CSS selectors allow designers to target and style specific elements within an HTML document. If you’ve ever wondered how websites achieve their unique and visually appealing designs, CSS selectors are a fundamental part of the answer. A CSS selector is a pattern that defines which elements in an HTML document should be styled. In this article, we’ll explore the basics of CSS selectors, and provide examples to help you grasp the concept.
What are CSS Selectors?
CSS selectors are patterns used to select and style HTML elements. Imagine a selector as a set of instructions that tells the browser which elements on the page should receive a particular style. These styles can include properties like color, font size, margin, and more. By using selectors, developers can apply styles to specific elements or groups of elements, providing a level of control and customization that enhances the overall design and user experience.
Universal Selector
The universal selector, denoted by an asterisk (*), selects all elements on a webpage. It’s a broad approach that can be useful in certain situations.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Document Title -->
<title>CSS | Selectors</title>
<style>
* {
margin: 0;
padding: 0;
}
</style>
</head>
<body>
<h1>CSS | Selectors</h1>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
</body>
</html>
In this example, the universal selector is used to remove default margin and padding from all elements.
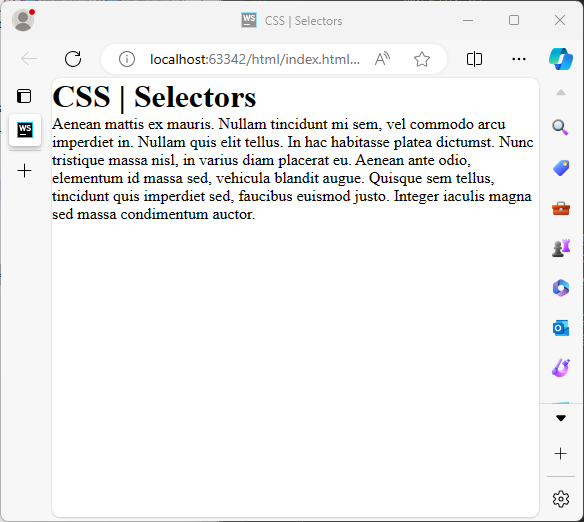
Element Selector
The most straightforward selector targets HTML elements directly. For example, to style all paragraphs on a page, you would use the paragraph selector like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Document Title -->
<title>CSS | Selectors</title>
<style>
p {
color: blue;
font-size: 16px;
}
</style>
</head>
<body>
<h1>CSS | Selectors</h1>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
</body>
</html>
In this example, the p selector targets all the paragraph elements in the HTML document, setting their text color to blue and font size to 16 pixels.
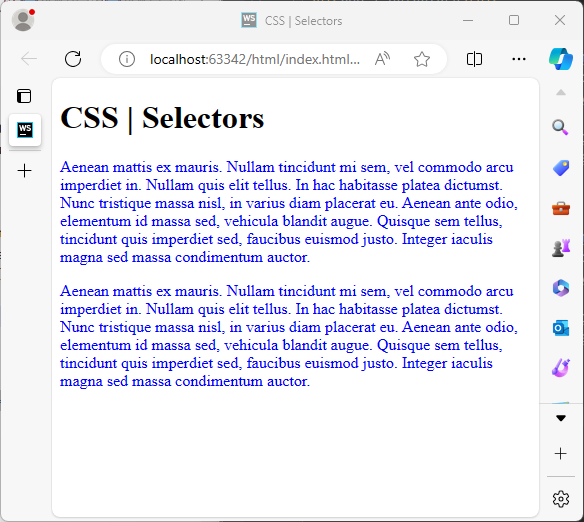
Class Selector
Classes provide a way to group elements together. You can apply the same style to multiple elements by assigning them the same class. Here’s an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Document Title -->
<title>CSS | Selectors</title>
<style>
.highlight {
background-color: yellow;
border: 2px solid orange;
}
</style>
</head>
<body>
<h1>CSS | Selectors</h1>
<p class="highlight">
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
<p class="highlight">
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
</body>
</html>
This class selector styles elements with the class “highlight,” creating a consistent element appearance.
