In modern software development, combining web technologies with native applications is becoming increasingly common. This fusion allows developers to leverage the best of both worlds, providing rich user interfaces and integrating web content seamlessly within desktop applications. One such technology that facilitates this synergy is JavaFX WebView.
What is JavaFX WebView?
JavaFX WebView is a component of the JavaFX library that enables developers to embed web content, typically HTML, CSS, and JavaScript, directly into Java applications. It provides a full-featured web browser engine, allowing users to interact with web content and display dynamic web pages within the JavaFX application window.
This powerful tool is particularly useful when you want to integrate web-based functionalities into your Java application without the need for a separate browser window. It opens up a wide range of possibilities, including displaying web-based documentation, integrating web-based tools, or even developing hybrid applications that mix native and web-based components.
Displaying a Simple Web Page
Let’s start with a straightforward example to display a static web page using JavaFX WebView:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
import java.io.IOException;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
WebView webView = new WebView();
webView.getEngine().load("https://www.coderscratchpad.com");
this.parent.setCenter(webView);
this.setupStage(stage);
}
private void setupStage(Stage stage) throws IOException {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX WebView");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
In this example, we create a new JavaFX application, add a WebView component, and load the URL “https://www.coderscratchpad.com” into it. When you run the application, you’ll see the web page displayed within the JavaFX window.
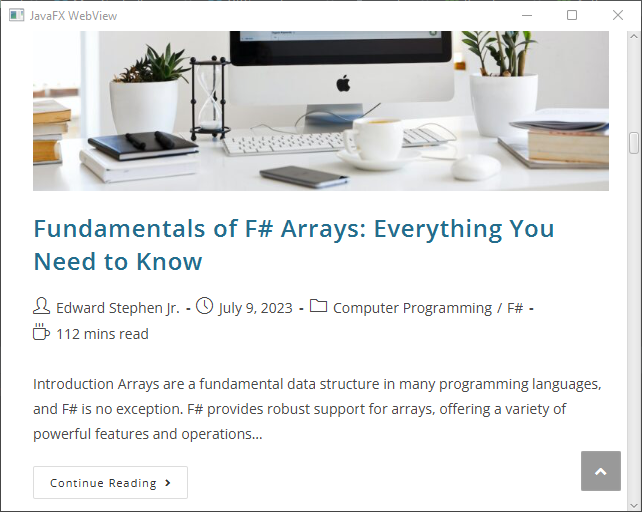
Displaying HTML content
Displaying HTML content using JavaFX WebView is straightforward. In this example, we’ll create a JavaFX application that shows a simple HTML content within the WebView component:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
import java.io.IOException;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
WebView webView = new WebView();
String htmlContent = "<html>" +
"<body>" +
"<h1>Hello, WebView!</h1>" +
"<p>This is an example of displaying HTML content using JavaFX WebView.</p>" +
"</body>" +
"</html>";
webView.getEngine().loadContent(htmlContent);
this.parent.setCenter(webView);
this.setupStage(stage);
}
private void setupStage(Stage stage) throws IOException {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX WebView");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
In this example, we create an HTML string htmlContent, which contains a basic HTML page with a heading and a paragraph. We then use webView.getEngine().loadContent(htmlContent) to load and display this HTML content within the JavaFX window.
When you run the application, you’ll see the HTML content rendered in the JavaFX WebView component, displaying the heading and paragraph as specified in the HTML string.
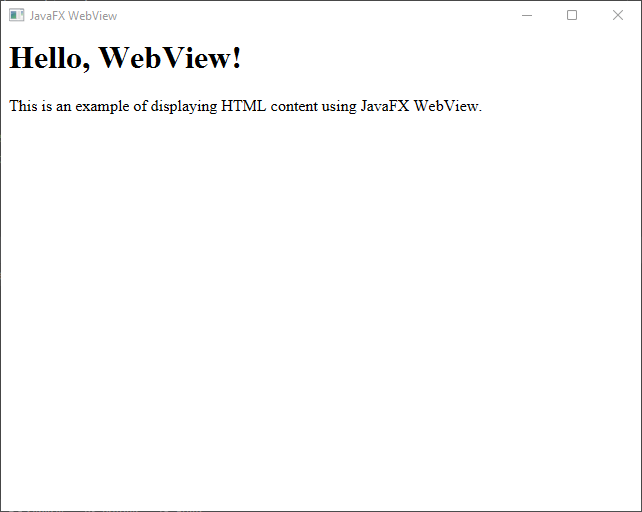
This method is useful when you want to display custom or dynamically generated HTML content within your JavaFX application without the need to load an external URL.
Displaying HTML Files
Displaying HTML files using JavaFX WebView is similar to displaying HTML content. In this example, we’ll load an external HTML file and display its content within the WebView component:
Suppose you have an HTML file named “example.html” with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Example HTML File</title>
</head>
<body>
<h1>Hello from HTML file!</h1>
<p>This content is loaded from an external HTML file using JavaFX WebView.</p>
</body>
</html>
Here’s the JavaFX application that loads and displays the “example.html” file:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
import java.io.IOException;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
WebView webView = new WebView();
String url = String.valueOf(this.getClass().getResource("example.html"));
webView.getEngine().load(url);
this.parent.setCenter(webView);
this.setupStage(stage);
}
private void setupStage(Stage stage) throws IOException {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX WebView");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
Replace “example.html” with the actual file path on your system where “example.html” is located, mine is in the same directory as the Main.java file. When you run the application, the content of the HTML file will be displayed within the JavaFX WebView component.
