In Python, repeating strings is a simple and powerful way to handle text. It allows you to create multiple copies of a string without writing repetitive code. String repetition is often used for tasks like creating patterns, formatting output, or generating repeated content dynamically.
For example, you might want to create a repeated sequence of characters for a visual pattern, generate separators for text formatting, or repeat a string multiple times based on user input. Python provides straightforward ways to repeat strings, making it an essential tool for many text-based applications.
Using the *
Operator for String Repetition
In Python, you can easily repeat strings using the *
operator. This operator allows you to multiply a string by a number, effectively repeating it that many times. It’s one of the simplest ways to repeat a string in Python.
Syntax:
string * number
Where:
string
is the string you want to repeat.number
is how many times you want to repeat the string.
Example: Repeating a string multiple times
word = "Hello "
repeated_word = word * 3
print(repeated_word)
In this example, the string "Hello "
is repeated 3 times to form "Hello Hello Hello "
. The *
operator makes it quick and easy to repeat the string any number of times, depending on the value of number
.
Repeating Strings with Variables
You can also repeat strings using variables, which allows you to create dynamic and flexible strings based on user input or other program logic. By combining string repetition with variables, you can control the number of repetitions or combine repeated strings with other text.
Example: Using variables to dynamically repeat strings
word = "Python "
repeats = 4
result = word * repeats
print(result)
In this example, the variable word
contains the string "Python "
and the variable repeats
controls how many times the string will be repeated. The *
operator multiplies the string word
by the value stored in repeats
, resulting in the string being repeated 4 times.
Example: Combining repeated strings with other text
word = "Hello "
repeats = 3
result = word * repeats + "World!"
print(result)
Output:

Here, the repeated string "Hello "
is combined with the string "World!"
. The *
operator repeats "Hello "
3 times, and then the +
operator concatenates "World!"
at the end, creating a new dynamic string.
By using variables in this way, you can make your string repetition more flexible and adaptable to various needs in your program.
Using String Multiplication in Loops
You can repeat strings inside a loop to create more complex patterns or perform repetitive tasks. This approach is useful when you want to repeat a string a varying number of times or as part of a pattern, especially when the number of repetitions depends on dynamic values (like user input or a counter).
Example: Repeating a string multiple times in a for
loop to create a pattern
# Create a simple pattern using string repetition in a loop
for i in range(5):
print("*" * (i + 1))
Output:
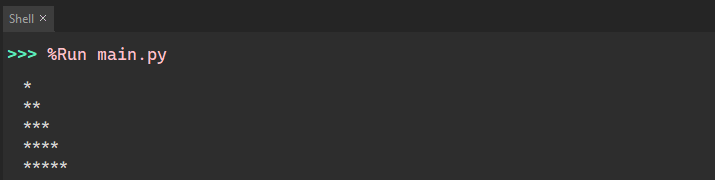
In this example, the for
loop runs 5 times (from 0
to 4
), and each time, the string "*"
is repeated i + 1
times. The i + 1
ensures that the number of stars increases with each iteration, creating a growing pattern.
This method of string multiplication inside a loop can be used to create various text-based shapes or patterns, making it useful for tasks like visual output or text formatting.
Combining String Repetition with Other Operations
You can combine string repetition with other operations, such as concatenation, to create more complex and dynamic strings. This allows you to build longer strings or perform additional formatting while repeating text.
Example: Repeating strings and concatenating them to form a longer string
word = "Hi! "
repeats = 3
message = word * repeats + "Welcome!"
print(message)
In this example, the string "Hi! "
is repeated 3 times using the *
operator. After repetition, the +
operator is used to concatenate "Welcome!"
to the repeated string.
Example: Creating a more dynamic pattern with repetition and concatenation
symbol = "#"
spaces = " "
repeats = 5
pattern = symbol * repeats + spaces * 2 + symbol * repeats
print(pattern)
Output:

