JavaFX, a popular Java library for creating rich desktop and web applications, offers a wide range of features and effects to enhance the visual appeal of your user interfaces. One of these powerful features is the Light.Spot effect, which allows you to create realistic lighting effects in your JavaFX applications. In this article, we’ll explore the Light.Spot effect, understand how it works, and learn how to use it in your JavaFX applications.
Introduction to Lighting Effects in JavaFX
Lighting effects are essential for creating visually engaging and immersive user interfaces. They add depth, dimension, and realism to your application’s graphical elements. JavaFX provides a comprehensive set of lighting effects, including Light.Spot, which simulates the behavior of a spotlight.
Understanding Light.Spot
The Light.Spot class is part of the JavaFX javafx.scene.effect package, and it represents a point light source with characteristics similar to a spotlight. When you apply the Light.Spot effect to a graphical node, it illuminates the node as if it were being lit by a spotlight.
Key Properties of Light.Spot
To effectively use the Light.Spot effect, it’s crucial to understand its key properties:
- Position (X, Y, Z): The Light.Spot effect is positioned in 3D space. You can control its X, Y, and Z coordinates to determine where the spotlight is located in your scene.
- Direction (Points At X, Points At Y, Points At Z): The spotlight has a direction in which it points. You can specify the X, Y, and Z coordinates that the spotlight should point towards.
- Color: You can define the color of the light emitted by the spotlight, allowing you to create various lighting moods and atmospheres in your application.
- Specular Exponent: This property controls the sharpness or softness of the light’s specular highlights. Higher values make the highlights sharper.
Creating a Light.Spot Effect
To demonstrate the Light.Spot effect, we’ll create a simple JavaFX application that uses this effect to illuminate an image. Here is a simple example application that demonstrates how to use Light.Spot:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.effect.Light;
import javafx.scene.effect.Lighting;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class SpotLightApp extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
// The parent layout manager for the application's UI
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("Lighting Effect: Light.Spot");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
// Load an image from a file
Image image = new Image("scorpion.jpg");
// Create an ImageView for the loaded image
ImageView imageView = new ImageView(image);
imageView.setPreserveRatio(true);
imageView.setFitWidth(250);
// Create a SpotLight source
Light.Spot spotLight = new Light.Spot();
// Create a Lighting effect and set the light source
Lighting lighting = new Lighting();
lighting.setLight(spotLight);
// Apply the Lighting effect to the ImageView
imageView.setEffect(lighting);
// Add the ImageView to the BorderPane's center region
this.parent.setCenter(imageView);
// Create a control panel for configuring the Lighting and SpotLight properties
LightingControlPanel lightingControlPanel = new LightingControlPanel(lighting, spotLight);
this.parent.setLeft(lightingControlPanel);
}
}
In this application, we create a Light.Spot instance to simulate a spotlight source and a Lighting effect to apply this light source to the ImageView. The result is an illuminated image that simulates the effect of a spotlight shining on it. Additionally, we’ve created a control panel, LightingControlPanel, which allows users to adjust the properties of both the Lighting effect and the Light.Spot effect. This control panel is placed on the left side of the application window.
Configuring Light.Spot Properties
Light.Spot provides a range of properties that you can customize to control the appearance and behavior of your spotlight effect. These properties include:
- Position (X, Y, Z): Specifies the location of the spotlight in the 3D coordinate system.
- Direction (Points At X, Points At Y, Points At Z): Defines the direction in which the spotlight points.
- Color: Sets the color of the spotlight.
- Specular Exponent: Controls the spread and intensity of the spotlight.
In our example application, we have a control panel that allows you to adjust these properties interactively. Let’s explore how this control panel is implemented.
import javafx.beans.property.DoubleProperty;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Node;
import javafx.scene.control.ColorPicker;
import javafx.scene.control.Label;
import javafx.scene.control.Slider;
import javafx.scene.effect.Light;
import javafx.scene.effect.Lighting;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
public class LightingControlPanel extends VBox {
/**
* Constructor for the LightingControlPanel.
*
* @param lighting The Lighting object to configure.
* @param spotLight The SpotLight object to configure.
