Modern user interfaces strive to provide users with intuitive and user-friendly features. One such feature is the clearable text field, which offers a convenient way for users to clear the entered text with a single click. In this article, we will explore how to create clearable text fields in JavaFX using ControlsFX, a library that extends the capabilities of JavaFX.
Introducing Clearable Text Fields
Clearable text fields are a common sight in many applications today. They come with a small “clear” button embedded within the text field, usually on the right-hand side. This button becomes visible when the user starts typing, allowing them to easily clear the entered text without having to manually select and delete the content.
JavaFX, a popular framework for building desktop applications, offers the TextField and PasswordField controls for text input. However, these controls do not include built-in support for clearable text fields. To address this limitation, we can leverage the ControlsFX library, which extends JavaFX with additional UI controls and enhancements.
Setting Up ControlsFX
Before we begin, make sure you have added the ControlsFX library to your JavaFX project. You can download the ControlsFX library from the official GitHub repository or include it as a dependency using a build tool like Maven or Gradle.
Creating a Clearable TextField
A clearable TextField is a TextField component that displays a clear button on the right-hand side of the input field. When the user enters text, this button allows them to easily clear the entered text with a single click. Here’s how you can create a clearable TextField using ControlsFX:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.textfield.TextFields;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create a ClearableTextField
TextField clearableTextField = TextFields.createClearableTextField();
// Set the TextField width to 200px
clearableTextField.setMaxWidth(200);
// Add the ClearableTextField to the center of the BorderPane
this.parent.setCenter(clearableTextField);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Clearable TextField in JavaFX using ControlsFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we use the TextFields.createClearableTextField() method to create a clearable TextField.
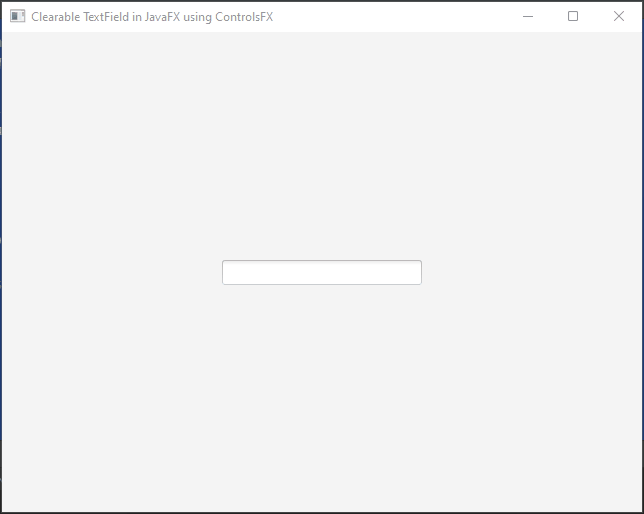
Creating a Clearable PasswordField
Similarly, a clearable PasswordField is a PasswordField component with a clear button that appears when the user enters text. This button allows users to quickly clear the entered password. Here’s how you can create a clearable PasswordField using ControlsFX:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.PasswordField;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.textfield.TextFields;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create a ClearablePasswordField
PasswordField clearablePasswordField = TextFields.createClearablePasswordField();
// Set the PasswordField width to 200px
clearablePasswordField.setMaxWidth(200);
// Add the ClearablePasswordField to the center of the BorderPane
this.parent.setCenter(clearablePasswordField);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Clearable TextField in JavaFX using ControlsFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we use the TextFields.createClearablePasswordField() method to create a clearable PasswordField.
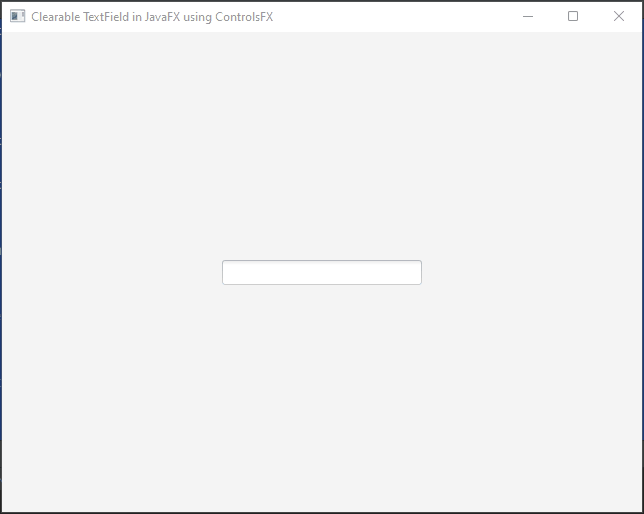
Conclusion
Adding clearable text fields to your JavaFX application can significantly enhance the user experience by providing a convenient way to clear input with minimal effort. ControlsFX simplifies this process by offering dedicated methods for creating clearable text fields. By incorporating such user-friendly features, you can create more intuitive and engaging applications that cater to your users’ needs. Remember to check the ControlsFX TextFields documentation for more.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!