JavaFX provides a rich set of UI controls that enable developers to create interactive and user-friendly applications. One such control is the ChoiceBox, which allows users to select options from a dropdown list.
Basic Usage
The code snippet provided demonstrates how to create a ChoiceBox, handle selection changes, bind data, and get the selected option:
import javafx.application.Application;
import javafx.beans.value.ObservableValue;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ChoiceBox;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private Scene scene;
private static final ObservableList<String> fruits = FXCollections.observableArrayList(
"Apple", "Banana", "Orange", "Grapefruit", "Lemon",
"Lime", "Mango", "Pineapple", "Watermelon", "Strawberry",
"Blueberry", "Raspberry", "Blackberry", "Cherry", "Peach",
"Plum", "Pear", "Kiwi", "Avocado", "Papaya", "Pomegranate",
"Fig", "Coconut", "Guava", "Passion Fruit", "Lychee", "Dragon Fruit",
"Cranberry", "Apricot", "Cantaloupe", "Honeydew Melon", "Nectarine",
"Grape", "Kiwifruit", "Persimmon", "Tangerine", "Clementine", "Star Fruit",
"Jackfruit", "Elderberry"
);
private ChoiceBox<String> fruitChoiceBox;
private Label buttonLabel;
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// create the main content pane
VBox mainContent = new VBox(10);
mainContent.setAlignment(Pos.CENTER);
// create the fruit choice box
this.fruitChoiceBox = new ChoiceBox<>(fruits);
// create the fruit choice box bound label
Label boundLabel = new Label();
// create the label to display the fruit on button click
this.buttonLabel = new Label();
// create submit button
Button button = new Button("Submit");
button.setOnAction(this::onFruitSubmit);
// select first fruit
fruitChoiceBox.getSelectionModel().selectFirst();
// bind the label to the choice box selected value
boundLabel.textProperty().bind(fruitChoiceBox.valueProperty());
// listen for choice box changes
fruitChoiceBox.getSelectionModel().selectedItemProperty().addListener(this::onFruitChanged);
// add all nodes to the vbox container
VBox container = new VBox(10, fruitChoiceBox, boundLabel, button, buttonLabel);
container.setMaxWidth(200.0);
// add container to the main content pane
mainContent.getChildren().addAll(container);
// create the layout manager using BorderPane
BorderPane layoutManager = new BorderPane(mainContent);
// create the scene with specified dimensions
this.scene = new Scene(layoutManager, 640.0, 480.0);
}
private void onFruitSubmit(ActionEvent actionEvent) {
// displays the selected fruit in a label on button click
this.buttonLabel.setText("The Selected Fruit Is: " + fruitChoiceBox.getValue());
}
private void onFruitChanged(ObservableValue<? extends String> observableValue, String oldSelectedFruit, String newSelectedFruit) {
// prints selected fruit out to the console whenever there is a change
System.out.println(newSelectedFruit);
}
@Override
public void start(Stage stage) throws Exception {
// set the scene for the stage
stage.setScene(this.scene);
// set the title for the stage
stage.setTitle("JavaFX ChoiceBox");
// center the stage on screen on startup
stage.centerOnScreen();
// show the stage
stage.show();
}
}
When you run the program, it generates a user interface (UI) as shown in the image below. The label below the ChoiceBox updates whenever there is a change in the selected fruit because the label is bound to the ChoiceBox. The submit button is responsible for displaying the text in the label below it. This is done to demonstrate how to retrieve the selected value from the ChoiceBox. Additionally, whenever there is a change in the selected fruit, the program prints the selected fruit to the console.
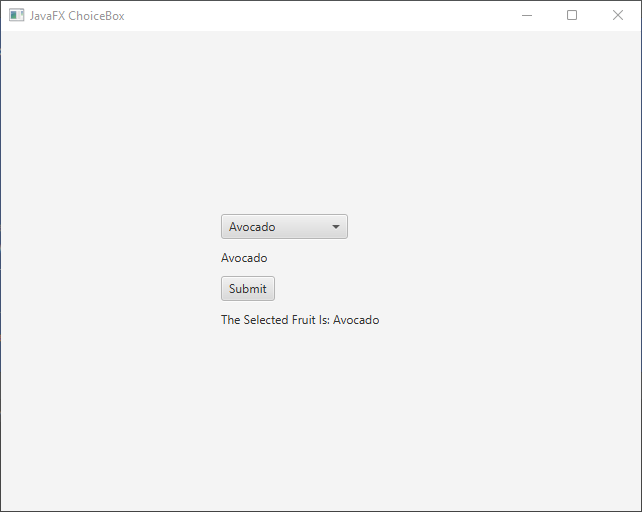
I hope you found this code informative and helpful in enhancing your JavaFX application. If you like this and would like to see more like it, make sure to subscribe to stay updated with my latest code snippets. 😊