Text input is a fundamental aspect of modern software applications, and when it comes to building rich graphical user interfaces (GUIs), JavaFX provides a powerful toolset for creating interactive and user-friendly applications. One of the essential components in a GUI application is the TextArea, which allows users to input and edit multiple lines of text. In this article, we will delve into the world of JavaFX TextArea, exploring its features, functionality, and providing comprehensive code examples.
Introduction to TextArea
TextArea is a JavaFX control designed for multi-line text input and display. It allows users to enter and edit text spanning multiple lines, making it ideal for tasks like text editing, note-taking, chat applications, and more.
Creating a Basic TextArea
Let’s start by creating a simple JavaFX application with a TextArea component. Here’s the basic structure of the application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we create a simple JavaFX application that displays a TextArea inside a BorderPane layout container. This provides a basic GUI environment to work with the TextArea.
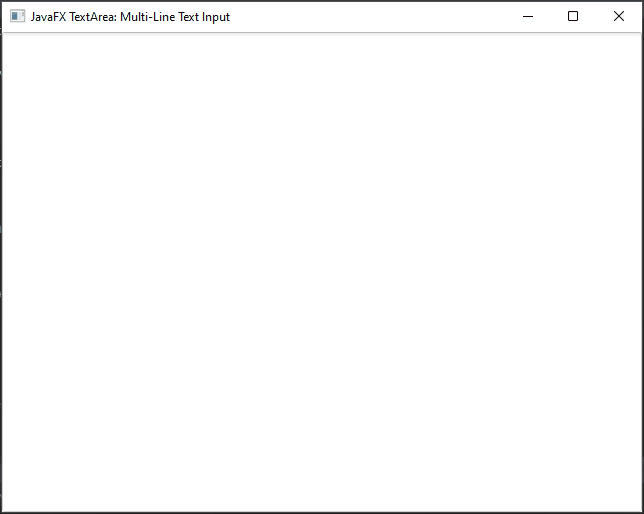
Customizing the TextArea
You can customize the appearance and behavior of a TextArea using its various properties and methods. Some common customizations include:
Setting and Getting Text
You can set and get the text content of the TextArea using its setText and getText methods, respectively:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
// Set initial text
textArea.setText("Hello, JavaFX!");
// Get the text in the TextArea
String content = textArea.getText();
// Print the text in the TextArea to the console
System.out.println(content);
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
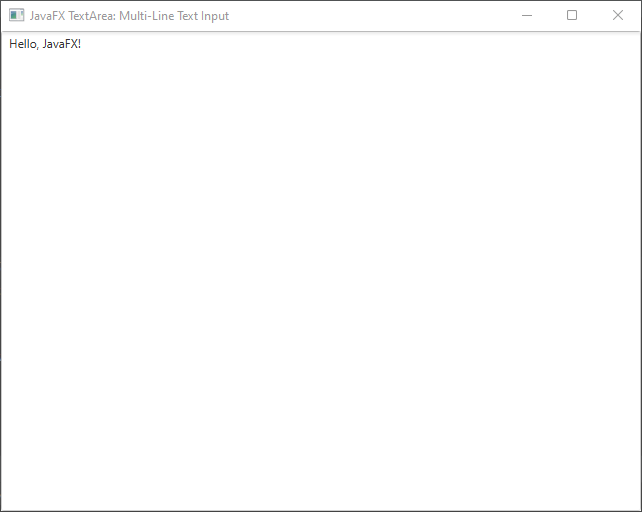
Changing Font and Size
Use the setFont method to change the font and size of the text.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.scene.text.Font;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
