This code example guarantees a secure JavaFX application close with confirmation dialogs. When a user tries to close the program, the code registers an event handler to catch it. An alert dialog is presented to ask the user if they want to save their changes before closing the program when the close request event is detected. The close request event is consumed and the program stays open if the user clicks the cancel button. If not, the application is closed and the close request event is not consumed.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.ButtonType;
import javafx.scene.image.Image;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
import javafx.stage.WindowEvent;
public class Main extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
@Override
public void start(Stage stage) {
BorderPane layoutManager = new BorderPane();
Scene scene = new Scene(layoutManager, WIDTH, HEIGHT);
Image icon = new Image("icon.png");
/* set stage icon */
stage.getIcons().add(icon);
stage.setOnCloseRequest(new CloseRequestHandler());
stage.setTitle("Ensuring Safe Program Close in JavaFX with Confirmation Dialogs");
stage.setScene(scene);
stage.centerOnScreen();
stage.show();
}
private static class CloseRequestHandler implements javafx.event.EventHandler<WindowEvent> {
@Override
public void handle(WindowEvent event) {
Alert alert = new Alert(Alert.AlertType.CONFIRMATION);
alert.titleProperty().setValue("Close Program");
alert.headerTextProperty().setValue("Do you want to save your changes before closing the program?");
alert.showAndWait().ifPresent(buttonType -> {
if (buttonType.equals(ButtonType.CANCEL)) {
event.consume();
}
});
}
}
}
When the program above is executed, a simple window is created. However, when the user attempts to close it, the confirmation dialog shown in the image below appears, prompting the user if they are sure they want to close the program.
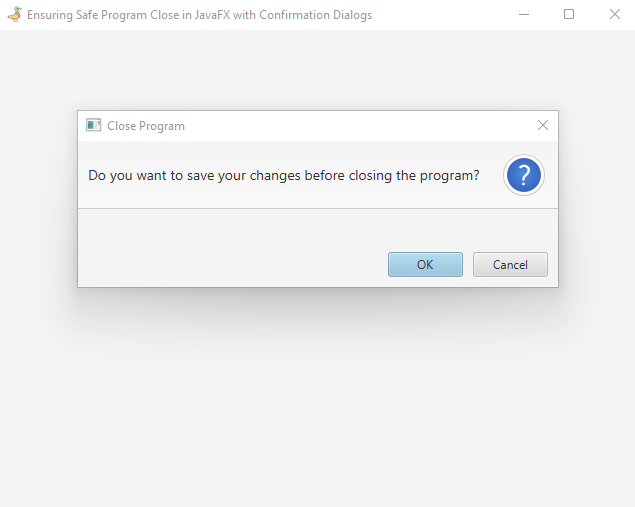
With this method, the user is always reminded to save their work before closing the program, avoiding the possible loss of previously unsaved information. I do sincerely hope you find this informative, and use it in your programs. If you wish to learn more about JavaFX, please subscribe to our newsletter today and continue your JavaFX learning journey with us!