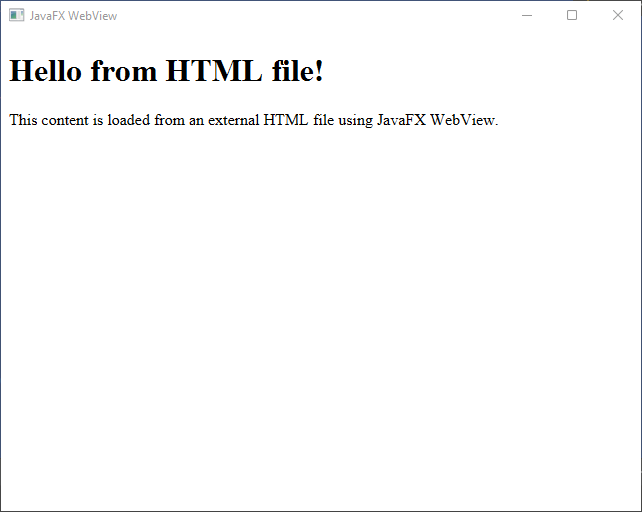
Interacting with JavaScript
JavaFX WebView allows you to communicate with JavaScript running within the web page and vice versa. This enables powerful interactions between the Java application and the embedded web content. Let’s see how we can call a JavaScript function from Java and receive a response:
import javafx.application.Application;
import javafx.concurrent.Worker;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
import java.io.IOException;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
WebView webView = new WebView();
webView.getEngine().loadContent("<html>" +
"<body>" +
"<h1 id='header'>Hello from JavaScript!</h1>" +
"</body>" +
"</html>");
webView.getEngine().getLoadWorker().stateProperty().addListener((ov, oldState, newState) -> {
if (newState == Worker.State.SUCCEEDED) {
String headerText = (String) webView.getEngine().executeScript("document.getElementById('header').innerText");
System.out.println("Header Text: " + headerText);
}
});
this.parent.setCenter(webView);
this.setupStage(stage);
}
private void setupStage(Stage stage) throws IOException {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX WebView");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
This example demonstrates how to load a simple HTML page with a heading and access the header’s text using JavaScript. The Java code executes JavaScript within the WebView, retrieves the text, and prints it to the console.
Controlling WebView Programmatically
Besides interacting with web content, you can also control WebView programmatically. For example, you can navigate to different URLs or execute JavaScript code directly from Java. Here’s an example of programmatically navigating to a URL:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.input.KeyCode;
import javafx.scene.layout.BorderPane;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
import java.io.IOException;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
private WebView webView;
@Override
public void start(Stage stage) throws Exception {
TextField textField = new TextField();
textField.setPromptText("Type a URL");
textField.setOnKeyReleased(keyEvent -> {
if(keyEvent.getCode() == KeyCode.ENTER) {
try {
this.webView.getEngine().load(textField.getText());
} catch(IllegalArgumentException exception) { exception.printStackTrace(); }
}
});
this.webView = new WebView();
// Loads Google by default
this.webView.getEngine().load("https://www.google.com");
this.parent.setTop(textField);
this.parent.setCenter(webView);
this.setupStage(stage);
}
private void setupStage(Stage stage) throws IOException {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX WebView");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
In this example, we load “https://www.google.com” initially and then, programmatically navigate to whatever URL the user types in the text field and presses the enter key.
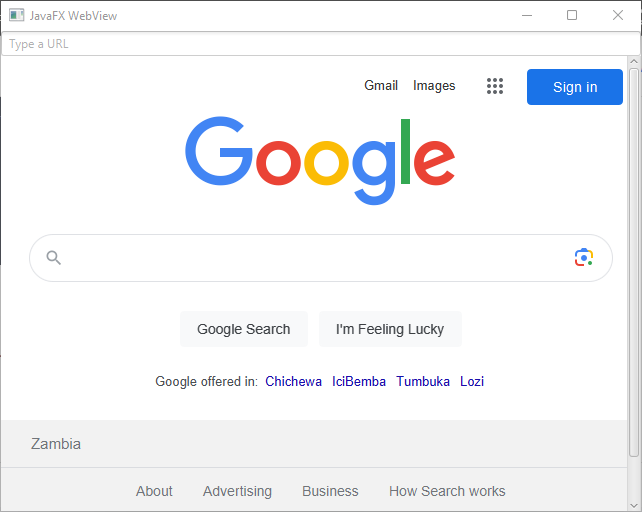
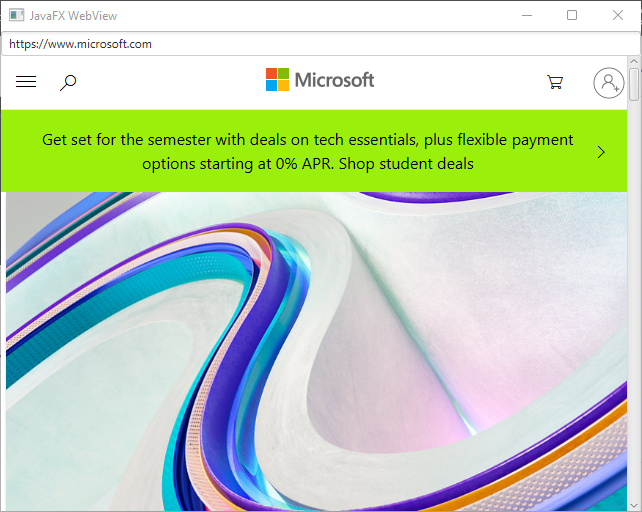
Conclusion
JavaFX WebView is a valuable tool for integrating web content into Java applications seamlessly. Whether you want to display web pages, interact with JavaScript, or control the WebView programmatically, JavaFX WebView empowers developers to create feature-rich and interactive applications.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!