JavaFX, a versatile and robust UI framework, has gained popularity among developers for creating cross-platform applications with stunning graphical interfaces. One of the libraries that complements JavaFX is ControlsFX, which provides additional controls and utilities to enhance the user experience. In this article, we’ll delve into the ControlsFX WorldMapView control, a powerful tool for incorporating interactive world maps into your JavaFX applications.
What’s ControlsFX WorldMapView?
The WorldMapView control from ControlsFX is designed to make geographical data visualization easier and more engaging. It allows developers to display world maps and plot data points on the map, providing a visually appealing way to showcase location-based information. Whether you’re building a weather app, a travel planning tool, or any application requiring geographic representation, the WorldMapView can be a valuable addition to your toolkit.
Using the WorldMapView Control
To get started with the WorldMapView control, you’ll need to add the ControlsFX library to your project. You can download the library from the ControlsFX GitHub repository, or include it using a build tool like Maven or Gradle.
Here’s an example of how to create a simple JavaFX application that utilizes the WorldMapView control:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we’ve created a simple JavaFX application that displays an instance of the WorldMapView control in a BorderPane layout container.
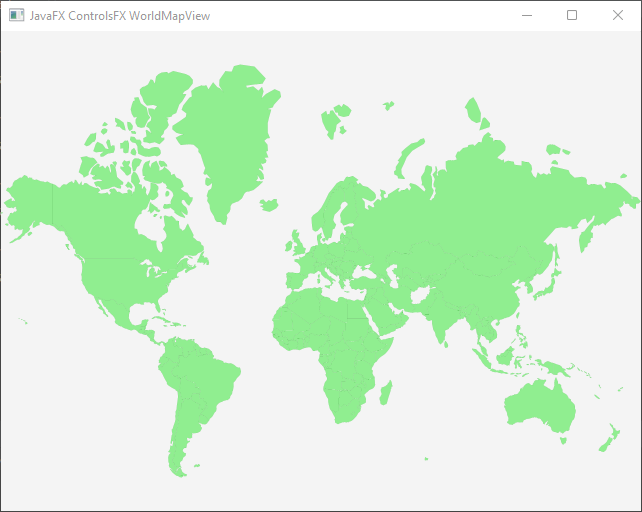
Customizing Displayed Countries
The setCountries method in the WorldMapView control allows you to define a specific list of countries that will be displayed on the map. This feature is particularly useful when you want to focus the user’s attention on a specific set of countries, regions, or territories. By customizing the list of displayed countries, you can create more focused and targeted visualizations within your JavaFX application.
Here’s an example of how you can use the setCountries method to display a selected set of countries on the map:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Create a list of countries to be displayed
ObservableList<WorldMapView.Country> selectedCountries = FXCollections.observableArrayList(
WorldMapView.Country.ZM, // Zambia
WorldMapView.Country.ZW, // Zimbabwe
WorldMapView.Country.MW, // Malawi
WorldMapView.Country.BW, // Botswana
WorldMapView.Country.NA, // Namibia
WorldMapView.Country.TZ, // Tanzania
WorldMapView.Country.MZ, // Mozambique
WorldMapView.Country.AO, // Angola
WorldMapView.Country.CD // Congo
);
// Set the list of selected countries to be displayed on the map
worldMapView.setCountries(selectedCountries);
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we create a JavaFX application that displays a custom set of countries—Zambia (ZM), Zimbabwe (ZW), Malawi (MW), Botswana (BW), Namibia (NA), Tanzania (TZ), Mozambique (MZ), Angola (AO), and Congo (CD)—on the map using the setCountries method. This allows you to narrow down the map’s content to a specific set of countries that are relevant to your application.
