Checkboxes are a common UI element used to offer users selectable or deselectable options. One prevalent scenario where checkboxes are used is during software installation when users must agree to the terms of service and license before proceeding. This is just one example. This guide explores the usage of checkboxes in JavaFX.
Basic Usage
The code shown below shows a straightforward example that demonstrates the use of checkboxes in JavaFX:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private Scene scene;
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// create the main content pane
VBox mainContent = new VBox(10);
mainContent.setAlignment(Pos.CENTER);
// create button
Button button = new Button("Install Software");
// disable button
button.setDisable(true);
CheckBox checkBox = new CheckBox("I agree to the license and terms.");
// set check box change listener
checkBox.selectedProperty().addListener((observableValue, prevState, currentState) -> button.setDisable(!currentState));
// create button container, and add check box and button
VBox buttonContainer = new VBox(10, checkBox, button);
buttonContainer.setMaxWidth(200.0);
// add button container to the main content pane
mainContent.getChildren().addAll(buttonContainer);
// Create the layout manager using BorderPane
BorderPane layoutManager = new BorderPane(mainContent);
// create the scene with specified dimensions
this.scene = new Scene(layoutManager, 640.0, 480.0);
}
@Override
public void start(Stage stage) throws Exception {
// set the scene for the stage
stage.setScene(this.scene);
stage.setTitle("Getting Started with JavaFX CheckBoxes");
stage.centerOnScreen();
stage.show();
}
}
When executed, the program above creates a simple user interface with a checkbox and a button, as shown in the images below. When the checkbox is checked, the button’s disabled state changes from true to false, and vice versa.
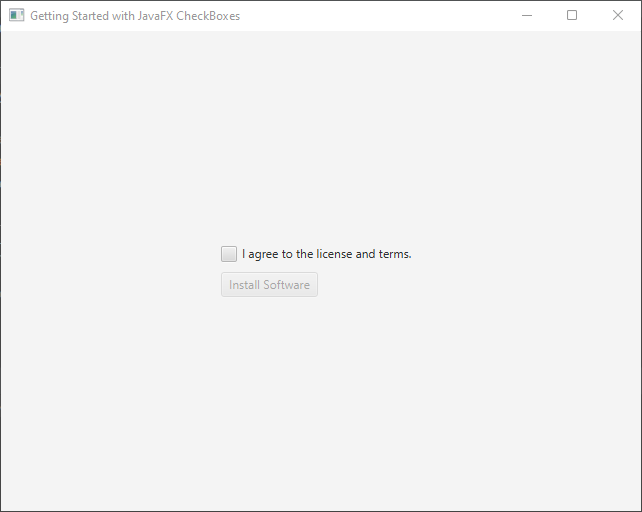
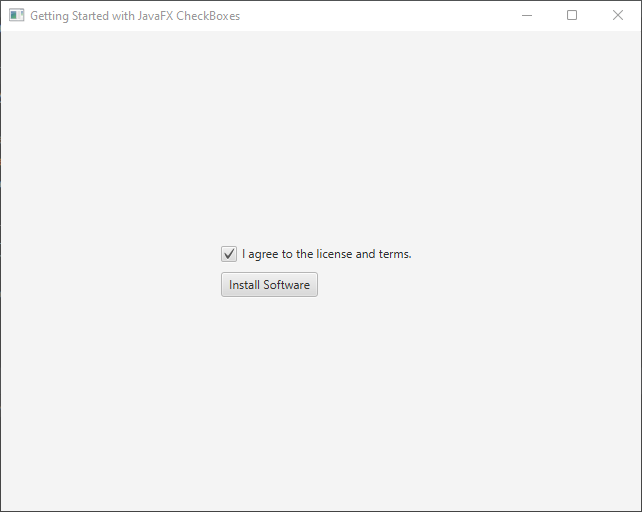
I hope you found this code informative and helpful in enhancing your JavaFX application. If you like this and would like to see more like it, make sure to subscribe to stay updated with my latest code snippets. 😊