// Set initial text
textArea.setText("Hello, JavaFX!");
// Set the Font
textArea.setFont(new Font("Open Sans", 18.0));
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
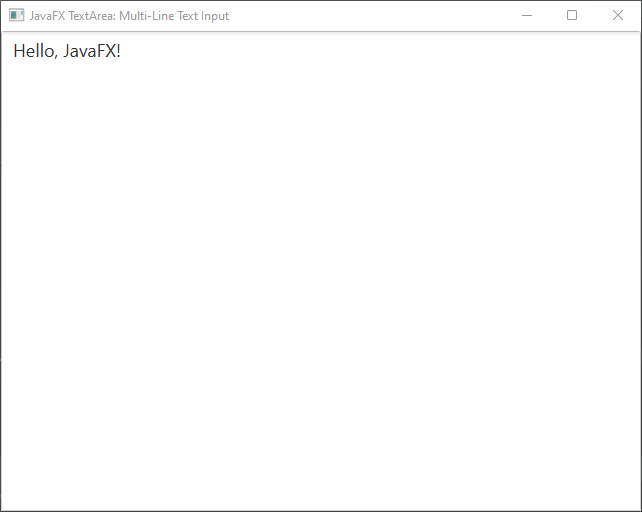
CSS Styling
JavaFX components, including the TextArea, can be styled using CSS. You can define styles in an external CSS file or directly in your code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
// Set initial text
textArea.setText("Hello, JavaFX!");
// Set the CSS Style
textArea.setStyle("-fx-font-size: 18px; -fx-text-fill: red;");
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
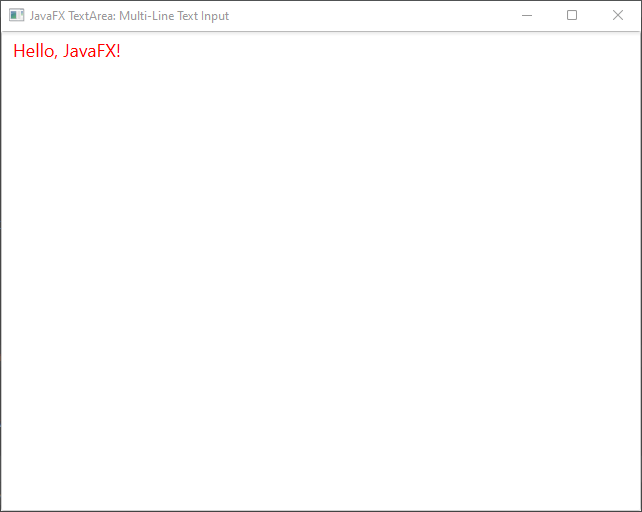
Enabling Word Wrap
You can control whether the text should wrap within the TextArea or extend horizontally beyond its boundaries.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
// Set initial text
textArea.setText("Pellentesque habitant morbi tristique senectus et netus et malesuada " +
"fames ac turpis egestas. Ut nec risus nec turpis facilisis vulputate. Aliquam erat " +
"volutpat. Curabitur a est eros. Vivamus sit amet consectetur arcu. Duis id rhoncus " +
"neque. In sit amet ligula sed diam auctor accumsan. Quisque gravida ullamcorper " +
"ultricies. Class aptent taciti sociosqu ad litora torquent per conubia nostra, per " +
"inceptos himenaeos. Donec condimentum mi vel tortor varius laoreet. Nullam nunc purus, " +
"pharetra at est sed, egestas auctor nunc. Sed faucibus, nulla sed sollicitudin pulvinar, " +
"magna erat placerat mauris, vel hendrerit erat ex eu velit. Vestibulum ante ipsum primis " +
"in faucibus orci luctus et ultrices posuere cubilia curae; Nullam at feugiat metus.");
// Enable text wrapping
textArea.setWrapText(true);
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
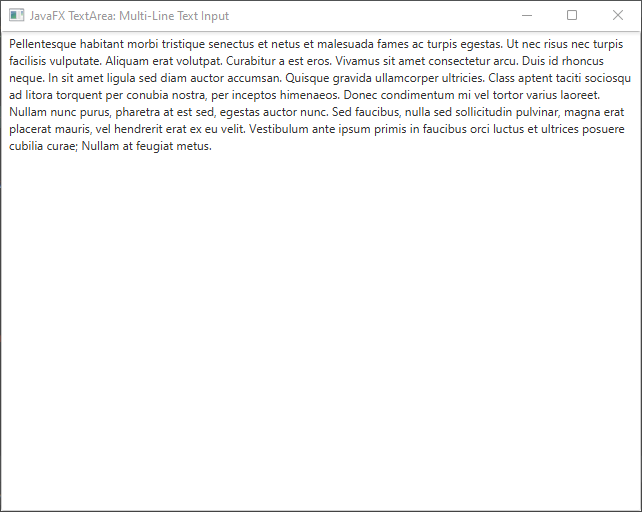
Setting Scrollbars