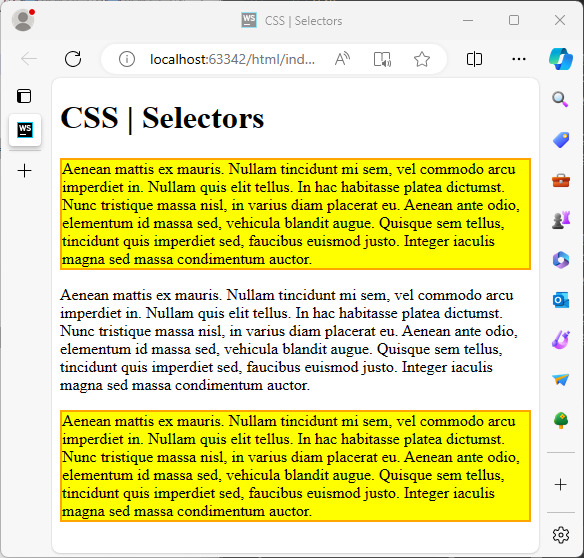
ID Selector
IDs are similar to classes but are intended for unique elements on a page. An element can have only one ID, and it must be unique. Here’s how you can apply styles to an element with a specific ID:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Document Title -->
<title>CSS | Selectors</title>
<style>
#main-title {
color: green;
font-size: 24px;
}
</style>
</head>
<body>
<h1 id="main-title">CSS | Selectors</h1>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
</body>
</html>
In this example, the style is applied to the element with the ID of “main-title.”
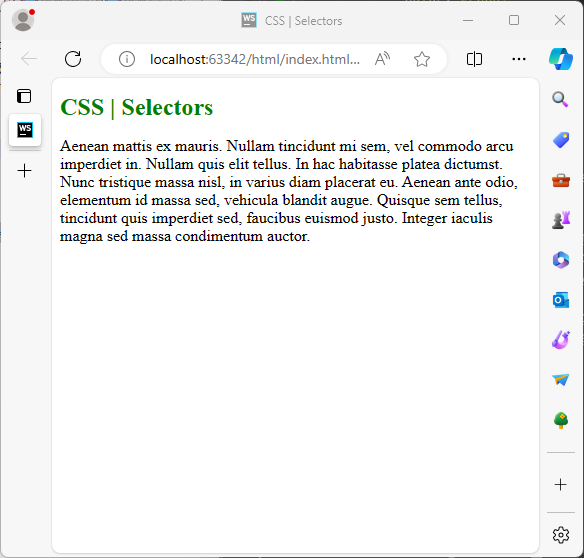
Grouping Selectors
Consider a scenario where you want to apply the same style to multiple elements. Instead of writing separate rules for each element, grouping selectors allows you to combine them into a single rule.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Document Title -->
<title>CSS | Selectors</title>
<style>
h1, h2, h3 {
color: #3498db;
font-family: 'Arial', sans-serif;
}
</style>
</head>
<body>
<h1>CSS | Selectors</h1>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
<h2>Styling Multiple Elements</h2>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
<h3>Conclusion</h3>
<p>
Aenean mattis ex mauris. Nullam tincidunt mi sem, vel commodo arcu imperdiet in.
Nullam quis elit tellus. In hac habitasse platea dictumst.
Nunc tristique massa nisl, in varius diam placerat eu.
Aenean ante odio, elementum id massa sed, vehicula blandit augue.
Quisque sem tellus, tincidunt quis imperdiet sed, faucibus euismod justo.
Integer iaculis magna sed massa condimentum auctor.
</p>
</body>
</html>
In this example, the styles inside the curly braces are applied to all <h1>, <h2>, and <h3> elements; it could be any selector other than those discussed here. The comma separates each selector, indicating that the same styles should be applied to each of them.
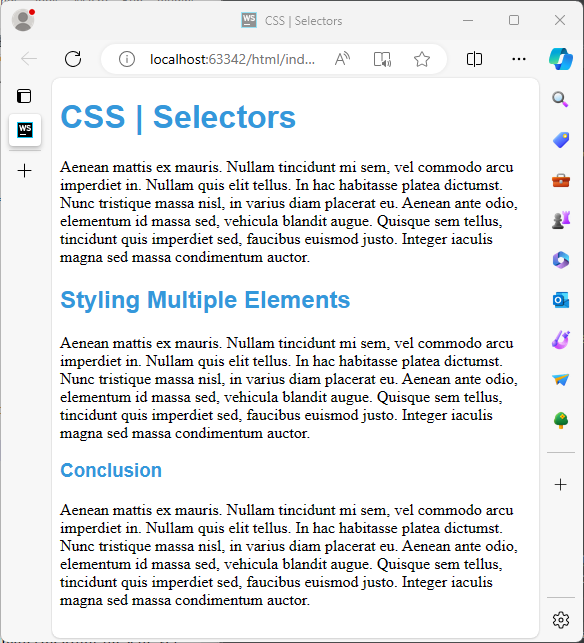
This approach not only saves you from duplicating code but also makes it easier to maintain and update styles. If you decide to change the font or color, you can do it in one place rather than updating each individual selector.
Conclusion
In conclusion, CSS selectors are indispensable tools for web developers seeking to create well-designed and visually appealing websites. Understanding of various types of selectors allows you to precisely target and style specific elements, making your web development journey more efficient and enjoyable. For more content, please subscribe to our newsletter.