In modern user interface (UI) design, providing a seamless and organized user experience is of paramount importance. One way to achieve this is by incorporating collapsible sections within your UI. JavaFX, a versatile framework for building desktop and rich internet applications, offers the Accordion control that enables developers to create such collapsible UI sections with ease. In this article, we will explore the JavaFX Accordion control and learn how to use it to create visually appealing collapsible sections within your application.
Understanding the Accordion Control
The Accordion is a user interface control that consists of a series of titled panes stacked vertically. Each titled pane represents a collapsible section that can be expanded or collapsed with a click. This component is particularly useful when you want to present a collection of related content or options in a compact and organized manner. It provides a clear visual hierarchy and allows users to focus on the content they’re interested in while keeping the rest hidden.
Creating a Basic Accordion
Let’s start by creating a simple JavaFX application with an Accordion containing a few TitledPanes. This will give you a hands-on experience of setting up collapsible sections in your user interface.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Accordion;
import javafx.scene.control.TitledPane;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an Accordion
Accordion accordion = new Accordion();
// Create TitledPanes with content
TitledPane pane1 = new TitledPane("Section 1", new VBox());
TitledPane pane2 = new TitledPane("Section 2", new VBox());
TitledPane pane3 = new TitledPane("Section 3", new VBox());
// Add TitledPanes to the Accordion
accordion.getPanes().addAll(pane1, pane2, pane3);
// Add the Accordion to the BorderPane
this.parent.setCenter(accordion);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Accordion: Creating Collapsible UI Sections");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we create an Accordion object and add three TitledPane instances to it. Each TitledPane represents a collapsible section with a title and an empty VBox as content. You can replace the content with any JavaFX nodes you need for your application.
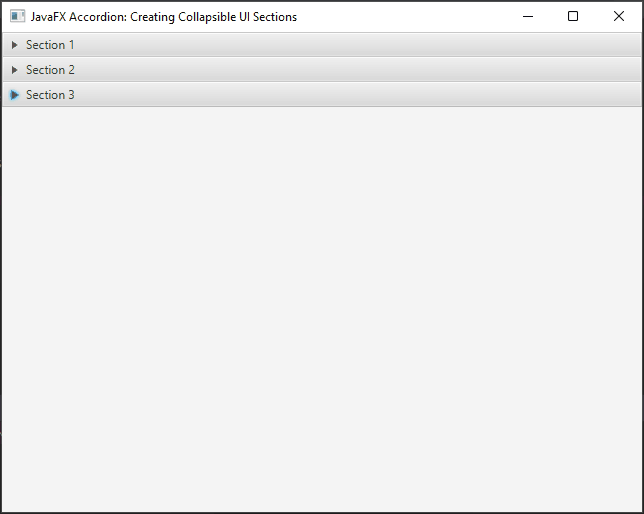
Adding Content to Collapsible Sections
While the above example demonstrates the basic structure of an Accordion, the real power comes from adding meaningful content to the collapsible sections. Let’s enhance our application by adding some content to the sections.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an Accordion
Accordion accordion = new Accordion();
// Create TitledPanes with content
for(int i = 1; i <= 3; ++i) {
// Create the TitlePane content
VBox content = new VBox(
new VBox(10, // Space between Nodes
new Label("Content for Section %d".formatted(i)),
new Button("Button %d".formatted(i)),
new CheckBox("Check Box %d".formatted(i))
)
);
content.setMaxWidth(200.0);
content.setAlignment(Pos.CENTER);
// Create the TitlePane with content
TitledPane pane = new TitledPane("Section %d".formatted(i), content);
// Add TitledPane to the Accordion
accordion.getPanes().addAll(pane);
}
// Add the Accordion to the BorderPane
this.parent.setCenter(accordion);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Accordion: Creating Collapsible UI Sections");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we’ve created three (3) VBox containers, each containing a label, a button, and a checkbox. These containers hold the content for the respective collapsible sections. By setting the content of each TitledPane using the setContent() method, we associate the content with the sections.