By default, TextArea automatically adds scrollbars when the text content overflows. You can control the scrollbar visibility explicitly.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
// Set initial text
textArea.setText("Pellentesque habitant morbi tristique senectus et netus et malesuada " +
"fames ac turpis egestas. Ut nec risus nec turpis facilisis vulputate. Aliquam erat " +
"volutpat. Curabitur a est eros. Vivamus sit amet consectetur arcu. Duis id rhoncus " +
"neque. In sit amet ligula sed diam auctor accumsan. Quisque gravida ullamcorper " +
"ultricies. Class aptent taciti sociosqu ad litora torquent per conubia nostra, per " +
"inceptos himenaeos. Donec condimentum mi vel tortor varius laoreet. Nullam nunc purus, " +
"pharetra at est sed, egestas auctor nunc. Sed faucibus, nulla sed sollicitudin pulvinar, " +
"magna erat placerat mauris, vel hendrerit erat ex eu velit. Vestibulum ante ipsum primis " +
"in faucibus orci luctus et ultrices posuere cubilia curae; Nullam at feugiat metus.");
// Set horizontal scroll position
textArea.setScrollLeft(50);
// Set vertical scroll position
textArea.setScrollTop(20);
// Enable text wrapping
textArea.setWrapText(false);
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
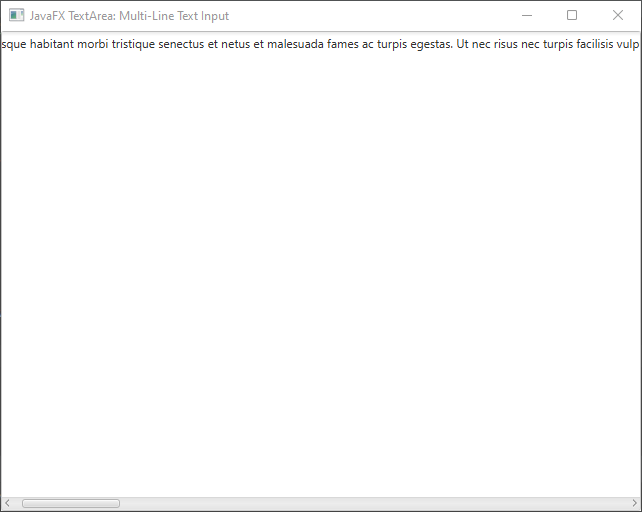
Limiting Input Length
To restrict the number of characters that can be entered in the TextArea, you can use a TextFormatter:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.control.TextFormatter;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
private static final int MAX_LENGTH = 10;
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
textArea.setTextFormatter(new TextFormatter<>(change -> {
if (change.isAdded() && textArea.getText().length() + change.getText().length() > MAX_LENGTH) {
return null;
}
return change;
}));
// Enable text wrapping
textArea.setWrapText(false);
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
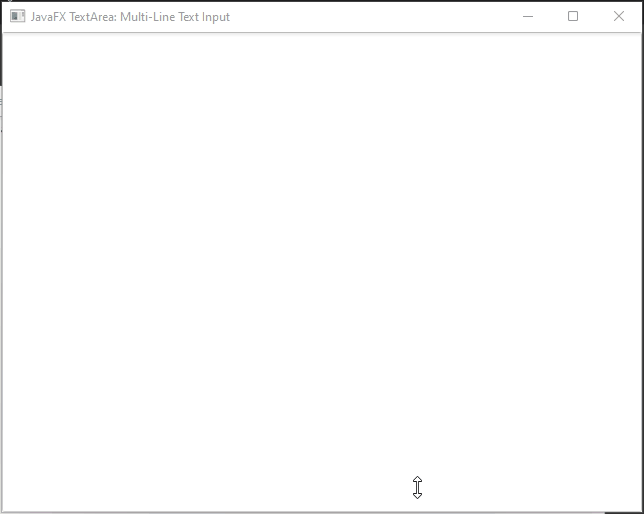
Handling TextArea Events
