In the world of modern software development, user experience is paramount. Users expect applications to be intuitive and efficient, and one crucial aspect of this experience is the clipboard functionality. Clipboard operations, such as copying and pasting, are fundamental to the user’s ability to interact with data. In the JavaFX framework, clipboard functionality is seamlessly integrated, making it easy for developers to provide a smooth and user-friendly experience. In this article, we will explore JavaFX’s clipboard capabilities and learn how to implement clipboard operations in your JavaFX applications.
Understanding the Clipboard
Before diving into JavaFX’s clipboard features, let’s start with a brief overview of what the clipboard is and how it works. The clipboard is a temporary storage area for data that users can copy and paste within or between applications. It typically stores various data formats, such as text, images, and more. Users can copy data to the clipboard using a “Copy” command and paste it using a “Paste” command.
In JavaFX, the clipboard functionality allows you to work with the system clipboard, enabling your application to interact with data from other applications and share data with them.
Clipboard Class in JavaFX
JavaFX provides the Clipboard class to interact with the clipboard. You can use this class to perform the following operations:
Copy Data to the Clipboard
To copy data to the clipboard, you can use the Clipboard class along with its setContent method. Here’s an example of how to copy a text string to the clipboard:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.ContextMenu;
import javafx.scene.control.Label;
import javafx.scene.control.MenuItem;
import javafx.scene.input.Clipboard;
import javafx.scene.input.ClipboardContent;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
// Get the system clipboard
private final Clipboard clipboard = Clipboard.getSystemClipboard();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a label with text
Label copyLabel = new Label("Hello, Clipboard!");
// Create a "Copy" menu item
MenuItem copyMenuItem = new MenuItem("Copy");
copyMenuItem.setOnAction(e -> {
// Create ClipboardContent to hold data
ClipboardContent content = new ClipboardContent();
// Set the label's text as a string in ClipboardContent
content.putString(copyLabel.getText());
// Set the ClipboardContent to the clipboard
clipboard.setContent(content);
});
// Create a context menu with the "Copy" menu item
ContextMenu cxtMenu = new ContextMenu(copyMenuItem);
// Attach the context menu to the label
copyLabel.setContextMenu(cxtMenu);
// Create an HBox container to hold the label
HBox container = new HBox(20, copyLabel);
// Center-align the container contents
container.setAlignment(Pos.CENTER);
// Add the container to the StackPane
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Clipboard");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we have created a label with the text “Hello, Clipboard!” When users right-click on the label, a context menu appears, presenting them with a “Copy” option. Once the “Copy” option is selected, the label’s text is promptly copied to the system clipboard.
Paste Data from the Clipboard
To paste data from the clipboard, you can retrieve the clipboard’s content using the Clipboard class and then check if it contains the data format you need. Here’s an example of how to paste text from the clipboard:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.ContextMenu;
import javafx.scene.control.Label;
import javafx.scene.control.MenuItem;
import javafx.scene.input.Clipboard;
import javafx.scene.input.ClipboardContent;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
// Get the system clipboard
private final Clipboard clipboard = Clipboard.getSystemClipboard();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a label with text for copying
Label copyLabel = new Label("Hello, Clipboard!");
// Create a "Copy" menu item
MenuItem copyMenuItem = new MenuItem("Copy");
// Define action for the "Copy" menu item
copyMenuItem.setOnAction(e -> {
ClipboardContent content = new ClipboardContent();
// Copy the label's text to the clipboard
content.putString(copyLabel.getText());
this.clipboard.setContent(content);
});
// Create a context menu with the "Copy" menu item for the copying label
ContextMenu copyContextMenu = new ContextMenu(copyMenuItem);
// Attach the context menu to the copying label
copyLabel.setContextMenu(copyContextMenu);
// Create a label for pasting
Label pasteLabel = new Label("Paste Here!");
// Create a "Paste" menu item
MenuItem pasteMenuItem = new MenuItem("Paste");
// Define action for the "Paste" menu item
pasteMenuItem.setOnAction(e -> {
if (this.clipboard.hasString()) {
String text = this.clipboard.getString();
pasteLabel.setText(text);
}
});
// Create a context menu with the "Paste" menu item for the pasting label
ContextMenu pasteContextMenu = new ContextMenu(pasteMenuItem);
// Attach the context menu to the pasting label
pasteLabel.setContextMenu(pasteContextMenu);
// Create an HBox container to hold both labels
HBox container = new HBox(20, copyLabel, pasteLabel);
// Center-align the container contents
container.setAlignment(Pos.CENTER);
// Add the Container to the parent layout (StackPane)
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Clipboard");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we’ve developed an application consisting of two labels: one labeled “Hello, Clipboard!” and the other as “Paste Here!” When you right-click on the “Hello, Clipboard!” label, a context menu appears with a “Copy” option. Selecting “Copy” efficiently copies the label’s text to the system clipboard.
The “Paste Here!” label, on the other hand, has its own context menu with a “Paste” option. Clicking “Paste” checks if there is a text string in the clipboard, and if so, it displays the copied text in the “Paste Here!” label.
