JavaFX is a powerful library for building desktop applications with rich graphical user interfaces. One essential component for managing large sets of data or content is pagination. Pagination divides content into manageable chunks and allows users to navigate through them easily. In this article, we will explore how to implement pagination in JavaFX using the Pagination control. We will cover the fundamental concepts and provide clear code examples to help you get started quickly.
Getting Started
Before we dive into the code examples, make sure you have JavaFX properly set up in your development environment. You can find the necessary libraries and tools at the official JavaFX website. Once everything is set up, let’s proceed with the implementation.
Setting Up the JavaFX Application
First, create a new JavaFX project or class and import the required packages.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX Pagination Made Simple");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
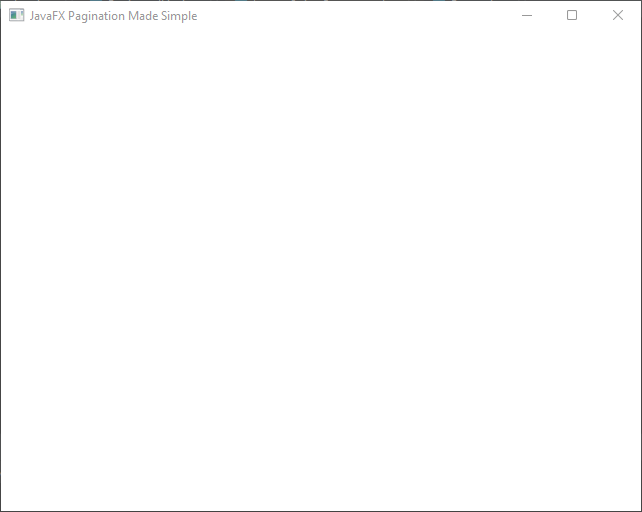
Creating the Pagination Control
In this step, we’ll initialize the Pagination control and set its properties.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Pagination;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private static final int ITEMS_PER_PAGE = 10;
private static final int TOTAL_ITEMS = 100;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Pagination pagination = new Pagination(TOTAL_ITEMS / ITEMS_PER_PAGE);
pagination.setMaxPageIndicatorCount(5);
this.parent.setCenter(pagination);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX Pagination Made Simple");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
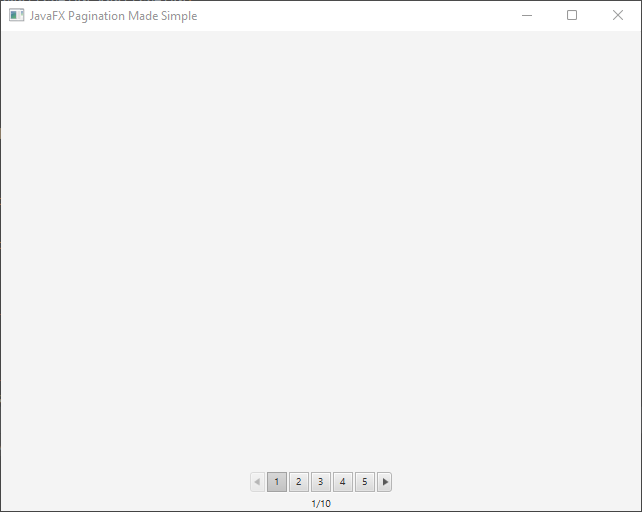
Implementing the Page Factory
Next, we’ll implement the createPage method, which will create and return the content for each page.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Pagination;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private static final int ITEMS_PER_PAGE = 10;
private static final int TOTAL_ITEMS = 100;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Pagination pagination = new Pagination(TOTAL_ITEMS / ITEMS_PER_PAGE);
pagination.setPageFactory(this::createPage);
pagination.setMaxPageIndicatorCount(5);
this.parent.setCenter(pagination);
}
private VBox createPage(int pageIndex) {
int startIndex = pageIndex * ITEMS_PER_PAGE;
int endIndex = Math.min(startIndex + ITEMS_PER_PAGE, TOTAL_ITEMS);