TextArea supports various event handlers to capture user interactions. Here are a couple of event examples:
Text Change Listener
You can listen for changes to the text content using a ChangeListener. This is useful for implementing auto-save or validation mechanisms.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
private static final int MAX_LENGTH = 10;
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
textArea.textProperty().addListener((observable, oldValue, newValue) -> {
System.out.println("Text changed: " + newValue);
});
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
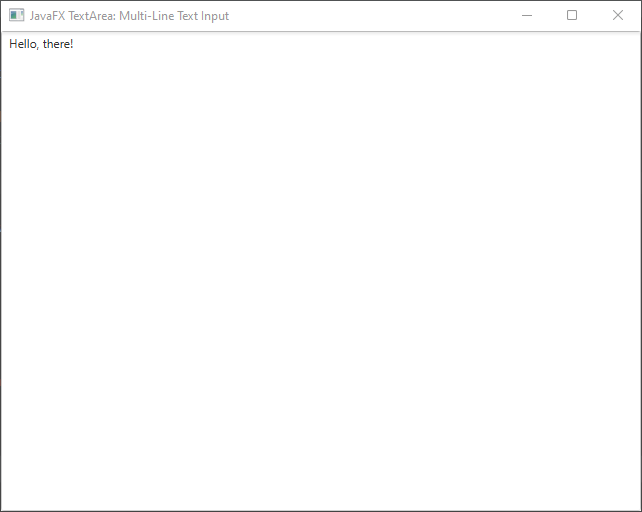
Key Press Event
Capture key press events within the TextArea using the setOnKeyPressed() method.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
private static final int MAX_LENGTH = 10;
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the TextArea
TextArea textArea = new TextArea();
textArea.setOnKeyPressed(event -> System.out.println("Key pressed: " + event.getCode()));
// Add the TextArea to the BorderPane
this.parent.setCenter(textArea);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX TextArea: Multi-Line Text Input");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
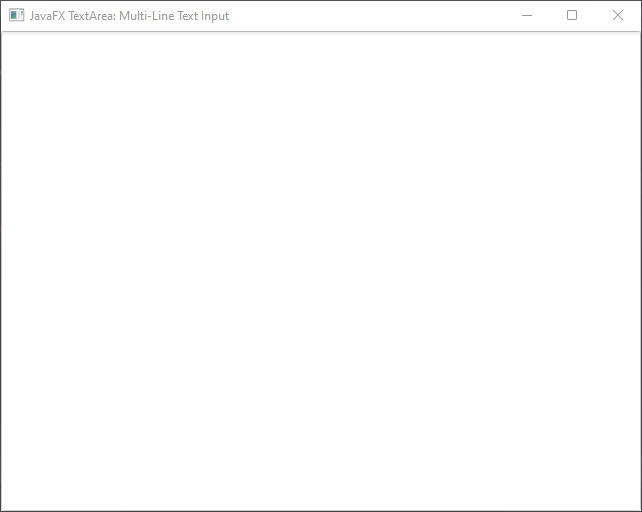
Editing and Interaction
The TextArea supports common text editing interactions, such as copying, cutting, pasting, and undo/redo operations. These can be accessed through the context menu or using keyboard shortcuts.
Conclusion
The JavaFX TextArea is a powerful component that provides multi-line text input capabilities to your Java applications. Whether you’re building a simple note-taking app or a more complex text editor, the TextArea offers a range of features to suit your needs. By following the examples and guidelines provided in this article, you can create interactive and functional text input interfaces using JavaFX.
Sources:
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!