*/
public LightingControlPanel(Lighting lighting, Light.Spot spotLight) {
super(15);
// Create a label for lighting properties
Label lightingPropertiesHeader = new Label("Lighting Properties");
lightingPropertiesHeader.setFont(new Font(18.0));
// Create a VBox for lighting properties with sliders
VBox lightingProperties = new VBox(
5,
lightingPropertiesHeader,
createLabeledBoundSlider(lighting.diffuseConstantProperty(), "Diffuse Constant", 2.0, 1.0),
createLabeledBoundSlider(lighting.specularConstantProperty(), "Specular Constant", 2.0, 0.3),
createLabeledBoundSlider(lighting.specularExponentProperty(), "Specular Exponent", 40.0, 20.0),
createLabeledBoundSlider(lighting.surfaceScaleProperty(), "Surface Scale", 10.0, 1.5)
);
// Create a label for spotlight properties
Label spotLightPropertiesHeader = new Label("Light.Spot Properties");
spotLightPropertiesHeader.setFont(new Font(18.0));
// Create the ColorPicker, and set DARKGREY as the default color
ColorPicker colorPicker = new ColorPicker(Color.DARKGREY);
// Bind the Light.Spot colorProperty to the ColorPicker valueProperty
spotLight.colorProperty().bind(colorPicker.valueProperty());
// Create the HBox to arrange the Label, and the ColorPicker
HBox colorContainer = this.createLabeledNode( 40, colorPicker, "Color" );
// Create a VBox for spotlight properties with sliders
VBox spotLightProperties = new VBox(
5,
spotLightPropertiesHeader,
createLabeledBoundSlider(spotLight.xProperty(), "X", 200.0, 0.0),
createLabeledBoundSlider(spotLight.yProperty(), "Y", 200.0, 0.0),
createLabeledBoundSlider(spotLight.zProperty(), "Z", 200.0, 0.0),
createLabeledBoundSlider(spotLight.pointsAtXProperty(), "Points At X", 200.0, 0.0),
createLabeledBoundSlider(spotLight.pointsAtYProperty(), "Points At Y", 200.0, 0.0),
createLabeledBoundSlider(spotLight.pointsAtZProperty(), "Points At Z", 200.0, 0.0),
createLabeledBoundSlider(spotLight.specularExponentProperty(), "Specular Exponent", 4.0, 1.0),
colorContainer
);
// Add lighting and spotlight property sections to the main VBox
getChildren().addAll(
lightingProperties,
spotLightProperties
);
// Set alignment and padding for the control panel
setAlignment(Pos.CENTER_LEFT);
setPadding(new Insets(15));
}
/**
* Create an HBox with a labeled bound slider for a property.
*
* @param property The DoubleProperty to bind to the slider.
* @param label The label for the slider.
* @param max The maximum value for the slider.
* @param defaultValue The default value for the slider.
* @return An HBox containing the labeled slider and its value label.
*/
private HBox createLabeledBoundSlider(DoubleProperty property, String label, double max, double defaultValue) {
Slider slider = new Slider(0.0, max, defaultValue);
property.bind(slider.valueProperty());
Label value = new Label();
value.setMinWidth(50);
value.textProperty().bind(slider.valueProperty().asString("%.2f"));
Label lblLabel = new Label(String.format("%-20s", label));
lblLabel.setMinWidth(110);
lblLabel.setAlignment(Pos.CENTER_LEFT);
return new HBox(5, lblLabel, slider, value);
}
private HBox createLabeledNode(double spacing, Node node, String label) {
HBox container = new HBox(
spacing,
new Label(String.format("%-20s", label)),
node
);
container.setAlignment(Pos.CENTER_LEFT);
return container;
}
}
The LightingControlPanel class is a user interface component that allows users to adjust the properties of the spotlight effect. It contains sliders and color pickers to interactively change the properties of the Light.Spot effect. These changes are reflected in real-time on the displayed image.
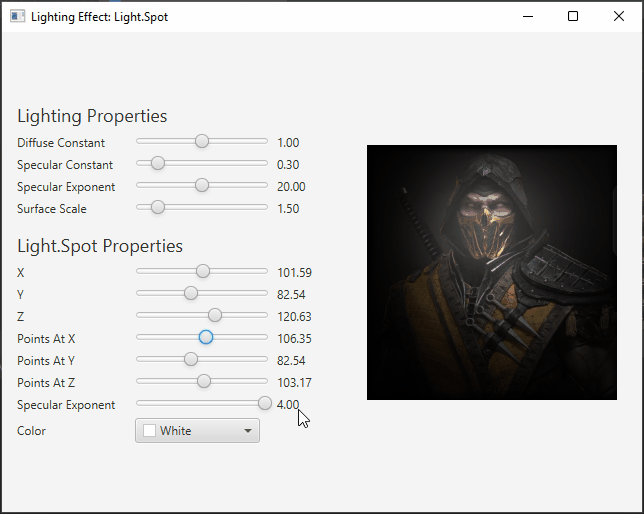
Conclusion
The Light.Spot class in JavaFX’s lighting effect system enables you to create spotlight effects that add depth and drama to your graphical user interfaces. Customizing its properties and integrating it with your JavaFX applications, you can create visually engaging and interactive user experiences.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.