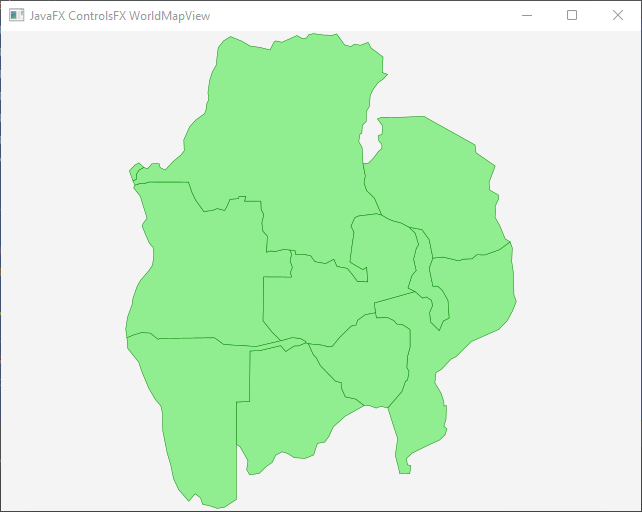
Selecting Countries Programmatically
The setSelectedCountries method in the ControlsFX WorldMapView class is used to set the list of currently selected countries on the map. This method allows you to programmatically select countries and visually highlight them on the map.
Here’s how you can use the setSelectedCountries method:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Set WorldMapView selection mode
worldMapView.setCountrySelectionMode(WorldMapView.SelectionMode.MULTIPLE);
// Create a list of selected countries
ObservableList<WorldMapView.Country> selectedCountries = FXCollections.observableArrayList(
WorldMapView.Country.ZM
);
// Set the list of selected countries on the map
worldMapView.setSelectedCountries(selectedCountries);
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, a list of Country objects is created and added to the WorldMapView using the setSelectedCountries method. Each Country object represents a selected country to be highlighted on the map.
Getting Selected Countries
The getSelectedCountries method in the ControlsFX WorldMapView class returns the list of currently selected countries from the selection model. This method allows you to retrieve the countries that are currently highlighted or selected on the map.
Here’s how you can use the getSelectedCountries method:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Set WorldMapView selection mode
worldMapView.setCountrySelectionMode(WorldMapView.SelectionMode.MULTIPLE);
// Create a list of selected countries
ObservableList<WorldMapView.Country> initialSelectedCountries = FXCollections.observableArrayList(
WorldMapView.Country.ZM
);
// Set the list of selected countries on the map
worldMapView.setSelectedCountries(initialSelectedCountries);
// Get the list of selected countries
ObservableList<WorldMapView.Country> selectedCountries = worldMapView.getSelectedCountries();
// Print selected countries
for (WorldMapView.Country country : selectedCountries) {
System.out.println("Selected Country: " + country.name());
}
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we used the getSelectedCountries method to retrieve the list of selected countries. The code then prints the names of the selected countries to the console.
Customizing the Country View Factory
The setCountryViewFactory method in the ControlsFX WorldMapView class allows you to set a custom factory for creating the visual representation of countries on the map. This factory is responsible for creating instances of the WorldMapView.CountryView class.
Here’s how you can use the setCountryViewFactory method:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.util.Callback;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Create a custom country view factory
Callback<WorldMapView.Country, WorldMapView.CountryView> countryViewFactory = country -> {
WorldMapView.CountryView customView = new WorldMapView.CountryView(country);
// Set a custom fill color
customView.setFill(Color.GREEN);
// Set a custom stroke color
customView.setStroke(Color.BLACK);
return customView;
};
// Set the custom country view factory
worldMapView.setCountryViewFactory(countryViewFactory);
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, a custom countryViewFactory is created using the Callback interface. The call method of the factory creates an instance of CountryView for each country and customizes its appearance by setting fill and stroke colors. This factory is then set using the setCountryViewFactory method.
Displaying Locations on the Map
The setShowLocations method is used in ControlsFX’s WorldMapView class to control the visibility of locations on the map. When you call this method with a true parameter, it indicates that locations should be shown on the map. Conversely, passing false as the parameter will hide the locations.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Set whether to show locations
worldMapView.setShowLocations(true);
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
By using the setShowLocations method, you can dynamically control whether the locations on the WorldMapView are displayed or hidden based on your application’s requirements.
