Incorporating background images can significantly enhance the visual appeal of your JavaFX application. Whether you want to set a captivating backdrop for your entire scene or add an image to a specific container, JavaFX provides the flexibility to accomplish this task.
Adding Background Images
The provided code snippet showcases the utilization of JavaFX’s VBox and BorderPane to arrange and position nodes within a graphical user interface. Additionally, it exemplifies how to set a background image for a specific region.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.image.Image;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private Scene scene;
private VTextField name;
private VTextField email;
private VTextField password;
private VTextField confirmPassword;
private CheckBox agreeToTerms;
private Button register;
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// create the main content pane
VBox mainContent = new VBox(15);
// set main content pane background image
setRegionBackgroundImage(mainContent, "image.jpg");
mainContent.setAlignment(Pos.CENTER);
// create input fields
this.name = new VTextField("Name");
this.name.setMaxWidth(300.0);
this.email = new VTextField("Email");
this.email.setMaxWidth(300.0);
this.password = new VTextField("Password", true);
this.password.setMaxWidth(300.0);
this.confirmPassword = new VTextField("Confirm Password", true);
this.confirmPassword.setMaxWidth(300.0);
// create checkbox for terms and conditions
this.agreeToTerms = new CheckBox("I agree to the terms and conditions.");
// create register button
this.register = new Button("Register");
// create container for checkbox and register button
VBox buttonContainer = new VBox(15, this.agreeToTerms, this.register);
buttonContainer.setMaxWidth(300.0);
// add all elements to the main content pane
mainContent.getChildren().addAll(
this.name,
this.email,
this.password,
this.confirmPassword,
buttonContainer
);
// create the layout manager
BorderPane layoutManager = new BorderPane(mainContent);
// create the scene with specified dimensions
this.scene = new Scene(layoutManager, 640.0,480.0);
}
private void setRegionBackgroundImage(Region region, String imageUrl) {
// load the background image
Image image = new Image(imageUrl);
// create the background image with specified properties
BackgroundImage backgroundImage = new BackgroundImage(
image,
BackgroundRepeat.NO_REPEAT,
BackgroundRepeat.NO_REPEAT,
BackgroundPosition.DEFAULT,
BackgroundSize.DEFAULT
);
// apply the background image to the region
Background background = new Background(backgroundImage);
region.setBackground(background);
}
@Override
public void start(Stage stage) throws Exception {
stage.setScene(this.scene);
stage.setTitle("Creating a Stylish User Registration Form with Background Image in JavaFX");
stage.centerOnScreen();
stage.show();
}
static class VTextField extends VBox {
private final TextField textField;
public VTextField(String label) {
this(label, false);
}
public VTextField(String label, boolean obscure) {
if(obscure)
this.textField = new PasswordField();
else
this.textField = new TextField();
this.getChildren().addAll(new Label(label), this.textField);
}
public String getText() {
return this.textField.getText();
}
}
}
When executed, the above program generates a visually appealing registration form, as depicted in the image below:
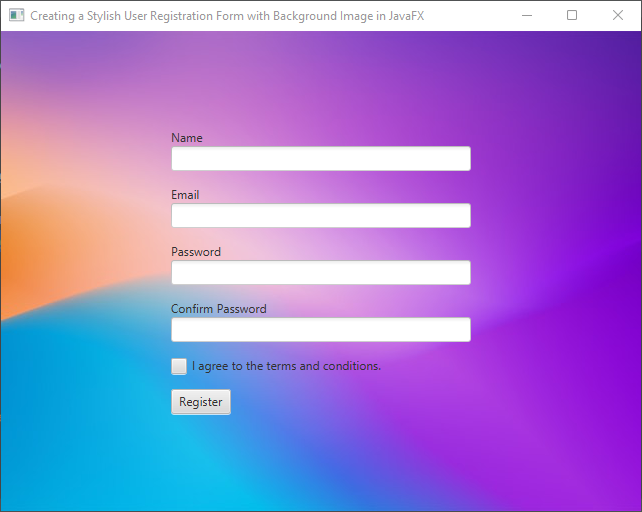
I hope you found this code informative and helpful in enhancing your JavaFX application. If you like this and would like to see more like it, make sure to subscribe to stay updated with my latest code snippets. 😊