JavaFX is a popular framework for building desktop applications with rich user interfaces. While it provides a range of standard controls, there are times when you need to customize these controls to meet specific design or functionality requirements. Adding icons to text fields is a common customization that can enhance the user experience. In this article, we will explore how to create custom text fields with icons in JavaFX using the ControlsFX library.
What is ControlsFX?
ControlsFX is an open-source library that extends JavaFX by providing custom controls, enhancements, and other useful features to simplify and enhance your JavaFX application’s user interface. It includes a wide range of controls and utilities that can save you time and effort when building JavaFX applications.
Setting Up ControlsFX
Before we dive into creating custom text fields with icons, make sure you have Java and JavaFX installed on your system. Additionally, you’ll need to include the ControlsFX library in your project. You can download it from ControlsFX GitHub.
Creating Custom Text Fields
ControlsFX provides a CustomTextField class that allows you to create text fields with custom icons. Let’s start by creating a simple JavaFX application that demonstrates how to use CustomTextField.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.ImageView;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.textfield.CustomTextField;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the CustomTextField
CustomTextField customTextField = new CustomTextField();
// Set the CustomTextField width to 200px
customTextField.setMaxWidth(200);
// Replace "icon.png" with your icon's path
customTextField.setLeft(new ImageView("icon.png"));
// Add the CustomTextField to the center of the BorderPane
this.parent.setCenter(customTextField);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text Field Icons in JavaFX with ControlsFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we create a simple JavaFX application with a CustomTextField. We set the left icon of the text field using the setLeft method, providing an ImageView with the path to your desired icon. You should replace “icon.png” with the actual path to your icon image.
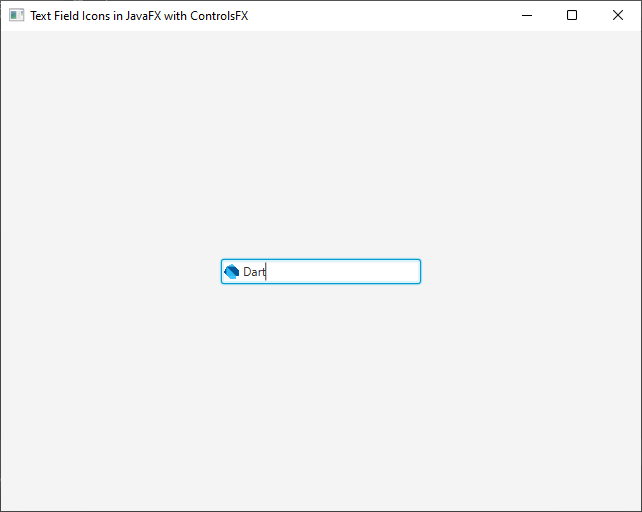
Creating Custom Password Fields
ControlsFX also provides a CustomPasswordField class that allows you to create password fields with custom icons. Let’s create a JavaFX application that demonstrates how to use CustomPasswordField.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.ImageView;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import org.controlsfx.control.textfield.CustomPasswordField;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the CustomPasswordField
CustomPasswordField customPasswordField = new CustomPasswordField();
// Set the CustomPasswordField width to 200px
customPasswordField.setMaxWidth(200);
// Replace "lock.png" with your icon's path
customPasswordField.setLeft(new ImageView("lock.png"));
// Add the CustomPasswordField to the center of the BorderPane
this.parent.setCenter(customPasswordField);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text Field Icons in JavaFX with ControlsFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we create a JavaFX application with a CustomPasswordField. We set the left icon of the password field using the setLeft method, just like we did with the CustomTextField.
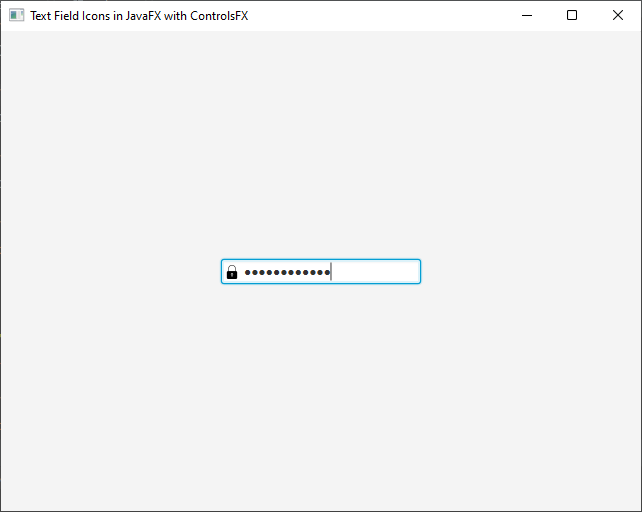
Conclusion
ControlsFX is a valuable library for extending the capabilities of JavaFX applications. By using CustomTextField and CustomPasswordField from ControlsFX, you can easily create custom text fields and password fields with icons to enhance the user experience of your JavaFX applications. This customization can make your application more visually appealing and user-friendly. Start exploring the possibilities of ControlsFX to take your JavaFX development to the next level.
Sources:
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!