Customizing Displayed Locations
The setLocations method in the ControlsFX WorldMapView class allows you to set a list of locations to be displayed on the map. Each location is represented by an instance of the WorldMapView.Location class.
Here’s how you can use the setLocations method to display a list of locations on the map:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Set whether to show locations
worldMapView.setShowLocations(true);
// Create a list of locations
ObservableList<WorldMapView.Location> locations = FXCollections.observableArrayList();
locations.add(new WorldMapView.Location("New York", 40.712776, -74.005974));
locations.add(new WorldMapView.Location("London", 51.5074, -0.1278));
locations.add(new WorldMapView.Location("Tokyo", 35.6895, 139.6917));
// Set the list of locations on the map
worldMapView.setLocations(locations);
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, the WorldMapView.Location instances are created with the name of the location and its latitude and longitude coordinates. The setLocations method then sets the provided list of locations on the map, and those locations will be displayed as markers on the map.
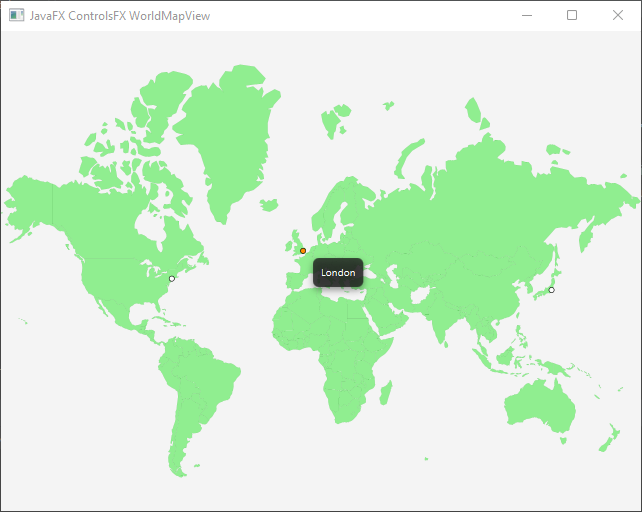
Selecting Locations Programmatically
The setSelectedLocations method in the ControlsFX WorldMapView class is used to set the list of currently selected locations on the map. This method allows you to programmatically select locations and visually highlight them on the map.
Here’s how you can use the setSelectedLocations method:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Set whether to show locations
worldMapView.setShowLocations(true);
// Create a list of locations
ObservableList<WorldMapView.Location> locations = FXCollections.observableArrayList();
locations.add(new WorldMapView.Location("New York", 40.712776, -74.005974));
locations.add(new WorldMapView.Location("London", 51.5074, -0.1278));
locations.add(new WorldMapView.Location("Tokyo", 35.6895, 139.6917));
// Set the list of selected locations on the map
worldMapView.setSelectedLocations(locations);
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, a list of Location objects is created and added to the WorldMapView using the setSelectedLocations method. Each Location object represents a selected location to be highlighted on the map.
Getting Selected Locations
The getSelectedLocations method in the ControlsFX WorldMapView class returns the list of currently selected locations from the selection model. This method allows you to retrieve the locations that are currently highlighted or selected on the map.
Here’s how you can use the getSelectedLocations method:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Set whether to show locations
worldMapView.setShowLocations(true);
// Create a list of locations
ObservableList<WorldMapView.Location> locations = FXCollections.observableArrayList();
locations.add(new WorldMapView.Location("New York", 40.712776, -74.005974));
locations.add(new WorldMapView.Location("London", 51.5074, -0.1278));
locations.add(new WorldMapView.Location("Tokyo", 35.6895, 139.6917));
// Set the list of selected locations on the map
worldMapView.setSelectedLocations(locations);
// Get the list of selected locations
ObservableList<WorldMapView.Location> selectedLocations = worldMapView.getSelectedLocations();
// Print selected locations
for (WorldMapView.Location location : selectedLocations) {
System.out.println("Selected Location: " + location.getName());
}
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we used the getSelectedLocations method to retrieve the list of selected locations. The code then prints the names of the selected locations to the console.
