JavaFX is a powerful framework for creating rich and interactive graphical user interfaces (GUIs) in Java applications. Among its many components, the JavaFX Slider stands out as a versatile tool that allows users to input a value within a specified range using a draggable slider. In this article, we’ll explore the basics of JavaFX Slider, its usage, and some real-life examples to demonstrate its practicality.
Understanding JavaFX Slider
The JavaFX Slider is a user interface component that enables users to select a value from a specified range by dragging a thumb along a track. It is commonly used for settings, volume control, progress bars, and any other scenario where continuous or discrete value selection is required. The slider is highly customizable, allowing developers to adapt its appearance and behavior to match the application’s theme.
Basic JavaFX Slider Implementation
To get started, let’s look at a simple JavaFX application that includes a Slider and a Label to display its current value:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Slider;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Minimum, Maximum, Initial value
Slider slider = new Slider(0, 100, 50);
Label valueLabel = new Label("Current Value: " + slider.getValue());
// Listen for value changes
slider.valueProperty().addListener((observable, oldValue, newValue) -> {
valueLabel.setText("Current Value: " + newValue.intValue());
});
VBox container = new VBox(20, slider, valueLabel);
container.setAlignment(Pos.CENTER);
this.parent.getChildren().addAll(container);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX Slider");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
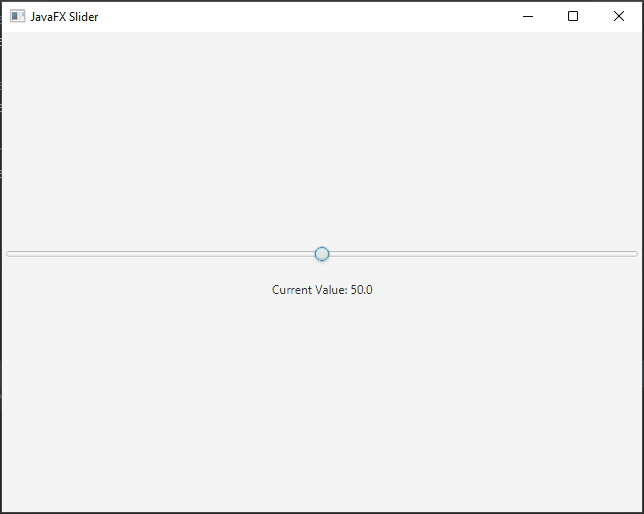
To utilize the JavaFX Slider, we first import the necessary JavaFX classes. Next, we instantiate a Slider object with a range spanning from 0 to 100 and set its initial value to 50. To provide visual feedback, we create a Label element that dynamically displays the current slider value. By attaching a listener to the slider’s valueProperty, we ensure that the label updates in real-time whenever the slider value changes. To achieve an organized layout, we group the slider and label within a VBox (vertical box) container.
Real-life Examples
Volume Control
Sliders are commonly used to adjust volume levels in multimedia applications. Users can drag the slider to set the desired volume for music, videos, or games. The volume level is continuously updated in real-time, providing instant feedback to the user.
Brightness Settings
In image editing or screen brightness control applications, a slider can be utilized to adjust the brightness level. Users can visually see the changes in real-time as they slide the thumb along the track.
Music Player Seek Bar
A slider can serve as a seek bar in a music player, allowing users to change the playback position of a song. This interactive feature enables users to skip forward or backward to a specific section of the track easily.
Temperature Control
In smart home applications or climate control systems, sliders can be employed to set the desired temperature. Users can adjust the slider to increase or decrease the temperature according to their preferences.
Conclusion
The JavaFX Slider is a versatile and intuitive user interface component that offers great flexibility in various application scenarios. Its ability to interactively set values within a specified range makes it a valuable tool for developers to enhance user experiences. By customizing its appearance and behavior, developers can seamlessly integrate sliders into their JavaFX applications for a wide range of real-life use cases. Whether it’s volume control, brightness adjustment, or any other continuous value selection, the JavaFX Slider proves to be an essential element in creating modern and engaging UIs.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!