JavaFX is a versatile library for creating graphical user interfaces and visual applications in Java. One of the fundamental features it offers is the Canvas class, which allows you to draw custom graphics, shapes, and images. In this article, we will explore how to draw arcs in JavaFX Canvas, complete with code examples.
Understanding Arcs
Arcs are segments of a circle, often used in various graphical applications for tasks such as creating pie charts or indicating progress. In JavaFX, arcs can be easily drawn on a Canvas using the GraphicsContext class, which provides methods for drawing shapes and paths.
Drawing an Arc
To draw an arc on the Canvas, you’ll need to use the strokeArc or fillArc methods provided by the GraphicsContext. These methods allow you to specify the starting angle, extent (angle range), center coordinates, width, and height of the arc. You can also set the stroke and fill colors as desired.
Drawing Filled Arcs
Here’s an example of how to draw a filled arc:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.ArcType;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Define arc parameters
double centerX = 200;
double centerY = 200;
double radiusX = 100;
double radiusY = 100;
double startAngle = 45; // in degrees
double arcExtent = 90; // in degrees
// Set the fill color for the arc
gc.setFill(Color.BLUE);
// Draw a filled arc on the Canvas
gc.fillArc(
centerX - radiusX,
centerY - radiusY,
2 * radiusX,
2 * radiusY,
startAngle,
arcExtent,
ArcType.ROUND
);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Arcs in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
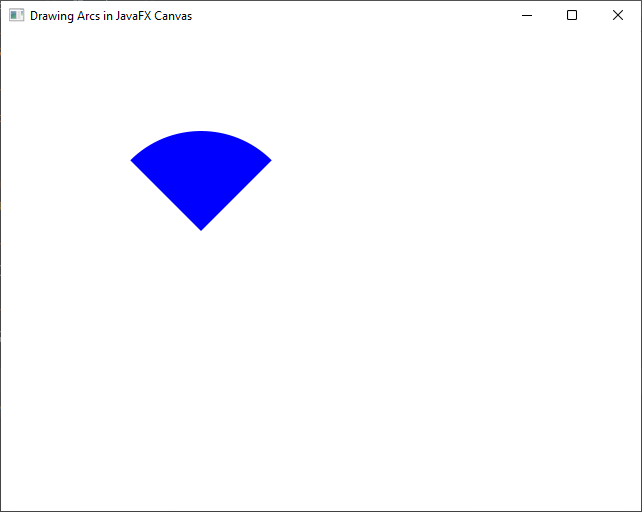
In this example, we’ve drawn a blue filled arc with a center at (200, 200), a horizontal radius of 100, and a vertical radius of 100. The arc starts at an angle of 45 degrees and spans 90 degrees. The ArcType.ROUND argument specifies that the arc is closed with rounded edges, making it look like a pie slice.
Drawing Stroked Arcs
If you want to draw just the outline of an arc, you can use the strokeArc method instead of fillArc:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.ArcType;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Define arc parameters
double centerX = 200;
double centerY = 200;
double radiusX = 100;
double radiusY = 100;
double startAngle = 45; // in degrees
double arcExtent = 90; // in degrees
// Set the stroke color for the arc
gc.setStroke(Color.RED);
gc.setLineWidth(3.0);
// Draw a stroked arc on the Canvas
gc.strokeArc(
centerX - radiusX,
centerY - radiusY,
2 * radiusX,
2 * radiusY,
startAngle,
arcExtent,
ArcType.OPEN
);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Arcs in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
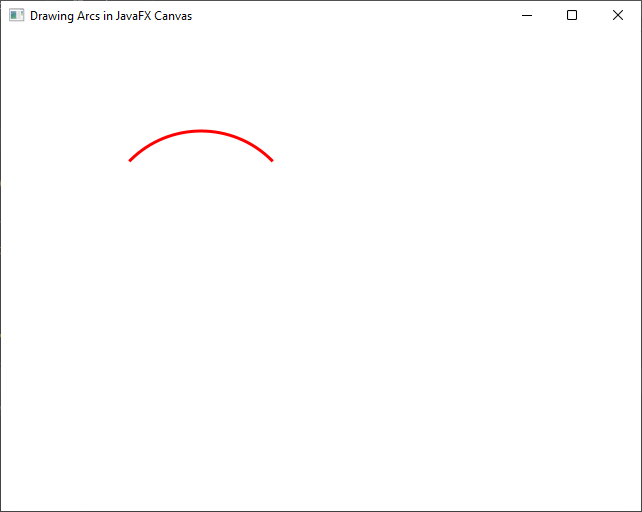
In this case, we’ve drawn a red stroked arc with a line width of 3.0. The ArcType.OPEN argument specifies that the arc is not closed, and it’s open-ended.
Another arc type is CHORD, which specifies that the arc is closed by drawing straight line segments from the start point to the center and then to the end point.
Conclusion
Drawing arcs in JavaFX is straightforward using the Canvas and GraphicsContext classes. You can customize the appearance of arcs by specifying their properties, such as start angle, extent, center coordinates, and colors. This flexibility allows you to create various graphical elements and visual representations in your JavaFX applications. Remember to check the JavaFX Canvas Documentation for more.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!