In JavaScript, objects and arrays are two fundamental data structures used to store and manipulate data. While objects are great for storing key-value pairs, sometimes you need to convert object data into an array format to perform operations like sorting, mapping, or filtering. Converting objects to arrays allows you to take advantage of array methods that make these operations easier and more efficient.
The goal of this article is to explore different ways of converting objects to arrays based on what you need to do with the data. Whether you’re looking to extract object keys, values, or both, or even convert complex nested objects, you’ll learn various methods to achieve this.
Common use cases for converting objects to arrays include:
- Sorting: When you need to sort object values or keys.
- Mapping: Applying transformations to object values.
- Filtering: Removing or selecting certain items based on conditions.
- Manipulating data: Sometimes, object data needs to be handled as arrays to leverage powerful array methods for easier manipulation.
Let’s dive into the different techniques for converting objects into arrays.
Converting Object Keys to an Array
To extract the keys from an object and convert them into an array, you can use the Object.keys()
method. This method returns an array containing the names of all the enumerable properties of the object.
const person = { name: "Samantha", age: 25, city: "Lusaka" };
const keys = Object.keys(person);
console.log(keys); // Output: ["name", "age", "city"]
In the example above, Object.keys(person)
takes the person
object and returns an array with all of its keys: "name"
, "age"
, and "city"
. These are the names of the properties in the object.
The returned array is a flat array of strings, where each string corresponds to a key in the original object. This can be useful if you want to perform operations on just the keys of the object, such as sorting them or mapping them to new values.
Converting Object Values to an Array
To extract the values from an object and convert them into an array, you can use the Object.values()
method. This method returns an array containing all the values associated with the object’s keys.
const person = { name: "Samantha", age: 25, city: "Lusaka" };
const values = Object.values(person);
console.log(values); // Output: ["Samantha", 25, "Lusaka"]
In the example above, Object.values(person)
extracts the values from the person
object and returns an array: ["Samantha", 25, "Lusaka"]
. These values are associated with the keys "name"
, "age"
, and "city"
.
The returned array contains all the values from the object in the same order as the object’s properties. This is useful when you need to manipulate or analyze the values of an object without concern for the keys. For instance, you can filter or map over the values, perform calculations, or any other array operations.
Converting Object Entries to an Array
To convert both keys and values from an object into an array, you can use the Object.entries()
method. This method returns an array of arrays, where each nested array contains a key-value pair.
const person = { name: "Samantha", age: 25, city: "Lusaka" };
const entries = Object.entries(person);
console.log(entries);
In this example, Object.entries(person)
converts the person
object into an array of arrays, where each inner array contains a key-value pair. The result is:
[["name", "Samantha"], ["age", 25], ["city", "Lusaka"]]
Each element in the resulting array is itself an array with two elements: the first element is the object’s key, and the second is the corresponding value. This can be particularly useful when you need to iterate over both keys and values simultaneously, or when you want to transform or manipulate the data in a more flexible way.
For instance, you can use methods like map()
or filter()
on the array of entries to apply transformations to both keys and values together.
Converting Objects with Array Methods (Using map
and reduce
)
You can manipulate object entries to transform them into arrays using higher-order array methods like map()
and reduce()
. These methods give you more control over how you want to process or reformat the object data.
Using map()
to Convert Object into a List of Strings
The map()
method is perfect when you need to iterate over object entries (obtained via Object.entries()
) and create a new array where each element is transformed in a specific way.
const person = { name: "Samantha", age: 25, city: "Lusaka" };
const entries = Object.entries(person);
const result = entries.map(([key, value]) => `${key}: ${value}`);
console.log(result); // Output: ["name: Samantha", "age: 25", "city: Lusaka"]
The Object.entries(person)
method converts the object into an array of key-value pairs. The map()
method then transforms each key-value pair into a string in the format "key: value"
. The output is an array of strings: ["name: Samantha", "age: 25", "city: Lusaka"]
.
Using reduce()
to Convert Object into an Array of Custom Format
The reduce()
method can be used to accumulate a result by processing each key-value pair in the object. It’s useful when you need to construct a custom array or format while iterating through the entries.
const person = { name: "Samantha", age: 25, city: "Lusaka" };
const result = Object.entries(person).reduce((acc, [key, value]) => {
acc.push(`${key}: ${value}`);
return acc;
}, []);
console.log(result); // Output: ["name: Samantha", "age: 25", "city: Lusaka"]
Again, Object.entries(person)
converts the object to an array of key-value pairs. reduce()
is used to accumulate each transformed entry (formatted as "key: value"
) into a new array. The second argument ([]
) is the initial value for the accumulator (acc
). The result is the same as with map()
, but you have more flexibility if you need to apply complex transformations or conditional logic while reducing the entries.
Why Use map()
or reduce()
?
map()
: Ideal for creating a new array by applying a transformation to each element (key-value pair). It’s a simple one-step transformation.reduce()
: Ideal when you need to perform more complex logic, accumulate values, or create a custom data structure based on object entries. It provides more control over the accumulation process.
