In modern-day software applications, security is of utmost importance. When it comes to handling sensitive information, such as user passwords, developers must ensure that the data is well-protected and hidden from prying eyes. JavaFX, a rich set of graphical user interface (GUI) tools, provides a secure way to handle password input with the PasswordField class. In this article, we will explore how to use JavaFX’s PasswordField to create a secure password input field in your Java applications.
What is JavaFX PasswordField?
The javafx.scene.control.PasswordField class in JavaFX is designed specifically to handle password input. Unlike a regular text field, the characters typed into a PasswordField are not displayed in clear text; instead, they are masked with bullets or asterisks, ensuring that the password remains hidden from view.
Creating a JavaFX PasswordField
Before we delve into the code examples, ensure that you have JavaFX properly set up in your development environment. Now, let’s create a simple JavaFX application containing a PasswordField and a button to retrieve the entered password.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create PasswordField
PasswordField passwordField = new PasswordField();
passwordField.setPromptText("Enter your password");
// Create Button to retrieve the password
Button submitButton = new Button("Submit");
submitButton.setOnAction(e -> {
String password = passwordField.getText();
// Print the password out to the console
System.out.println("Entered password: " + password);
});
// Create layout and add controls
VBox vbox = new VBox(10, passwordField, new VBox(submitButton));
vbox.setAlignment(Pos.CENTER);
vbox.setMaxWidth(400.0);
this.parent.setCenter(vbox);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX PasswordField: Secure Input Handling in Your Java Applications");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
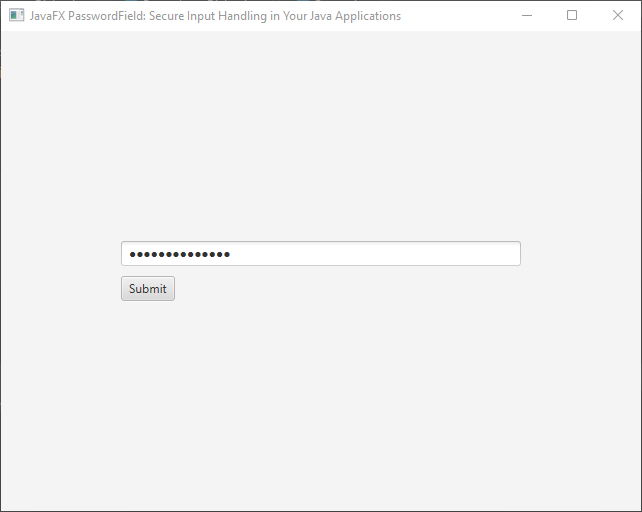
Customizing the PasswordField
The JavaFX PasswordField allows for various customizations to match the style and requirements of your application. Here are some common customizations you can apply:
Prompt Text
You can set a prompt text inside the PasswordField to provide a hint or description to the user about what is expected. This prompt text will be displayed when the PasswordField is empty.
// Set a prompt text
passwordField.setPromptText("Enter your password");
Max Length
To limit the maximum number of characters that a user can enter in the PasswordField, you can achieve it by adding an event filter to the TextField of the PasswordField and manually restricting the length of the input. Here’s how you can do it:
// Limit the maximum number of characters to 10
int maxLength = 10;
passwordField.textProperty().addListener((observable, oldValue, newValue) -> {
if (newValue.length() > maxLength) {
passwordField.setText(oldValue);
}
});
Event Handling
Besides the example above, you can handle events like pressing the Enter key to submit the password or any other custom events as needed. For instance, you can add an event handler to the PasswordField to process the password when the user presses the Enter key:
passwordField.setOnAction(e -> {
String password = passwordField.getText();
// Do something with the entered password
System.out.println("Password submitted: " + password);
});
Styling
Apply CSS styling to change the appearance of the PasswordField. You can modify the appearance of the masked characters, background, border, and more using CSS.
// Apply CSS style to the PasswordField
passwordField.setStyle("-fx-background-color: #f2f2f2; -fx-border-radius: 3px; -fx-border-color: rgba(0, 0, 0, 0.5); -fx-text-fill: #333333; -fx-font-size: 14px;");
Secure Password Handling in Java
When dealing with passwords or any sensitive information in Java, it is crucial to prioritize security and take measures to protect the data from potential threats. One of the essential aspects of secure password handling is ensuring that the password is not stored as plain text in memory, as plain text passwords can be easily compromised in case of a security breach. Instead, Java developers should use the char[] array to temporarily store passwords and take advantage of its mutability to erase the sensitive data when it is no longer needed. In this section, we will discuss best practices for secure password handling in Java.
Using char[] instead of String
In Java, strings are immutable, which means that once a string is created, its value cannot be changed. When a password is stored as a String, it remains in memory until the garbage collector removes it, making it susceptible to unauthorized access.
To mitigate this risk, use the char[] array to store the password temporarily. Unlike strings, arrays are mutable, allowing you to overwrite the password data explicitly, reducing the window of opportunity for potential attackers to retrieve the password from memory.
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import java.util.Arrays;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create PasswordField
PasswordField passwordField = new PasswordField();
passwordField.setPromptText("Enter your password");
// Create Button to retrieve the password
Button submitButton = new Button("Submit");
submitButton.setOnAction(e -> {
char[] passwordChars = passwordField.getText().toCharArray();
// Process the password securely here (e.g., validate, store, etc.)
System.out.println("Enter Password: " + Arrays.toString(passwordChars));
// Clear the password after processing
clearPasswordChars(passwordChars);
});
// Create layout and add controls
VBox vbox = new VBox(10, passwordField, new VBox(submitButton));
vbox.setAlignment(Pos.CENTER);
vbox.setMaxWidth(400.0);
this.parent.setCenter(vbox);
}
// Helper method to clear the password from memory
private void clearPasswordChars(char[] passwordChars) {
// Overwrite each character with null character
Arrays.fill(passwordChars, '\u0000');
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX PasswordField: Secure Input Handling in Your Java Applications");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Limiting Password Exposure
It’s essential to limit the exposure of passwords as much as possible. Avoid logging passwords or displaying them in error messages. Only request passwords when necessary, and once the password is no longer required, clear it from memory promptly.
Securely Hashing Passwords
When storing passwords in databases or other storage systems, it is critical to hash the passwords using strong and secure cryptographic hash functions (e.g., bcrypt, Argon2, or SHA-256). Hashing transforms the password into an irreversible string of characters, making it challenging for attackers to retrieve the original password even if they gain access to the stored hash.
Conclusion
In this article, we explored how to use the JavaFX PasswordField to create a secure password input field in your Java applications. By masking the characters, the PasswordField ensures that sensitive information, like user passwords, remains hidden from view. Remember that while JavaFX’s PasswordField helps with the presentation of passwords, it is crucial to implement secure password handling techniques when storing or transmitting sensitive information. As developers, safeguarding user data should always be a top priority.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!