Creating visually appealing graphical user interfaces (GUIs) is an essential aspect of software development. PyQt, a set of Python bindings for the Qt application framework, offers a wide range of tools to design and customize GUI applications. One often overlooked but important customization is setting a window icon. In this article, we will explore how to set a custom icon for your PyQt application’s window.
Why Set a Window Icon?
A window icon is a small image that represents your application in the taskbar, title bar, and system tray. It is a crucial aspect of branding your application and making it stand out. The default window icon is typically a generic one, but setting a custom icon can make your application look more professional and recognizable.
Prerequisites
Before we dive into setting a custom icon for your PyQt window, ensure that you have PyQt installed on your system. You can install it using pip:
pip install PyQt6
Setting a Custom Window Icon
Preparing the Icon
To set a custom icon for your PyQt window, you’ll need an image file in one of the supported formats, such as .png, .ico, or .svg. The icon image should be square for best results and should have a resolution of at least 32×32 pixels.
Writing the PyQt Application
Here’s the code that demonstrates how to set a custom window icon in a PyQt application:
import sys
from PyQt6.QtGui import QIcon
from PyQt6.QtWidgets import *
# Create a QMainWindow subclass
class AppWindow(QMainWindow):
def __init__(self, title, width, height, left=100, top=100):
super().__init__()
# Set the window title
self.setWindowTitle(title)
# Set the window location and size
self.setGeometry(left, top, width, height)
def center(self):
# Get the geometry of the screen
screen = QApplication.primaryScreen().size()
# Calculate the center point of the screen
x = (screen.width() - self.width()) // 2
y = (screen.height() - self.height()) // 2
# Move the window to the center
self.move(x, y)
if __name__ == "__main__":
app = QApplication(sys.argv)
# Create a new app window with title and dimensions
window = AppWindow("Setting PyQt Window Icon", 640, 480)
# Replace with the actual path to your icon
icon = QIcon("icon.png")
window.setWindowIcon(icon)
# Center the app window on the screen
window.center()
# Show the app window on the screen
window.show()
# Run the application event loop
sys.exit(app.exec())
Make sure to replace ‘icon.png’ with the actual path to your icon file. PyQt supports various image formats, so you can use .ico, .png, .svg, or other compatible formats. This code creates a basic PyQt window and sets the window title and icon.
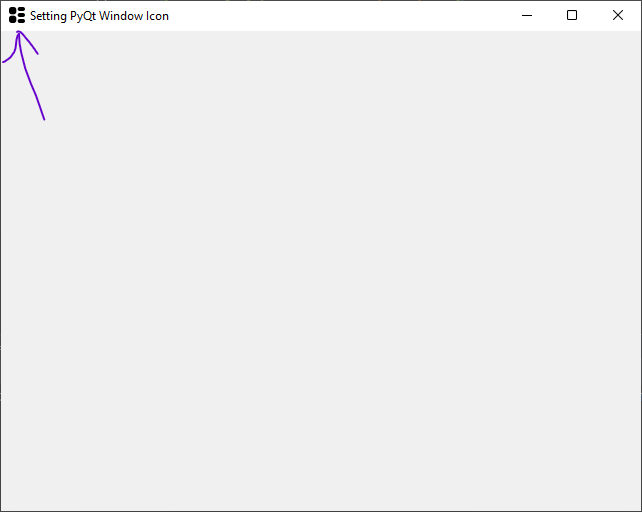
Best Practices for Choosing a Window Icon
When selecting or designing an icon for your application, consider the following best practices:
Keep it Simple: Icons should be easily recognizable at various sizes, so avoid complex designs.
Consistency: Maintain consistency with your application’s branding and style.
Transparency: Use transparency where appropriate to allow the icon to blend well with different backgrounds.
Testing: Test your icon at various sizes to ensure it remains clear and appealing.
Conclusion
Setting a custom icon for your PyQt window is a straightforward process. Icons enhance the visual appeal of your application and make it more recognizable. Experiment with different icons and make your PyQt-based applications more visually appealing to your users.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.