Graphical User Interfaces (GUIs) play a vital role in modern software development, providing users with an interactive and visually appealing way to interact with applications. PyQt, a set of Python bindings for the popular Qt framework, enables developers to create powerful and cross-platform GUI applications effortlessly. Among the plethora of widgets available in PyQt, the QLabel widget stands out as a versatile tool for displaying text and images. In this article, we will explore the PyQt QLabel widget and provide a code example to demonstrate its functionality.
Introducing the QLabel Widget
The QLabel widget in PyQt allows developers to display text or images on the GUI. It is one of the fundamental widgets that can be used to build more complex and interactive applications. QLabel supports various features like text alignment, word wrapping, rich text formatting, image display, and even links.
Key Features of QLabel Widget
- Text Display: QLabel can display simple plain text or support rich text formatting using HTML-like tags, enabling bold, italic, and other text styles.
- Image Display: Besides displaying text, QLabel can also display images in various formats such as PNG, JPEG, GIF, and more.
- Text Alignment: Developers can set the alignment of the text displayed within the QLabel, enabling proper text positioning.
- Word Wrapping: QLabel supports word wrapping, ensuring that text automatically wraps to fit within the specified boundaries.
- Hyperlinks: QLabel can handle clickable hyperlinks, allowing users to navigate to external URLs or perform specific actions.
- Accessibility: QLabel provides accessibility support, making it easier for users with disabilities to interact with the application.
Basic Usage of the QLabel Widget
To get started with the QLabel widget, ensure you have PyQt installed. If not, you can install it using the following pip command:
pip install PyQt6
Now, let’s dive into a basic example demonstrating how to create a simple PyQt application with a QLabel widget that displays text.
import sys
from PyQt6.QtCore import Qt
from PyQt6.QtGui import QFont
from PyQt6.QtWidgets import QApplication, QMainWindow, QLabel
class AppWindow(QMainWindow):
def __init__(self, title, width, height, left=100, top=100):
super().__init__()
self.setWindowTitle(title)
# Set the window location and size
self.setGeometry(left, top, width, height)
self.initUI()
def initUI(self):
# Create a QLabel widget and set its text
label = QLabel("Hello, PyQt QLabel!")
# Set alignment and font properties
label.setAlignment(Qt.AlignmentFlag.AlignCenter)
label.setFont(QFont("Arial", 18))
# Add the QLabel widget to the main window
self.setCentralWidget(label)
def center(self):
# Get the geometry of the screen
screen = QApplication.primaryScreen().size()
# Calculate the center point of the screen
x = (screen.width() - self.width()) // 2
y = (screen.height() - self.height()) // 2
# Move the window to the center
self.move(x, y)
if __name__ == "__main__":
app = QApplication(sys.argv)
# Create new app window with title, and dimensions
window = AppWindow("The PyQt QLabel Widget: Text and Image Display Tool", 640, 480)
# Center app window on screen
window.center()
# show app window on screen
window.show()
sys.exit(app.exec())
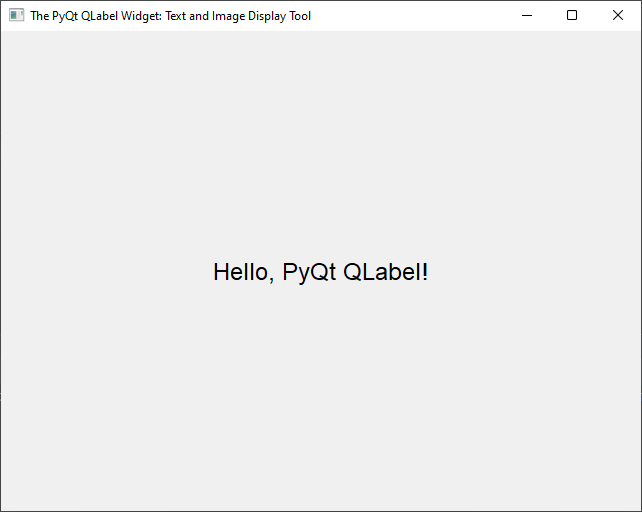
In this example, we create a simple QMainWindow subclass named AppWindow. Inside the window, we create a QLabel widget, set its text content, and customize its alignment and font properties. The setCentralWidget() method is used to add the QLabel widget to the main window. Finally, the application is run using the QApplication class.
Displaying Images with the QLabel Widget
Apart from text, the QLabel widget is also capable of displaying images. To illustrate this, let’s modify our example to load and display an image using the QLabel widget.
