JavaFX offers a powerful Media Player API that allows developers to create fully functional video players with ease. Whether you want to build a simple video playback application or create a more complex media player with custom controls and features, JavaFX provides the necessary tools and functionality. In this blog post, we will explore how to use the Media Player API in JavaFX to create a video player.
Creating a Video Player
The code defines a simple JavaFX application that creates a video player. If you have any questions or comments, please feel free to leave them below.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
import java.nio.file.Paths;
public class Main extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
@Override
public void start(Stage stage) {
BorderPane layoutManager = new BorderPane();
Scene scene = new Scene(layoutManager, WIDTH, HEIGHT);
MediaFileView mediaView = new MediaFileView("video.mp4");
// add media view to the center of the BorderPane
layoutManager.setCenter(mediaView);
layoutManager.setBottom(new MediaPlayerControls(mediaView.getMediaPlayer()));
stage.setTitle("Creating a Video Player with JavaFX");
stage.setScene(scene);
stage.centerOnScreen();
stage.show();
}
private static class MediaFileView extends MediaView {
public MediaFileView(String path) {
super();
this.init(path, false, 0.2);
}
public MediaFileView(String path, boolean autoplay, double volume) {
super();
this.init(path, autoplay, volume);
}
private void init(String path, boolean autoplay, double volume) {
Media media = new Media(Paths.get(path).toUri().toString());
MediaPlayer mediaPlayer = new MediaPlayer(media);
mediaPlayer.setAutoPlay(autoplay);
mediaPlayer.setVolume(volume);
setMediaPlayer(mediaPlayer);
}
}
}
The MediaPlayerControls class represents a set of media player controls for a MediaPlayer object. It extends HBox, allowing its child nodes to be displayed in a horizontal row. This class is defined in the following code:
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.control.Button;
import javafx.scene.layout.HBox;
import javafx.scene.media.MediaPlayer;
public class MediaPlayerControls extends HBox {
private final MediaPlayer mediaPlayer;
public MediaPlayerControls(MediaPlayer mediaPlayer) {
super(2);
this.mediaPlayer = mediaPlayer;
setAlignment(Pos.CENTER);
setPadding(new Insets(8));
getChildren().addAll(
new MediaPlayerButton("PLAY", MediaPlayerControls.this::play),
new MediaPlayerButton("PAUSE", MediaPlayerControls.this::pause),
new MediaPlayerButton("STOP", MediaPlayerControls.this::stop)
);
}
protected void play(ActionEvent actionEvent) {
this.mediaPlayer.play();
}
protected void pause(ActionEvent actionEvent) {
this.mediaPlayer.pause();
}
protected void stop(ActionEvent actionEvent) {
this.mediaPlayer.stop();
}
private static class MediaPlayerButton extends Button {
public MediaPlayerButton(String label, EventHandler<ActionEvent> handler) {
super(label);
setOnAction(handler);
}
}
}
When executed, the program above produces a video player, as depicted in the image below:
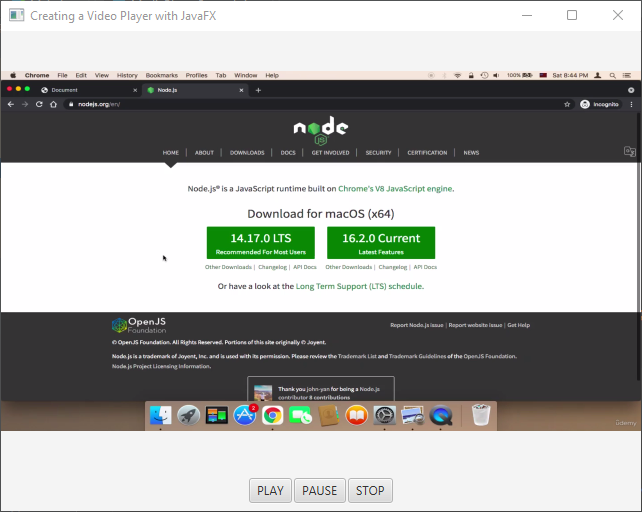
I sincerely hope that you find this code helpful. If you wish to learn more about JavaFX, please subscribe to our newsletter today and continue your JavaFX learning journey with us!