VBox page = new VBox(10);
page.setAlignment(Pos.CENTER);
for (int i = startIndex; i < endIndex; i++) {
Label label = new Label("Item " + (i + 1));
page.getChildren().add(label);
}
return page;
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX Pagination Made Simple");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
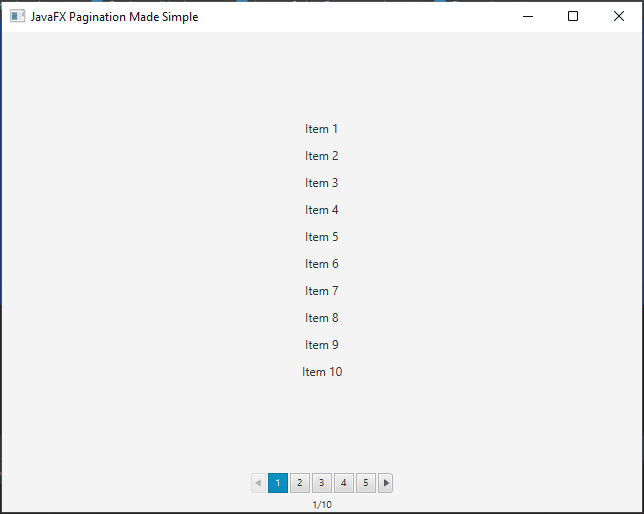
Handling Page Change Events (Optional)
If you wish to perform additional actions when the user changes the page, you can add an event handler for the pagination control.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Pagination;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private static final int ITEMS_PER_PAGE = 10;
private static final int TOTAL_ITEMS = 100;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Pagination pagination = new Pagination(TOTAL_ITEMS / ITEMS_PER_PAGE);
pagination.setPageFactory(this::createPage);
pagination.setMaxPageIndicatorCount(5);
pagination.currentPageIndexProperty().addListener((obs, oldIndex, newIndex) -> {
//Print the current page to the console
System.out.println("Page Changed: " + (newIndex.intValue() + 1));
});
this.parent.setCenter(pagination);
}
private VBox createPage(int pageIndex) {
int startIndex = pageIndex * ITEMS_PER_PAGE;
int endIndex = Math.min(startIndex + ITEMS_PER_PAGE, TOTAL_ITEMS);
VBox page = new VBox(10);
page.setAlignment(Pos.CENTER);
for (int i = startIndex; i < endIndex; i++) {
Label label = new Label("Item " + (i + 1));
page.getChildren().add(label);
}
return page;
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX Pagination Made Simple");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
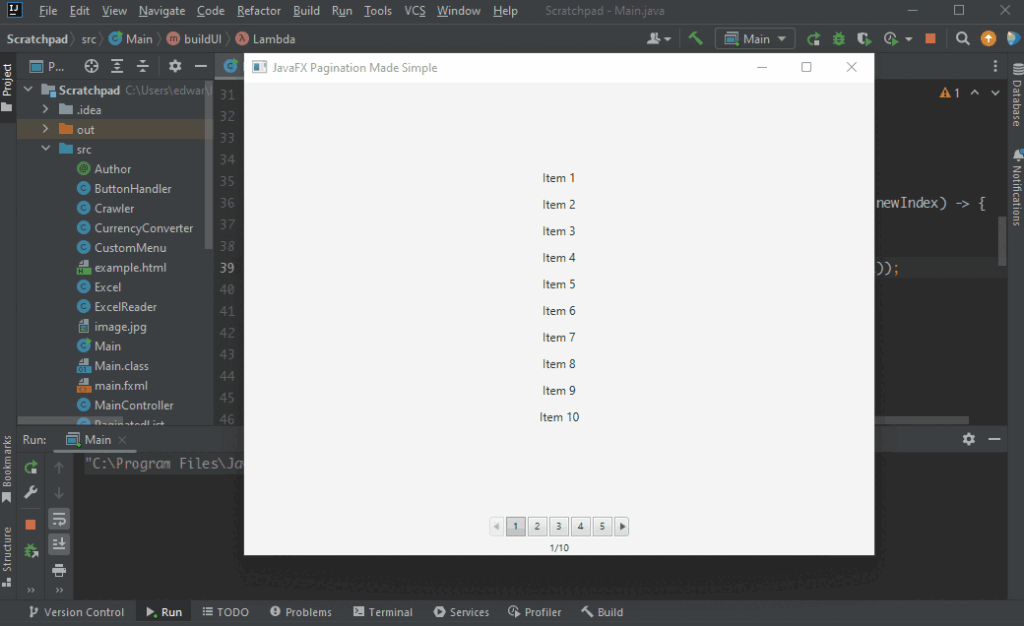
Conclusion
In this article, we’ve explored how to implement pagination in JavaFX using the Pagination control. By dividing large sets of data into smaller, manageable chunks, pagination enhances user experience and makes navigating through content more convenient. With the provided concise code examples, you should now be able to integrate pagination into your JavaFX applications with ease.
Remember that JavaFX offers various customization options for the Pagination control, allowing you to tailor the appearance and behavior to suit your specific application requirements. To delve deeper into JavaFX and explore more components and features, refer to the official documentation and community resources.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!