Both methods are powerful tools for transforming objects into arrays, allowing for flexible and customized output formats.
Converting Arrays of Objects to Arrays (Flattening)
When you have an array of objects and you want to extract specific data or flatten it into a single array, you can use array methods like map()
. This is useful when you have multiple objects within an array and you want to focus on one aspect of each object, such as all the names or all the values of a particular property.
Flattening an Array of Objects
In this case, the goal is to “flatten” the array of objects by extracting a specific property from each object in the array.
const users = [
{ name: "Samantha", age: 25 },
{ name: "Edward", age: 30 }
];
const names = users.map(user => user.name);
console.log(names); // Output: ["Samantha", "Edward"]
The map()
method is used to iterate over each object in the users
array. For each object, we extract the name
property and return it as an element in the new array. The resulting names
array contains all the names from the users
array: ["Samantha", "Edward"]
.
When to Use Flattening
This method is helpful when you need to:
- Extract a specific property from an array of objects.
- Create a new array that is a subset of the original objects.
- Perform operations like sorting or filtering on extracted data.
For example, you can easily extract all the ages or all the cities from an array of user objects, or you could use it in combination with other array methods like filter()
or reduce()
to manipulate or format the data further.
Flattening arrays in this way helps simplify data manipulation when working with complex structures, making it easier to handle and process individual values from a collection of objects.
Converting Object to Array of Specific Values
In some cases, you may want to convert an object to an array but only include certain values based on specific conditions. Using Object.entries()
, along with filter()
and map()
, you can easily achieve this. This approach allows you to focus on particular key-value pairs in the object, filtering and transforming the data as needed.
Extracting Specific Values from an Object
You can first convert the object to an array of key-value pairs using Object.entries()
, then apply filter()
to select the pairs you want, followed by map()
to transform the data as required.
const person = { name: "Samantha", age: 25, city: "Lusaka" };
const filtered = Object.entries(person)
.filter(([key, value]) => key === 'name') // Only select the "name" key
.map(([key, value]) => value); // Extract just the value of "name"
console.log(filtered); // Output: ["Samantha"]
In this example:
Object.entries(person)
: Converts theperson
object into an array of key-value pairs:[["name", "Samantha"], ["age", 25], ["city", "Lusaka"]]
..filter(([key, value]) => key === 'name')
: Filters out all the entries except the one where the key is'name'
, resulting in[["name", "Samantha"]]
..map(([key, value]) => value)
: Maps over the filtered entries and extracts just the values, returning the array["Samantha"]
.
Use Cases for This Approach
- Selective Data Extraction: If you only need to get values for specific keys in an object, like names or certain attributes.
- Conditional Transformation: You can filter the object entries based on more complex conditions, such as numerical thresholds or string matching, and then transform the selected values.
- Dynamic Value Extraction: You can dynamically select which keys to filter by, making this method flexible and reusable.
This approach is a powerful way to extract and transform specific parts of an object when you don’t need to work with all of its properties, making the data more focused and useful for your needs.
Handling Nested Objects
Sometimes, objects can contain other objects within them (nested objects). Converting these nested objects into arrays involves accessing the inner object and then applying the same methods (like Object.entries()
, Object.keys()
, or Object.values()
) to extract the data from the nested structure.
Converting Nested Objects to Arrays
To handle nested objects, you can first access the nested object and then use the standard object-to-array methods. This approach works well when the depth of nesting is known or when you’re interested in extracting specific parts of the nested object.
const person = {
name: "Samantha",
address: { city: "Lusaka", zip: "10101" }
};
// Extracting the entries of the nested 'address' object
const addressEntries = Object.entries(person.address);
console.log(addressEntries); // Output: [["city", "Lusaka"], ["zip", "10101"]]
In this example:
person.address
: Accesses the nestedaddress
object inside theperson
object.Object.entries(person.address)
: Converts theaddress
object into an array of key-value pairs:[["city", "Lusaka"], ["zip", "10101"]]
.
Deep Traversal for More Complex Structures
In cases where you have deeply nested objects (e.g., objects inside objects inside arrays), you may need to perform deep traversal to convert all parts into arrays. This can be done recursively, ensuring that no matter how deep the nesting is, every part gets converted.
const user = {
name: "Samantha",
contact: {
email: "samantha@example.com",
phone: {
home: "555-1234",
mobile: "555-5678"
}
}
};
// Recursively converting all nested objects to arrays
function deepEntries(obj) {
return Object.entries(obj).map(([key, value]) => {
if (typeof value === "object" && !Array.isArray(value)) {
return [key, deepEntries(value)]; // Recursively convert nested objects
}
return [key, value]; // Return key-value pair as is for primitive values
});
}
const userEntries = deepEntries(user);
console.log(userEntries);
// Output: [ [ 'name', 'Samantha' ], [ 'contact', [ [Array], [Array] ] ] ]
In this example:
deepEntries()
: A recursive function that checks if a value is an object. If it is, the function calls itself to convert that object into entries. If it’s not, it simply returns the key-value pair.- Recursive Conversion: This allows you to handle objects of arbitrary depth, converting each nested object into an array of key-value pairs.
