In JavaFX, layout panes play a vital role in organizing and positioning graphical elements within the user interface. One such layout pane is the VBox, which stands for Vertical Box. The VBox allows you to arrange components vertically, stacking them one on top of another.
The code creates a simple registration form whose fields are organized in a vertical column using a VBox layout container:
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
@Override
public void start(Stage stage) {
VBox layoutManager = new VBox();
layoutManager.setAlignment(Pos.CENTER);
Scene scene = new Scene(layoutManager, WIDTH, HEIGHT);
/* add nodes to the VBox */
layoutManager.getChildren().add(new FormFields());
stage.setTitle("JavaFX Layouts with VBox");
stage.setScene(scene);
stage.centerOnScreen();
stage.show();
}
private static class FormFields extends VBox {
public FormFields() {
/* 15-pixel spacing between nodes */
super(15);
/* sets the VBox width */
this.setMinWidth(300);
this.setMaxWidth(300);
this.setPrefWidth(300);
/* adds nodes to the VBox layout panel */
getChildren().addAll(
new RegistrationFormField("First Name"),
new RegistrationFormField("Last Name"),
new RegistrationFormField("Email"),
new RegistrationFormField("Password", true),
new RegistrationFormField("Confirm Password", true),
new CheckBox("I agree to the terms & conditions"),
new Button("Register")
);
}
private static class RegistrationFormField extends VBox {
private TextField textField;
public RegistrationFormField(String label)
{
super(2);
init(label, false);
}
public RegistrationFormField(String label, boolean obscure)
{
super(2);
init(label, obscure);
}
private void init(String label, boolean obscure)
{
this.textField = obscure ? new PasswordField() : new TextField();
this.textField.setPadding(new Insets(6));
this.getChildren().addAll(new Label(label), textField);
}
public String getText() {
return this.textField.getText();
}
}
}
}
When executed, the program above produces a window, as depicted in the image below.
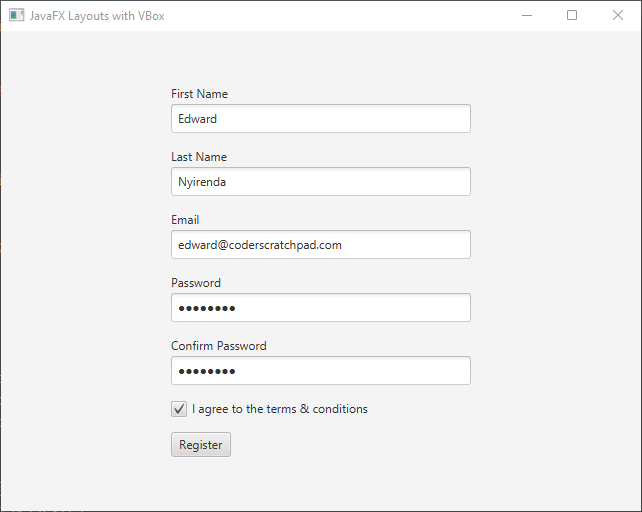
I sincerely hope that you find this code helpful. If you wish to learn more about JavaFX, please subscribe to our newsletter today and continue your JavaFX learning journey with us!