How to add a stage icon to a JavaFX application? A stage icon is a small image that appears in the title bar of a JavaFX window as well as the taskbar, and it can help to give your application a professional and polished look. We will use JavaFX’s Image class and the Stage class’s getIcons() method to add an icon to our application as demonstrated in the code below:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Main extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
@Override
public void start(Stage stage) {
BorderPane layoutManager = new BorderPane();
Scene scene = new Scene(layoutManager, WIDTH, HEIGHT);
Image icon = new Image("duck.png");
/* set stage icon */
stage.getIcons().add(icon);
stage.setTitle("Adding an Icon to Your JavaFX Stage");
stage.setScene(scene);
stage.centerOnScreen();
stage.show();
}
}
When above code is executed, it creates a window with an icon in the top left corner as depicted in the image down below:
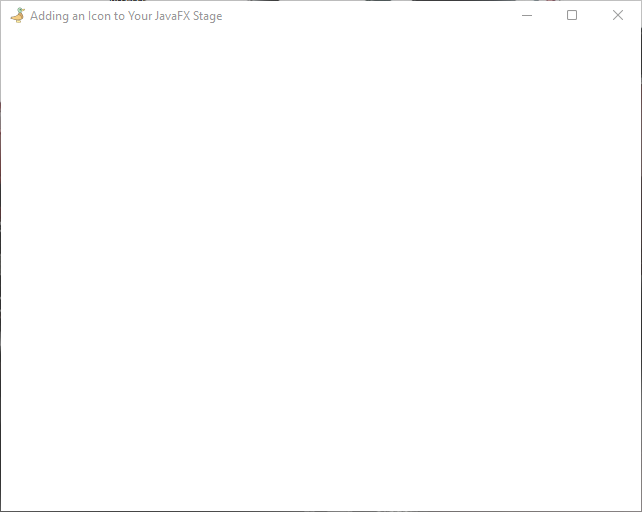
I hope it’s been informative to you. If you wish to learn more about JavaFX, please subscribe to our newsletter today and continue your JavaFX learning journey with us!