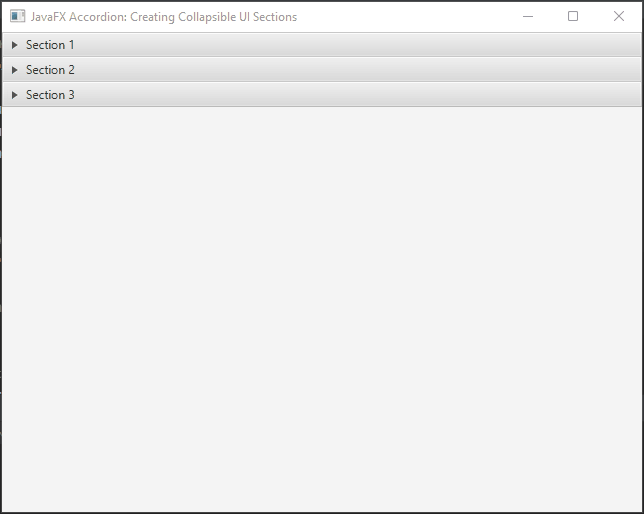
Setting the Initially Expanded Pane
To determine which pane is expanded when the Accordion is first displayed, you can use the setExpandedPane method. This method accepts a TitledPane instance as an argument and makes that pane the initially expanded one. Here’s how you can implement this:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an Accordion
Accordion accordion = new Accordion();
// Create TitledPanes with content
for(int i = 1; i <= 3; ++i) {
// Create the TitlePane content
VBox content = new VBox(
new VBox(10, // Space between Nodes
new Label("Content for Section %d".formatted(i)),
new Button("Button %d".formatted(i)),
new CheckBox("Check Box %d".formatted(i))
)
);
content.setMaxWidth(200.0);
content.setAlignment(Pos.CENTER);
// Create the TitlePane with content
TitledPane pane = new TitledPane("Section %d".formatted(i), content);
if(i == 2) {
// Set the pane 2 the initially expanded pane
accordion.setExpandedPane(pane);
}
// Add TitledPane to the Accordion
accordion.getPanes().addAll(pane);
}
// Add the Accordion to the BorderPane
this.parent.setCenter(accordion);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Accordion: Creating Collapsible UI Sections");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, the second pane (pane2) is set as the initially expanded pane using the setExpandedPane method. When the application runs, “Section 2” will be open by default, while the other sections remain collapsed.
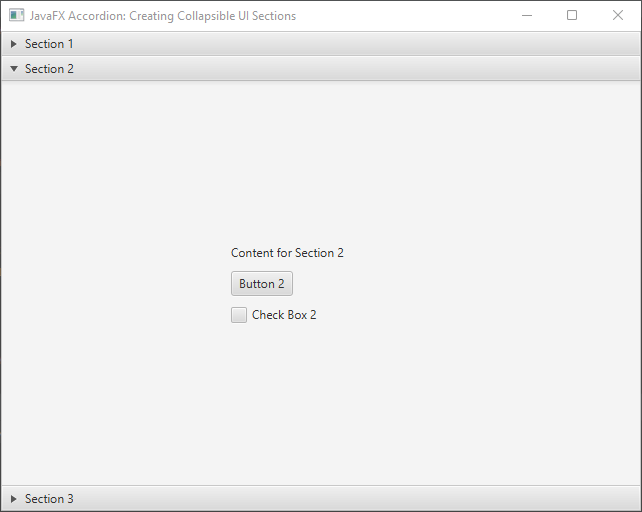
User Interaction and Dynamic Pane Expansion
Once the application is running, users can interact with the Accordion to expand or collapse sections as needed. The Accordion automatically handles the opening and closing animations for a smooth user experience.
You can also implement event listeners to respond to user actions. For example, you can attach a listener to the expandedPaneProperty to perform actions when a pane is expanded or collapsed:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an Accordion
Accordion accordion = new Accordion();
// Create TitledPanes with content
for(int i = 1; i <= 3; ++i) {
// Create the TitlePane content
VBox content = new VBox(
new VBox(10, // Space between Nodes
new Label("Content for Section %d".formatted(i)),
new Button("Button %d".formatted(i)),
new CheckBox("Check Box %d".formatted(i))
)
);
content.setMaxWidth(200.0);
content.setAlignment(Pos.CENTER);
// Create the TitlePane with content
TitledPane pane = new TitledPane("Section %d".formatted(i), content);
// Add TitledPane to the Accordion
accordion.getPanes().addAll(pane);
}
accordion.expandedPaneProperty().addListener((observable, oldPane, newPane) -> {
if (newPane != null) {
System.out.println("Expanded: " + newPane.getText());
}
if(oldPane != null) {
System.out.println("Collapsed: " + oldPane.getText());
}
});
// Add the Accordion to the BorderPane
this.parent.setCenter(accordion);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX Accordion: Creating Collapsible UI Sections");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Conclusion
The JavaFX Accordion component provides a user-friendly way to organize and present content in collapsible sections, enhancing the overall user experience of your Java applications. By creating TitledPanes within an Accordion, developers can easily design interfaces that provide a structured layout while allowing users to focus on the information they need. With the ability to customize the appearance and behavior of the Accordion, it becomes a powerful tool for creating dynamic and interactive UIs.
Sources:
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!