JavaFX is a versatile framework for building graphical user interfaces (GUIs) in Java applications. Among its many components, the FileChooser class stands out as a powerful tool for integrating file selection and handling functionality into your JavaFX applications. In this article, we’ll dive into the details of the JavaFX FileChooser, its features, and provide you with comprehensive code examples to get you started.
Understanding the FileChooser Class
The FileChooser class in JavaFX is used to create a standard file dialog that allows users to browse, select, and potentially open or save files. It provides a user-friendly way to interact with the file system without needing to implement all the file handling logic from scratch.
Creating and Using the FileChooser
Let’s start with a simple example of using the FileChooser class to open a file for reading:
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import java.io.File;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Button button = new Button("Open");
Label label = new Label("Selected File: N/A");
button.setOnAction(actionEvent -> openFile(actionEvent, label));
// Create the VBox container
// Add the Button and the Label to the VBox container
VBox container= new VBox(15, button, label);
container.setAlignment(Pos.CENTER);
// Add the VBox container to the BorderPane layout manager
this.parent.setCenter(container);
}
private void openFile(ActionEvent actionEvent, Label label) {
Stage stage = (Stage) ((Button) actionEvent.getSource()).getScene().getWindow();
// Create the file chooser
FileChooser chooser = new FileChooser();
// Set title for the file dialog
chooser.setTitle("Open File");
// Show the file dialog and get the selected file
File selectedFile = chooser.showOpenDialog(stage);
if (selectedFile != null) {
// Process the selected file
label.setText("Selected File: " + selectedFile.getAbsolutePath());
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FileChooser");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, a FileChooser object is created in the open Button handler, and its title is set. The showOpenDialog() method is then called to display the file chooser dialog. If the user selects a file, its absolute path is displayed in the label.
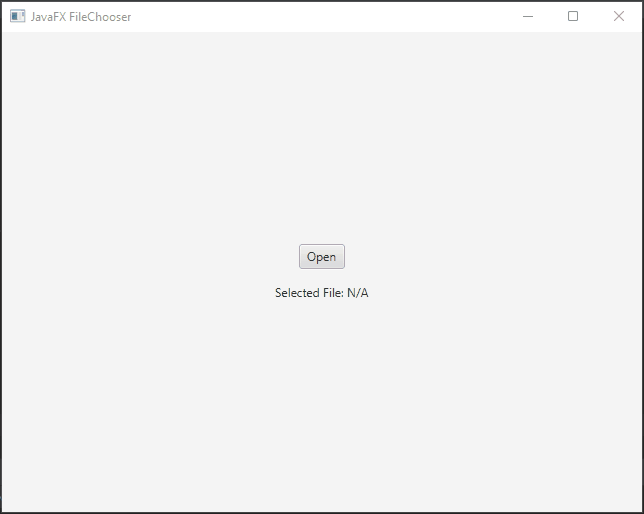
Configuring FileChooser Options
The FileChooser class provides various methods to customize its behavior and appearance. Here are some commonly used options:
Setting Initial Directory
You can set the initial directory from which the file dialog opens:
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import java.io.File;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Button button = new Button("Open");
Label label = new Label("Selected File: N/A");
button.setOnAction(actionEvent -> openFile(actionEvent, label));
// Create the VBox container
// Add the Button and the Label to the VBox container
VBox container= new VBox(15, button, label);
container.setAlignment(Pos.CENTER);
// Add the VBox container to the BorderPane layout manager
this.parent.setCenter(container);
}
private void openFile(ActionEvent actionEvent, Label label) {
Stage stage = (Stage) ((Button) actionEvent.getSource()).getScene().getWindow();
// Create the file chooser
FileChooser chooser = new FileChooser();
// Set title for the file dialog
chooser.setTitle("Open File");
// Setting the initial directory
chooser.setInitialDirectory(new File("C:/Users/edwar/Desktop"));
// Show the file dialog and get the selected file
File selectedFile = chooser.showOpenDialog(stage);
if (selectedFile != null) {
// Process the selected file
label.setText("Selected File: " + selectedFile.getAbsolutePath());
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FileChooser");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Adding File Filters
You can specify the types of files that should be displayed using filters:
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import java.io.File;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Button button = new Button("Open");
Label label = new Label("Selected File: N/A");
button.setOnAction(actionEvent -> openFile(actionEvent, label));
// Create the VBox container
// Add the Button and the Label to the VBox container
VBox container= new VBox(15, button, label);
container.setAlignment(Pos.CENTER);
// Add the VBox container to the BorderPane layout manager
this.parent.setCenter(container);
}
private void openFile(ActionEvent actionEvent, Label label) {
Stage stage = (Stage) ((Button) actionEvent.getSource()).getScene().getWindow();
// Create the file chooser
FileChooser chooser = new FileChooser();
// Set title for the file dialog
chooser.setTitle("Open File");
// Setting the initial directory
chooser.setInitialDirectory(new File("C:/Users/edwar/Desktop"));
// Specifying the types of files to be displayed (All, Text Files, or Images)
FileChooser.ExtensionFilter allFilter = new FileChooser.ExtensionFilter("All Files (*.*)", "*.*");
