In Flutter, the Text
widget is used to display words on the screen. But text is more than just letters and words — the way your text is spaced can make your app look clean, modern, and easy to read.
In this article, we’ll explore how to control text spacing in Flutter using the TextStyle
class. We’ll look at letter spacing, word spacing, and line height, with simple examples to help you understand how they work.
What Is Text Spacing?
Text spacing means adjusting how close or far apart your text elements are:
- Letter spacing: The space between individual letters.
- Word spacing: The space between words.
- Line height: The vertical space between lines of text.
These small adjustments can make a big difference in how readable and beautiful your app looks.
Letter Spacing with letterSpacing
The letterSpacing
property changes the space between each letter.
Here’s a simple example:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Text Spacing')),
body: Center(
child: Padding(
padding: const EdgeInsets.all(12.0),
child: Text(
'Flutter Spacing',
style: TextStyle(letterSpacing: 5.0),
),
),
),
),
);
}
}
This adds extra space between every letter. It’s great for large headings or when you want the text to feel more open.
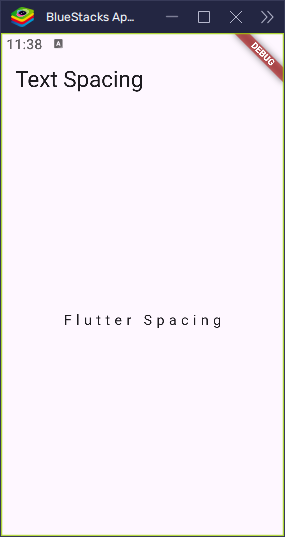
You can use negative values too, like letterSpacing: -1.0
, to bring letters closer together. But be careful not to make it hard to read.
Word Spacing with wordSpacing
The wordSpacing
property controls the space between words.
Example:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Text Spacing')),
body: Center(
child: Padding(
padding: const EdgeInsets.all(12.0),
child: Text(
'Flutter makes apps fast and beautiful.',
style: TextStyle(wordSpacing: 10.0),
),
),
),
),
);
}
}
In this case, there’s more space between each word, making the sentence look airy. You can use this to improve layout in banners, labels, or titles.
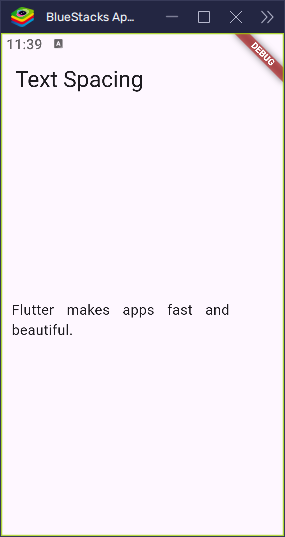
Line Height with height
The height
property (also called line height) adjusts the space between lines of multi-line text. It multiplies the font size.
For example:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Text Spacing')),
body: Center(
child: Padding(
padding: const EdgeInsets.all(12.0),
child: Text(
'This is line one.\nThis is line two.',
style: TextStyle(height: 2.0, fontSize: 16),
),
),
),
),
);
}
}
Here, the space between the lines will be twice the font size (16 × 2 = 32 pixels between lines). This is helpful when showing paragraphs, lists, or any block of text that needs to be easy to read.
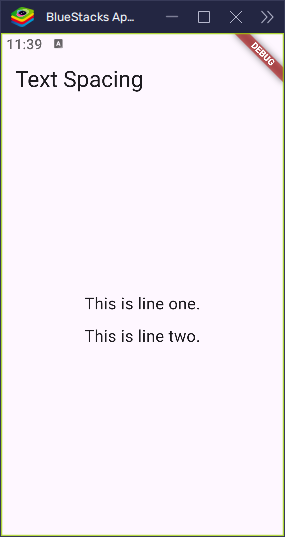
Real-World Use Cases
Here are some common places where text spacing matters:
- Titles and Headings: Use letter spacing to give them a bold, modern look.
- Paragraphs: Adjust line height to improve readability.
- Buttons and Labels: Use word spacing to make short text look clean and balanced.
Example – a styled heading:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Text Spacing')),
body: Center(
child: Padding(
padding: const EdgeInsets.all(12.0),
child: Text(
'WELCOME',
style: TextStyle(
fontSize: 28,
fontWeight: FontWeight.bold,
letterSpacing: 4,
),
),
),
),
),
);
}
}
This gives the heading a spaced-out, elegant feel.
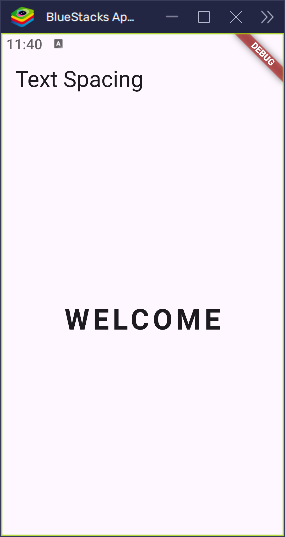
Tips for Better Text Layout
- Don’t use too much spacing. If letters or lines are too far apart, the text becomes harder to read.
- Always test on real devices with different screen sizes.
- Try combining spacing with other
TextStyle
features like font size, weight, and color to create beautiful text.
Conclusion
In Flutter, controlling text spacing is easy and powerful. You can use:
letterSpacing
to adjust the space between letters.wordSpacing
to control the space between words.height
to manage line spacing for multi-line text.
These small changes can make a big difference in how your app feels to users. So go ahead, play with spacing, and make your text stand out in the best way!