In JavaScript, splitting a string means breaking it into smaller parts, typically based on a delimiter such as spaces, commas, or any other character. This allows you to work with individual pieces of the string, which can be useful for processing or extracting data.
For example, you can split a sentence into an array of words, making it easier to analyze each word separately:
const sentence = "JavaScript is awesome";
const words = sentence.split(" ");
console.log(words); // Output: ["JavaScript", "is", "awesome"]
Splitting strings is a common task when working with text data, as it helps transform a continuous string into a more manageable format (like an array of words, letters, or parts).
Using .split()
with a Single Character
The .split()
method in JavaScript allows you to split a string into an array of substrings based on a specific character or delimiter. When you pass a single character to the .split()
method, it will divide the string wherever that character appears.
Splitting by a Space
A common use case is splitting a string by spaces. This is useful when you want to turn a sentence into individual words.
const sentence = "JavaScript is awesome";
const words = sentence.split(" ");
console.log(words); // Output: ["JavaScript", "is", "awesome"]
In this example, we split the sentence into words by using the space (" "
) as the delimiter.
Splitting by a Comma or Semicolon
You can also split a string by other delimiters, such as commas or semicolons. For instance, if you have a list of items separated by commas, you can split them into an array:
const fruits = "apple,banana,orange";
const fruitArray = fruits.split(",");
console.log(fruitArray); // Output: ["apple", "banana", "orange"]
Similarly, you can use a semicolon as a delimiter:
const items = "pen;book;notebook";
const itemList = items.split(";");
console.log(itemList); // Output: ["pen", "book", "notebook"]
Splitting with No Argument
When the .split()
method is called without any argument, it behaves differently than when a delimiter is provided. In this case, it splits the string into an array, but since there’s no specific delimiter, it returns an array with the entire string as a single element.
Calling .split()
without a parameter will result in an array that contains the original string as its only element. It doesn’t split the string into individual characters or perform any other action.
const greeting = "Hello there";
const result = greeting.split();
console.log(result); // Output: ["Hello there"]
In this example, the string "Hello there"
is returned as an array with one element: the entire string itself.
Here’s another simple example:
const word = "world cup";
const result = word.split();
console.log(result); // Output: ["world cup"]
No delimiter means the string remains intact as a single array element.
Splitting into Characters
If you want to split a string into individual characters, you can use an empty string (''
) as the separator in the .split()
method. This tells JavaScript to break the string at every position between characters.
By passing an empty string ''
to .split()
, the string is split between each character, returning an array where each character is a separate element.
const word = "abc";
const result = word.split('');
console.log(result); // Output: ["a", "b", "c"]
In this example, "abc"
is split into three separate elements: "a"
, "b"
, and "c"
, each as individual items in an array.
Here’s a practical example with a simple word:
const letters = "hello";
const letterArray = letters.split('');
console.log(letterArray); // Output: ["h", "e", "l", "l", "o"]
In this case, "hello"
is split into individual characters, creating an array where each letter is an element.
Using .split()
with a Limit
In JavaScript, the .split()
method can take a second parameter called the limit. This limit controls how many parts the string will be split into.
const example = "a-b-c-d";
const result = example.split('-', 2);
console.log(result); // Output: ["a", "b"]
In this example, the string "a-b-c-d"
is split by the hyphen -
, but the limit is set to 2
. This means only the first two parts—"a"
and "b"
—are included in the result. The rest of the string ("c-d"
) is ignored.
Here’s another example that shows how the limit works with commas:
const phrase = "apple,banana,cherry,dates";
const result = phrase.split(',', 3);
console.log(result); // Output: ["apple", "banana", "cherry"]
Here, the string is split into three parts at the commas. The last item ("dates"
) is not included because the limit is 3
.
Splitting by Multiple Characters
In JavaScript, the .split()
method can also be used to split a string by multi-character separators. This allows you to specify more complex delimiters, such as a combination of characters or a string of multiple characters, to break the string into parts.
To split a string by multiple characters, simply pass the multi-character string as the separator to the .split()
method. JavaScript will then break the string at every occurrence of that separator.
const example = "one::two::three";
const result = example.split("::");
console.log(result); // Output: ["one", "two", "three"]
In this example, the string "one::two::three"
is split by the multi-character delimiter "::"
, resulting in an array of the individual parts: "one"
, "two"
, and "three"
.