FileChooser.ExtensionFilter txtFilter = new FileChooser.ExtensionFilter("Text Files (*.txt)", "*.txt");
FileChooser.ExtensionFilter imageFilter = new FileChooser.ExtensionFilter("Image Files", "*.jpg", "*.png", "*.gif");
// Set filters
chooser.getExtensionFilters().addAll(allFilter, txtFilter, imageFilter);
// Show the file dialog and get the selected file
File selectedFile = chooser.showOpenDialog(stage);
if (selectedFile != null) {
// Process the selected file
label.setText("Selected File: " + selectedFile.getAbsolutePath());
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FileChooser");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Multiple File Selection
You can also enable users to select multiple files at once using the showOpenMultipleDialog method:
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import java.io.File;
import java.util.List;
import java.util.stream.Collectors;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Button button = new Button("Open");
Label label = new Label("Selected Files: N/A");
button.setOnAction(actionEvent -> openFile(actionEvent, label));
// Create the VBox container
// Add the Button and the Label to the VBox container
VBox container= new VBox(15, button, label);
container.setAlignment(Pos.CENTER);
// Add the VBox container to the BorderPane layout manager
this.parent.setCenter(container);
}
private void openFile(ActionEvent actionEvent, Label label) {
Stage stage = (Stage) ((Button) actionEvent.getSource()).getScene().getWindow();
// Create the file chooser
FileChooser chooser = new FileChooser();
// Set title for the file dialog
chooser.setTitle("Open File");
// Setting the initial directory
chooser.setInitialDirectory(new File("C:/Users/edwar/Desktop"));
// Specifying the types of files to be displayed (All, Text Files, or Images)
FileChooser.ExtensionFilter allFilter = new FileChooser.ExtensionFilter("All Files (*.*)", "*.*");
FileChooser.ExtensionFilter txtFilter = new FileChooser.ExtensionFilter("Text Files (*.txt)", "*.txt");
FileChooser.ExtensionFilter imageFilter = new FileChooser.ExtensionFilter("Image Files", "*.jpg", "*.png", "*.gif");
// Set filters
chooser.getExtensionFilters().addAll(allFilter, txtFilter, imageFilter);
// Show the file dialog and get selected files
List<File> selectedFileList = chooser.showOpenMultipleDialog(stage);
if (selectedFileList != null && !selectedFileList.isEmpty()) {
// Convert the selected file list to a string
String selectedFiles = selectedFileList.stream()
.map(File::getAbsolutePath)
.collect(Collectors.joining("\n"));
// Process the selected files
label.setText("Selected Files: \n\n" + selectedFiles);
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FileChooser");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
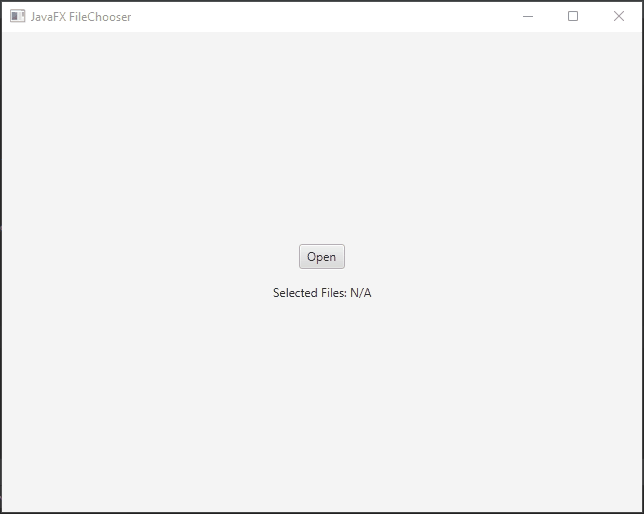
Save Dialog
The FileChooser can also be used to create save dialogs:
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import java.io.File;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Button button = new Button("Save");
Label label = new Label("Saved File: N/A");
button.setOnAction(actionEvent -> saveFile(actionEvent, label));
// Create the VBox container
// Add the Button and the Label to the VBox container
VBox container= new VBox(15, button, label);
container.setAlignment(Pos.CENTER);
// Add the VBox container to the BorderPane layout manager
this.parent.setCenter(container);
}
private void saveFile(ActionEvent actionEvent, Label label) {
Stage stage = (Stage) ((Button) actionEvent.getSource()).getScene().getWindow();
// Create the file chooser
FileChooser saver = new FileChooser();
// Set title for the file dialog
saver.setTitle("Save File");
// Specify the types of files to be saved (Text Files)
FileChooser.ExtensionFilter txtFilter = new FileChooser.ExtensionFilter("Text Files (*.txt)", "*.txt");
// Set filters
saver.getExtensionFilters().addAll(txtFilter);
// Set the initial file name
saver.setInitialFileName("new_document.txt");
File fileToSave = saver.showSaveDialog(stage);
if (fileToSave != null) {
// Save logic here
label.setText("Saved File: " + fileToSave .getAbsolutePath());
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FileChooser");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Conclusion
In this article, we’ve explored the FileChooser class in JavaFX, which provides a streamlined way to integrate file selection and handling capabilities into your applications. We’ve covered the basic usage, configuration options, and demonstrated how to create both open and save dialogs. With the FileChooser class at your disposal, you can enhance the user experience of your JavaFX applications by providing an intuitive and standardized file interaction mechanism.
Remember to refer to the official JavaFX documentation for more in-depth information and additional features available in the FileChooser class.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!