When you run a Flutter app in debug mode, you’ll see a small “DEBUG” banner at the top right of the screen. This banner shows that the app is in debug mode, which is useful for testing and fixing bugs, but it’s not needed when the app is ready for use. If you want to get rid of it, Flutter makes it easy to remove.
Understanding the Debug Banner
The debug banner shows up when the app is in debug mode to let you know that the app isn’t fully optimized for performance yet. Debug mode helps developers by allowing hot reload, showing detailed error messages, and giving extra logs, making it perfect for testing and fixing. But in release mode, which is when the app is ready for users, the banner disappears, and the app is fully optimized.
How to Remove the Debug Banner
The easiest way to remove the debug banner is by setting the debugShowCheckedModeBanner
property to false
in your MaterialApp
or CupertinoApp
widget. Here’s how you can do it:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false, // Hides the debug banner
home: Scaffold(
appBar: AppBar(title: const Text('Flutter App')),
body: const Center(child: Text('Hello, Flutter!')),
),
);
}
}
By setting debugShowCheckedModeBanner: false
, you turn off the debug banner but still keep all the useful features of debug mode, like hot reload and detailed error logs. This way, you can test your app without the banner showing up.
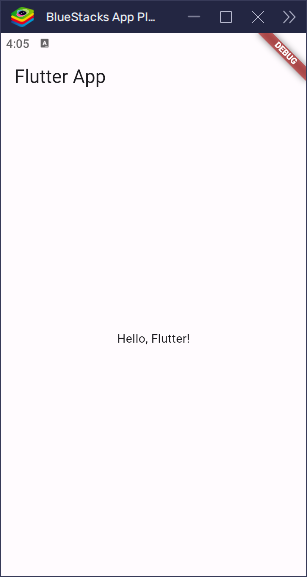
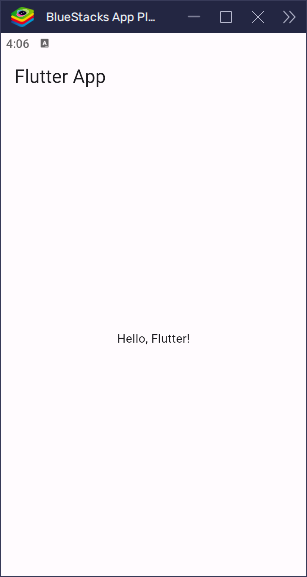
Alternative Methods
Running in Release Mode
Another way to remove the debug banner is by running your app in release mode. The banner only shows up in debug mode, so switching to release mode will make it go away automatically. To run your app in release mode, use this command:
flutter run --release
Release mode makes the app faster and smaller by removing debugging tools. However, you won’t be able to use features like hot reload or see detailed error logs while in release mode.
Using Profile Mode
If you want to remove the debug banner but still need some debugging tools, you can run your app in profile mode:
flutter run --profile
Profile mode helps you test your app’s performance while still giving you some debugging features, like tracing and profiling tools.
Conclusion
The debug banner in Flutter is helpful for development but not needed in production. You can easily remove it by setting debugShowCheckedModeBanner
to false
in your app’s main widget. Alternatively, running the app in release or profile mode will also remove the banner. By understanding the different modes Flutter offers, you can make sure your app runs smoothly in both development and production environments.