Here’s another example using a multi-character separator:
const path = "folder1/folder2/folder3";
const parts = path.split("/");
console.log(parts); // Output: ["folder1", "folder2", "folder3"]
In this case, the string representing a file path is split by the slash (/
), which is a multi-character separator in this context, resulting in an array of folder names.
Using Regular Expressions with .split()
In JavaScript, you can use regular expressions (regex) as the separator in the .split()
method. This allows for more advanced and flexible string splitting, such as splitting by multiple different characters or patterns at once.
With regular expressions, you can specify multiple delimiters to split a string by. For example, you might want to split a string by both commas and semicolons, which can be done by passing a regex that matches both characters.
const example = "a,b;c";
const result = example.split(/[,;]/);
console.log(result); // Output: ["a", "b", "c"]
In this case, the regex /[,;]/
matches either a comma or a semicolon, and the string is split wherever either of these characters appears.
Splitting by Any Whitespace
You can also use regex to split a string by any whitespace character, such as spaces, tabs, or newline characters. The regular expression /\s+/
matches any sequence of whitespace characters.
const string = "dog\tcat\nfish";
const result = string.split(/\s+/);
console.log(result); // Output: ["dog", "cat", "fish"]
In this example, the string "dog\tcat\nfish"
contains tab (\t
) and newline (\n
) characters between words. The regex /\s+/
splits the string wherever any sequence of whitespace characters appears, resulting in an array of the words: "dog"
, "cat"
, and "fish"
.
Combining .split()
with .map()
By combining .split()
with .map()
, you can transform the resulting array after splitting a string into a new array, such as converting string elements into numbers or other data types. This combination is useful when you need to perform additional operations on the individual elements after splitting.
Example: Turning a String of Numbers into an Array of Integers
You can split a string of numbers by a delimiter (like a comma) and then use .map()
to convert each element of the resulting array into an integer using the Number
constructor.
const numbers = "1,2,3";
const result = numbers.split(',').map(Number);
console.log(result); // Output: [1, 2, 3]
In this example, the string "1,2,3"
is first split by commas into an array of strings: ["1", "2", "3"]
. Then, .map(Number)
is used to convert each string element into a number, resulting in an array of integers: [1, 2, 3]
.
Using .split()
on User Input
You can use .split()
on user input to split strings into arrays based on a separator provided by the user. This is useful in many situations, like when a user enters a comma-separated list, and you want to process the individual items.
Example: Split a Comma-Separated List from an Input Box
Let’s say you have a simple form where users can input a list of items separated by commas. You can use .split()
to break the input into an array of items.
Here’s a simple example using an HTML form and JavaScript:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Split User Input</title>
</head>
<body>
<h2>Enter a list of items (comma-separated):</h2>
<input type="text" id="inputBox" placeholder="Enter items...">
<button onclick="splitInput()">Submit</button>
<p id="output"></p>
<script>
function splitInput() {
const input = document.getElementById("inputBox").value;
const items = input.split(",");
document.getElementById("output").textContent = "You entered: " + items.join(", ");
}
</script>
</body>
</html>
In this example, the user enters a list of items, separated by commas, into the input box. When the “Submit” button is clicked, the splitInput()
function is triggered. The function grabs the input value, splits it by commas using .split(",")
, and displays the individual items in the paragraph element.
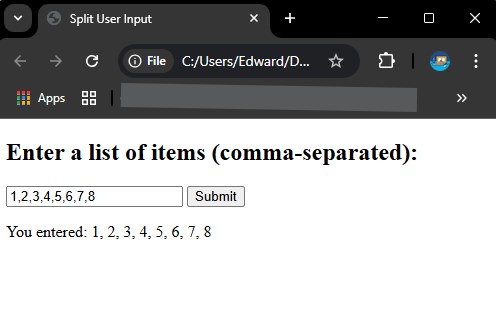
This example is a simple demo idea that can be expanded for more advanced use cases, like handling different delimiters or processing more complex input.
Conclusion
The .split()
method in JavaScript is a powerful tool for breaking down strings into arrays. It offers flexibility by allowing you to split by single or multiple characters, regular expressions, and even user-defined input. Here are some common use cases:
- Splitting by simple characters (like spaces or commas)
- Splitting by multiple delimiters
- Turning strings into arrays of characters or words
- Using
.split()
in combination with methods like.map()
to transform the data
Try experimenting with different separators and practice splitting strings in different ways. It’s a great way to manipulate and process text in JavaScript!