Here, the symbol
string ("#"
) is repeated 5 times, then concatenated with 2 spaces (" "
), and followed by another repetition of the symbol. The result forms a custom pattern.
By combining string repetition with concatenation, you can easily manipulate and format strings to fit specific requirements, making your code both flexible and efficient.
Repeating Strings with join()
(for more complex patterns)
The join()
method in Python allows you to join multiple strings together with a specified separator. It offers more control over how repeated strings are combined, especially when you want to insert a separator between repetitions, such as a space, comma, or custom character.
Unlike the *
operator, which simply repeats a string a fixed number of times, join()
can be useful for creating more structured or complex patterns with repeated strings.
Example: Repeating strings with separators using join()
word = "Python"
repeats = 4
separator = ", "
result = separator.join([word] * repeats)
print(result)
Output:

In this example, the join()
method takes a list of repeated strings (created using [word] * repeats
). The separator
(,
) is used to insert a comma and a space between each repeated instance of the string.
By using join()
, you gain more flexibility in how the strings are repeated and combined, making it ideal for creating complex patterns or formatted output with repeated elements.
Repeating Strings with String Formatting
You can also use string formatting methods, like f-strings or .format()
, to create repeated patterns or dynamically repeat text within a formatted string. While not as direct as the *
operator or join()
, formatting methods can be useful for adding structure and variables into the repeated strings.
Example: Using f-strings for repeating text with formatting
word = "Hello"
repeats = 3
result = f"{word} " * repeats
print(result)
In this example, an f-string is used, where {word}
is repeated 3 times by multiplying the entire string pattern f"{word} "
with the repeats
variable. This approach is useful when you want to embed variables inside the repeated string.
Example: Using .format()
for string repetition with formatting
word = "Goodbye"
repeats = 2
result = "{} ".format(word) * repeats
print(result)
Here, .format()
is used to insert the string word
into a formatted string, and then this formatted string is repeated using the *
operator. This allows you to combine the flexibility of string formatting with repetition.
Both methods—f-strings and .format()
—allow you to repeat and format text dynamically, especially when the repetition needs to incorporate variables or complex structures.
Repeating Strings for Creating Patterns or Shapes
String repetition can be a fun and creative way to generate text-based graphics, such as shapes or patterns. By repeating strings and arranging them in loops, you can create visually appealing output, like boxes, triangles, or other designs.
Example: Using string repetition to create a box
# Creating a simple box with string repetition
width = 5
height = 3
row = "*" * width
for i in range(height):
print(row)
In this example, the *
character is repeated to create a row of stars, and the loop prints that row multiple times to form a box.
Example: Creating a triangle pattern
# Creating a simple triangle pattern using string repetition
height = 5
for i in range(1, height + 1):
print("*" * i)
Here, the number of stars increases with each row, creating a triangle shape. The loop controls the repetition, ensuring the number of stars grows incrementally.
Example: Creating a checkerboard pattern
# Creating a checkerboard pattern using string repetition
size = 4
for i in range(size):
if i % 2 == 0:
print("* " * size)
else:
print(" *" * size)
This example uses string repetition combined with conditional logic to alternate the pattern, creating a simple checkerboard effect.
By repeating strings in loops and combining them with different characters or logic, you can create various text-based shapes, designs, and patterns for fun or visual representation in Python programs.
Conclusion
In this article, we’ve explored several methods for repeating strings in Python. Here’s a quick recap of the key techniques:
- Using the
*
Operator: The simplest way to repeat a string a fixed number of times. - Loops: Repeating strings inside loops allows for more dynamic patterns and control over repetition.
join()
Method: Useful for repeating strings with a separator, offering more control over formatting.- String Formatting: Techniques like f-strings and
.format()
provide a flexible way to repeat and format strings with variables or more complex structures.
These methods give you the flexibility to create everything from simple repetitions to complex patterns and text-based graphics. By combining them, you can dynamically generate output tailored to your needs, whether it’s for formatting, visual design, or complex data manipulation.