Use Cases for Handling Nested Objects
- Complex Data Structures: When working with deeply nested JSON data, such as API responses, and you need to manipulate or display the data in a flat structure.
- Data Transformation: Converting nested objects to arrays is helpful when you need to iterate over the data or apply array-specific methods (like
map()
,filter()
, etc.) to the nested values. - Flattening Data: In some cases, you may need to “flatten” nested data for easier handling or exporting it into a specific format.
This approach allows you to handle complex, nested data structures effectively, making them easier to manipulate and work with in array-based operations.
Practical Example: Extracting Data for Display
In real-world applications, it’s common to need data from an object to display it in a table or a list. For example, when dealing with product data in an e-commerce site, you may need to extract specific details (such as the product name, price, etc.) and present them in a more user-friendly format.
Example: Converting Product Data to an Array for Display
Imagine we have a list of products in an object, where each product has properties like name
and price
. We can extract these details and convert the object into an array of objects that can be easily displayed in a table or a list.
const products = {
"product1": { name: "Laptop", price: 1200 },
"product2": { name: "Phone", price: 800 }
};
// Convert the products object to an array for easier display
const productArray = Object.entries(products).map(([key, product]) => ({
id: key,
name: product.name,
price: product.price
}));
console.log(productArray);
// Output: [
// { id: "product1", name: "Laptop", price: 1200 },
// { id: "product2", name: "Phone", price: 800 }
// ]
In this example:
Object.entries(products)
: Converts theproducts
object into an array of key-value pairs where each key represents the product ID (like"product1"
) and each value is an object with the product details (likename
andprice
).map()
: This method is used to iterate over each key-value pair in the array. For each product, we create a new object with propertiesid
,name
, andprice
for easy display.productArray
: The result is an array of product objects, which is more convenient for rendering in a table, list, or any other display format.
Use Case: Displaying in a Table
This approach is helpful when you need to display data extracted from objects in a structured format. For instance, you could use the productArray
to generate rows in an HTML table or list items in a shopping cart.
<!DOCTYPE html>
<head>
<title>Use Case: Displaying in a Table</title>
</head>
<body>
<table style="width: 100%; text-align: left; padding: 16px;">
<thead>
<tr>
<th>Product ID</th>
<th>Product Name</th>
<th>Price</th>
</tr>
</thead>
<tbody></tbody>
</table>
<script>
const productArray = [
{ id: "product1", name: "Laptop", price: 1200 },
{ id: "product2", name: "Phone", price: 800 }
];
productArray.forEach(product => {
const row = document.createElement('tr');
row.innerHTML = `
<td>${product.id}</td>
<td>${product.name}</td>
<td>${product.price}</td>
`;
document.querySelector('tbody').appendChild(row);
});
</script>
</body>
This code takes the productArray
, creates table rows dynamically, and appends them to the table in the HTML.
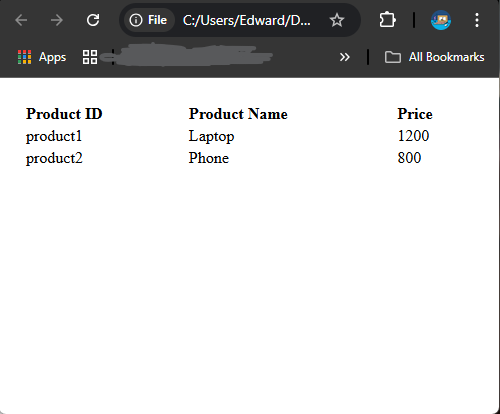
Using Object.entries()
along with map()
is a practical way to convert and manipulate object data into an array of objects for display. This method can be applied to many real-world scenarios, such as rendering lists of items, displaying user information, or generating dynamic content in web applications.
Conclusion
In this article, we explored several ways to convert objects to arrays in JavaScript using Object.keys()
, Object.values()
, Object.entries()
, and other array methods like map()
and reduce()
. Each method serves a specific purpose and can be applied based on the structure of the object and the task at hand.
By converting objects to arrays, you gain the ability to leverage the full power of array methods to manipulate data, such as sorting, filtering, and transforming values. This can simplify tasks like extracting specific parts of an object, flattening nested structures, and preparing data for display.
Ultimately, knowing how to convert objects to arrays is a versatile tool in your JavaScript toolkit. Depending on your needs, you can choose the method that fits best with your data structure, whether you’re working with object keys, values, or key-value pairs.
Feel free to experiment with these methods in different scenarios to become more comfortable with object-to-array conversions and make your data manipulation tasks even easier.