import sys
from PyQt6.QtCore import Qt
from PyQt6.QtGui import QPixmap
from PyQt6.QtWidgets import QApplication, QMainWindow, QLabel
class AppWindow(QMainWindow):
def __init__(self, title, width, height, left=100, top=100):
super().__init__()
self.setWindowTitle(title)
# Set the window location and size
self.setGeometry(left, top, width, height)
self.initUI()
def initUI(self):
# Load an image and create a QLabel widget to display it
pixmap = QPixmap("image.jpeg")
label = QLabel()
label.setPixmap(pixmap)
# Align label contents to the center
label.setAlignment(Qt.AlignmentFlag.AlignCenter)
# Add the QLabel widget to the main window
self.setCentralWidget(label)
def center(self):
# Get the geometry of the screen
screen = QApplication.primaryScreen().size()
# Calculate the center point of the screen
x = (screen.width() - self.width()) // 2
y = (screen.height() - self.height()) // 2
# Move the window to the center
self.move(x, y)
if __name__ == "__main__":
app = QApplication(sys.argv)
# Create new app window with title, and dimensions
window = AppWindow("The PyQt QLabel Widget: Text and Image Display Tool", 640, 480)
# Center app window on screen
window.center()
# show app window on screen
window.show()
sys.exit(app.exec())
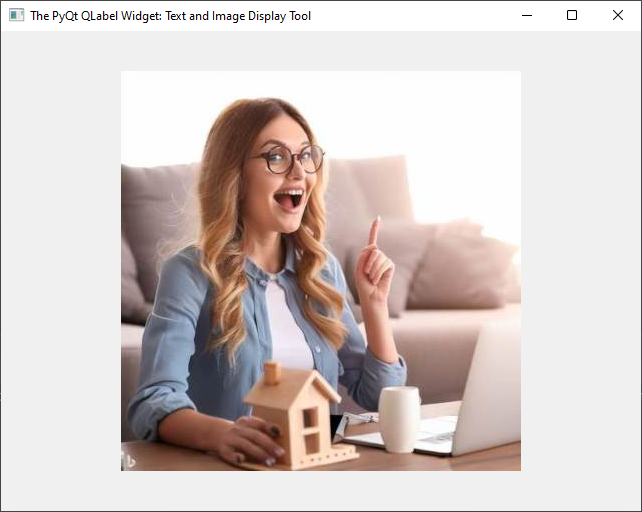
In this modified example, we load an image named “image.jpeg” using the QPixmap class and set it as the pixmap for the QLabel widget. This allows us to display the image within the main window. Make sure to replace “image.jpeg” with the path to an actual image file on your system.
Clickable Hyperlinks
In PyQt, you can use a QLabel to display clickable hyperlinks, which enables users to navigate to external URLs or perform custom actions when the hyperlink is clicked. To achieve this functionality, you need to use the QLabel’s setOpenExternalLinks(True) method, which tells PyQt to open the hyperlink in the default web browser when clicked.
Here’s an example of how to create a clickable hyperlink in a QLabel:
import sys
from PyQt6.QtCore import Qt
from PyQt6.QtWidgets import QApplication, QMainWindow, QLabel
class AppWindow(QMainWindow):
def __init__(self, title, width, height, left=100, top=100):
super().__init__()
self.setWindowTitle(title)
# Set the window location and size
self.setGeometry(left, top, width, height)
self.initUI()
def initUI(self):
# Create the QLabel
label = QLabel()
label.setText('<a href="https://www.coderscratchpad.com">Click me!</a>')
# Allow opening links in the default browser
label.setOpenExternalLinks(True)
# Align label contents to the center
label.setAlignment(Qt.AlignmentFlag.AlignCenter)
# Add the QLabel widget to the main window
self.setCentralWidget(label)
def center(self):
# Get the geometry of the screen
screen = QApplication.primaryScreen().size()
# Calculate the center point of the screen
x = (screen.width() - self.width()) // 2
y = (screen.height() - self.height()) // 2
# Move the window to the center
self.move(x, y)
if __name__ == "__main__":
app = QApplication(sys.argv)
# Create new app window with title, and dimensions
window = AppWindow("The PyQt QLabel Widget: Text and Image Display Tool", 640, 480)
# Center app window on screen
window.center()
# show app window on screen
window.show()
sys.exit(app.exec())
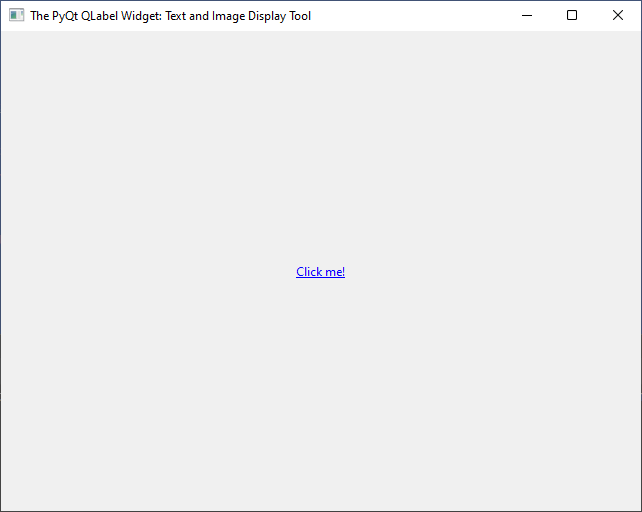
In this PyQt example, we create a QLabel widget and embed an HTML link (anchor tag) within its text, with the href attribute pointing to an external URL. By calling setOpenExternalLinks(True), we ensure that when users click the “Click me!” link in the QLabel, it will seamlessly open the specified URL in their default web browser. To enhance the visual presentation, we center-align the label text using the Qt.AlignmentFlag.AlignCenter alignment setting. This straightforward implementation makes QLabel a powerful tool for incorporating clickable hyperlinks into PyQt applications, enhancing user interactivity and navigation.
Conclusion
The PyQt QLabel widget is a versatile tool that enables developers to display both text and images within GUI applications. Its flexibility and various features make it a powerful element to enhance the user experience. In this article, we explored the key features of the QLabel widget and provided a practical code example to demonstrate its usage. As you continue to explore PyQt and develop GUI applications, you’ll find QLabel to be an invaluable asset in your toolkit.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!