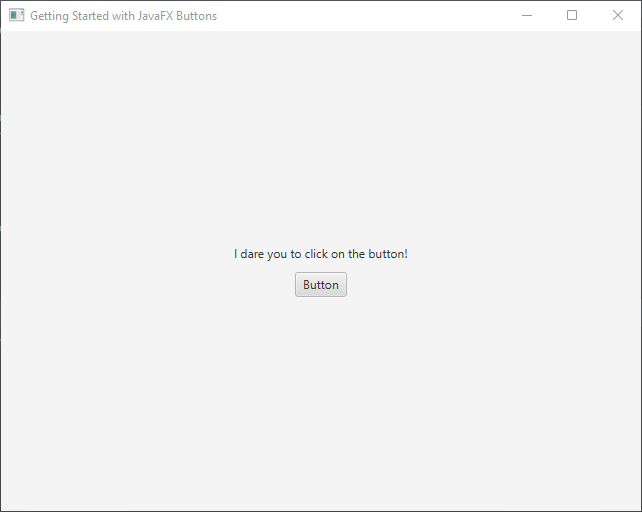
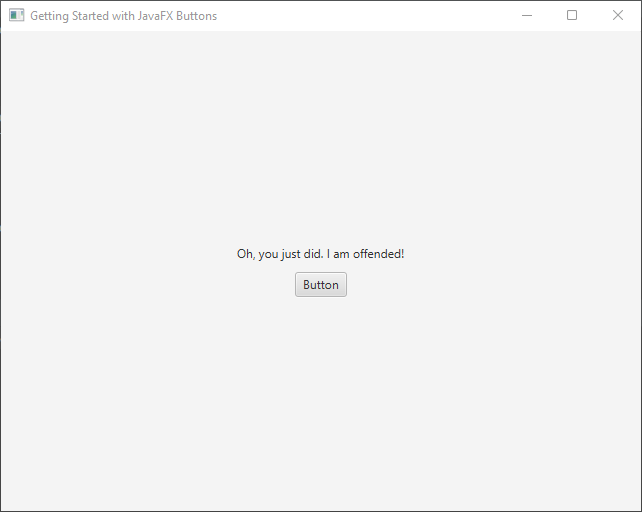
I hope you found this code informative and helpful in enhancing your JavaFX application. If you like this and would like to see more like it, make sure to subscribe to stay updated with my latest code snippets. 😊