Customizing the Location View Factory
The setLocationViewFactory method in the ControlsFX WorldMapView class allows you to set a custom factory for creating the visual representation of locations on the map. This factory is responsible for creating instances of Node that represent the locations.
Here’s how you can use the setLocationViewFactory method:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Callback;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Set whether to show locations
worldMapView.setShowLocations(true);
// Create a list of locations
ObservableList<WorldMapView.Location> locations = FXCollections.observableArrayList();
locations.add(new WorldMapView.Location("New York", 40.712776, -74.005974));
locations.add(new WorldMapView.Location("London", 51.5074, -0.1278));
locations.add(new WorldMapView.Location("Tokyo", 35.6895, 139.6917));
// Set the list of locations on the map
worldMapView.setLocations(locations);
// Create a custom location view factory
Callback<WorldMapView.Location, Node> locationViewFactory = location -> new Circle(10, Color.RED);
// Set the custom location view factory
worldMapView.setLocationViewFactory(locationViewFactory);
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, a custom locationViewFactory is created using the Callback interface. The call method of the factory creates a custom Node (in this case, a red circle) to represent each location. This factory is then set using the setLocationViewFactory method.
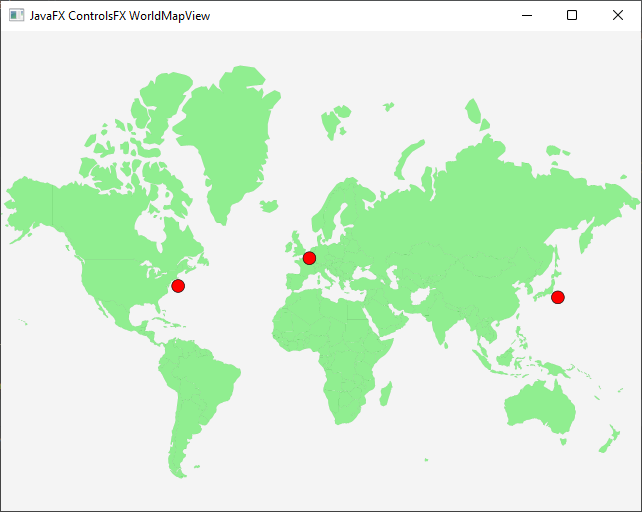
Adding Event Handling
To add event handling for changes to the selectedCountriesProperty() or selectedLocationsProperty() of a ControlsFX WorldMapView, you can use the JavaFX property’s addListener method. Here’s an example of how you can achieve this:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.WorldMapView;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create the WorldMapView
WorldMapView worldMapView = new WorldMapView();
// Set background color to transparent
worldMapView.setStyle("-fx-background-color: transparent;");
// Add a listener to the selected countries property
worldMapView.selectedCountriesProperty().addListener((observable, oldValue, newValue)
-> System.out.println("Selected countries changed: " + newValue));
// Add the WorldMapView to the BorderPane
this.parent.setCenter(worldMapView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX ControlsFX WorldMapView");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, a ChangeListener is added to the selectedCountriesProperty or the selectedLocationsProperty. The changed method of the listener will be invoked whenever the selected countries or locations change. You can access the new value of the selected countries or locations using the newValue parameter.
Conclusion
The WorldMapView control from the JavaFX ControlsFX library opens up new possibilities for creating interactive world maps within JavaFX applications. Whether you’re building a data visualization tool, a geographic information system, or any application requiring geographic representation, the WorldMapView control provides powerful features and customization options. By incorporating this control into your projects, you can enhance the user experience and deliver visually appealing and informative maps.
Remember to consult the official ControlsFX documentation for more detailed information about the WorldMapView control and its various options.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!