When developing Java applications with graphical user interfaces (GUIs), it’s crucial to maintain a clean and maintainable codebase. JavaFX, a popular GUI framework, provides developers with powerful tools for building user-friendly applications. One key principle in software development is the separation of concerns, and JavaFX makes it easier to achieve this by allowing you to separate the user interface (UI) from the application’s logic. In this article, we’ll explore how to do this using JavaFX and FXML.
Separation of Concerns
The separation of concerns is a design principle that encourages dividing a software application into distinct and loosely-coupled components, each responsible for a specific aspect of the application’s functionality. In the context of GUI development, this often means separating the user interface from the application’s underlying logic.
By separating the UI from the logic, you can achieve several benefits:
Modularity: It becomes easier to understand and maintain the code when different parts of the application have well-defined responsibilities.
Reusability: You can reuse UI components or logic in other parts of the application or in entirely different projects.
Collaboration: Multiple developers can work on the UI and logic independently, reducing conflicts and streamlining development.
Introducing FXML
FXML is an XML-based markup language that allows you to define the structure and layout of a JavaFX UI in a declarative manner. Instead of creating UI components programmatically in Java code, you can define them in FXML files and then connect them to your Java code using controllers. This separation of the UI definition (FXML) from the logic (Java code) is a key aspect of the separation of concerns.
Building a To-Do List Application
We’ll create a basic to-do list application with a JavaFX user interface. The UI will include a text field for adding tasks and a list view for displaying them. We’ll follow the principles of separation of concerns by using FXML to define the UI and Java code to handle the logic.
Design the UI with FXML
Let’s define the UI components (TextField, Button, and ListView) in the FXML file (todo.fxml) and specify the controller class (TodoController) using fx:controller.
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.ListView?>
<?import javafx.scene.control.TextField?>
<?import javafx.scene.layout.VBox?>
<VBox xmlns="http://javafx.com/javafx"
xmlns:fx="http://javafx.com/fxml"
spacing="10"
style="-fx-padding: 30px;"
fx:controller="TodoController">
<TextField fx:id="taskInput" promptText="Enter a task" />
<Button text="Add" onAction="#addTask" />
<ListView fx:id="taskList" />
</VBox>
The FXML file is an XML-based markup that defines the user interface for our to-do list application. It specifies the layout of the elements and their properties. In this case, it uses a VBox (vertical box) layout to stack the UI elements vertically. Inside the VBox, we define three UI components: a TextField for entering tasks, a “Add” Button for adding tasks, and a ListView for displaying the list of tasks. Each UI component has attributes and properties specified, such as fx:id to uniquely identify them and onAction to specify the action to be taken when the button is clicked.
Create a Controller Class
import javafx.fxml.FXML;
import javafx.scene.control.ListView;
import javafx.scene.control.TextField;
public class TodoController {
// Reference to the TextField for task input
@FXML
private TextField taskInput;
// Reference to the ListView for displaying tasks
@FXML
private ListView<String> taskList;
@FXML
public void addTask() {
// Retrieve the task text from the input field
// and trim any leading/trailing spaces
String task = taskInput.getText().trim();
// Check if the task is not empty
if (!task.isEmpty()) {
// Add the task to the ListView
taskList.getItems().add(task);
// Clear the input field for the next task
taskInput.clear();
}
}
}
The controller class (TodoController) is responsible for managing the application’s logic. It’s annotated with @FXML, indicating that it’s associated with the FXML file. In this class, we declare two UI component fields (taskInput and taskList) and annotate them with @FXML. These fields are automatically injected with references to the corresponding UI elements defined in the FXML file. The addTask method is also annotated with @FXML and is called when the “Add” button is clicked. In this method, we retrieve the text from the taskInput field, add it to the taskList, and then clear the input field.
Load the FXML File
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Scene;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.io.IOException;
import java.net.URL;
public class Main extends Application {
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) throws IOException {
URL fxml = this.getClass().getResource("todo.fxml");
VBox parent = FXMLLoader.load(fxml);
// Create a scene with the VBox as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX and FXML: Separating UI from Logic");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
The Main class is responsible for setting up the JavaFX stage, loading the FXML file, and displaying the UI. When the application runs, you will see a window with the title “JavaFX and FXML: Separating UI from Logic.” Inside this window, you’ll find the user interface components defined in the todo.fxml file, which includes a text field for adding tasks, a button labeled “Add” to add tasks, and a list view for displaying the tasks. Users can interact with this UI to add and display tasks, and the logic for adding tasks is handled by the TodoController class.
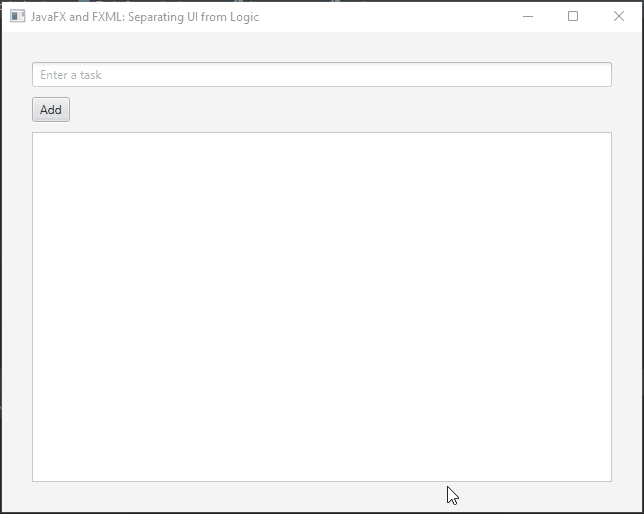
This separation of UI definition in FXML and logic in Java code allows for a clean and modular approach to building JavaFX applications, making them more maintainable and extensible.
Conclusion
JavaFX and FXML provide a powerful way to separate the user interface from the application logic, following the principles of the separation of concerns. By using FXML to define the UI and Java code for the logic, you can create maintainable, modular, and reusable GUI applications.
In this article, we demonstrated how to build a basic to-do list application as an example. However, the principles discussed here apply to more complex JavaFX projects as well. As you continue to work with JavaFX and FXML, you’ll discover how this separation of concerns enhances your productivity and helps you create more robust GUI applications.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!