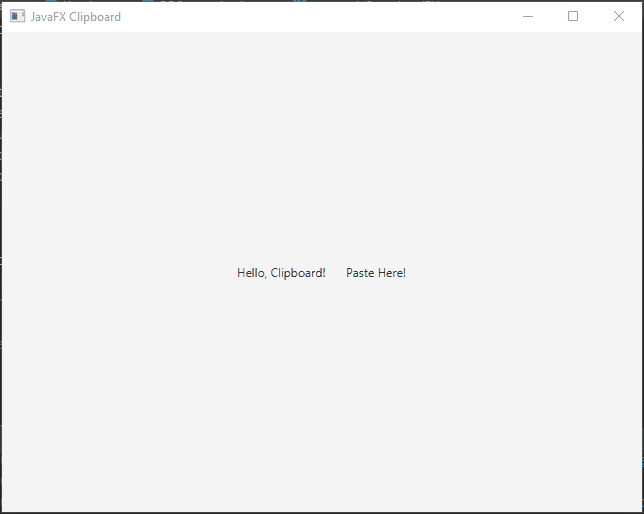
Clearing the Clipboard
Clearing the clipboard is a straightforward task in JavaFX. You can remove the contents from the clipboard by setting it to an empty ClipboardContent object or by using the clear method provided by the Clipboard class. Here’s how you can clear the clipboard:
Setting the Clipboard Content to an Empty ClipboardContent Object
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.input.Clipboard;
import javafx.scene.input.ClipboardContent;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
// Get the system clipboard
private final Clipboard clipboard = Clipboard.getSystemClipboard();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a button to clear the clipboard
Button clearClipboardButton = new Button("Clear Clipboard");
// Define action for the "Clear Clipboard" button
clearClipboardButton.setOnAction(e -> {
ClipboardContent content = new ClipboardContent();
// Set the clipboard content to an empty ClipboardContent object (clearing it)
this.clipboard.setContent(content);
});
// Add the "Clear Clipboard" Button to the parent layout
this.parent.getChildren().add(clearClipboardButton);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Clipboard");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this method, you create a new ClipboardContent object with no data and set it as the clipboard’s content. This effectively clears the clipboard, as it no longer contains any data.
Using the clear Method
The Clipboard class also provides a clear method that you can use to remove all data from the clipboard:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.input.Clipboard;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
// Get the system clipboard
private final Clipboard clipboard = Clipboard.getSystemClipboard();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create a button to clear the clipboard
Button clearClipboardButton = new Button("Clear Clipboard");
// Define action for the "Clear Clipboard" button
clearClipboardButton.setOnAction(e -> {
// Clear the system clipboard
this.clipboard.clear();
});
// Add the "Clear Clipboard" Button to the parent layout
this.parent.getChildren().add(clearClipboardButton);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Clipboard");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
Using the clear method is a more direct way to empty the clipboard, and it achieves the same result as setting an empty ClipboardContent object.
Supporting Multiple Data Formats
The clipboard can hold various data formats. To support multiple formats, you can use methods like hasHtml, hasImage, or hasFiles and then retrieve the data using corresponding methods like getHtml, getImage, or getFiles. This allows your application to work with a wide range of data types.
Here’s an example of how to display Files on the Clipboard in a ListView:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ListView;
import javafx.scene.input.Clipboard;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import java.io.File;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
// Get the system clipboard
private final Clipboard clipboard = Clipboard.getSystemClipboard();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Create an observable list to hold files
ObservableList<File> fileList = FXCollections.observableArrayList();
// Create a ListView to display the files
ListView<File> listView = new ListView<>(fileList);
// Create a button to clear/paste files
Button pasteFilesButton = new Button("Clear/Paste Files");
// Define action for the "Clear/Paste Files" button
pasteFilesButton.setOnAction(e -> {
if(this.clipboard.hasFiles()) {
fileList.clear();
fileList.addAll(this.clipboard.getFiles());
}
});
// Create a VBox to hold the ListView and button
VBox container = new VBox(30, listView, pasteFilesButton);
// Center-align the container contents
container.setAlignment(Pos.CENTER);
// Add the VBox container to the parent layout (StackPane)
this.parent.getChildren().add(container);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Clipboard");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this code example, we’ve developed a file management interface that includes a ListView for file display and a “Clear/Paste Files” button. When the button is clicked, it checks the system clipboard for files, clears the existing list, and pastes the newly found files into it.
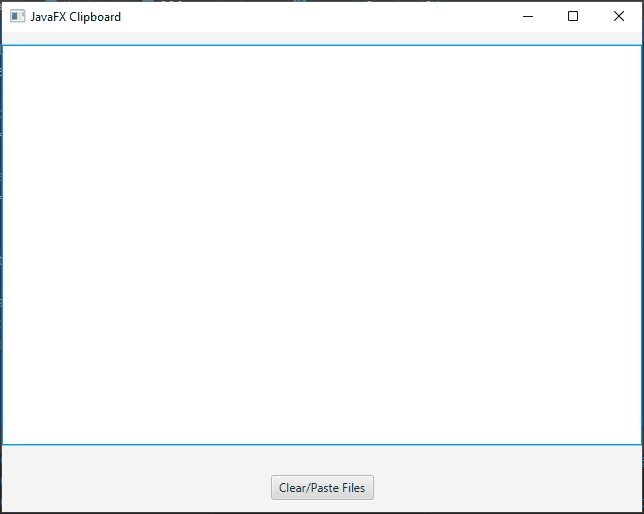
Security Considerations
While clipboard functionality can greatly enhance user experience, it’s essential to handle user data responsibly. Be mindful of security concerns, as sensitive information can be inadvertently copied to the clipboard. Ensure that your application respects user privacy and does not expose confidential data.
Conclusion
Clipboard operations are a fundamental part of modern software applications, and JavaFX makes it easy to implement these features. By utilizing the Clipboard class and listening to clipboard events, you can provide a seamless and user-friendly experience in your JavaFX applications. Whether you’re building a text editor, image editor, or any other application that involves copying and pasting data, JavaFX’s clipboard functionality is a valuable tool in your development toolkit.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.