JavaFX, a powerful library for building graphical user interfaces (GUIs) in Java, provides developers with a variety of tools to create interactive and visually appealing applications. One such tool is the DirectoryChooser, which allows users to select directories (folders) from their local file system. In this article, we will explore the JavaFX DirectoryChooser in detail, including its features, benefits, and usage through comprehensive code examples.
What is the DirectoryChooser?
The DirectoryChooser is a class in the JavaFX library that facilitates the selection of directories (folders) by users through a graphical user interface. It offers an intuitive way for users to pick a directory from their file system, providing them with a familiar interface and improving the overall user experience of your application.
Why Use the DirectoryChooser?
Integrating a DirectoryChooser into your JavaFX application brings several benefits:
- User-Friendly Interface: The DirectoryChooser dialog provides users with a straightforward and consistent method for selecting directories, ensuring a more intuitive and user-friendly experience.
- Platform Independence: JavaFX applications can run on multiple platforms, including Windows, macOS, and Linux. The DirectoryChooser abstracts the file system differences between these platforms, allowing your application to work seamlessly across all of them.
- Enhanced Functionality: By enabling users to select directories, your application can provide features like specifying save locations, opening directories for file manipulation, or setting working directories.
- Consistency: Utilizing the JavaFX DirectoryChooser ensures a consistent look and feel across different JavaFX applications, contributing to a cohesive user experience.
Basic Usage of DirectoryChooser
Let’s start with a simple example that demonstrates how to use the DirectoryChooser to open a directory selection dialog and retrieve the selected directory’s path.
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.DirectoryChooser;
import javafx.stage.Stage;
import java.io.File;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Button button = new Button("Browse...");
Label label = new Label("No directory selected.");
button.setOnAction(actionEvent -> this.onBrowse(actionEvent, label));
// Add the Button and the Label to the VBox container
VBox container = new VBox(15, button, label);
container.setAlignment(Pos.CENTER);
// Add the VBox container to the BorderPane layout manager
this.parent.setCenter(container);
}
private void onBrowse(ActionEvent actionEvent, Label label) {
Stage stage = (Stage) ((Button) actionEvent.getSource()).getScene().getWindow();
DirectoryChooser directoryChooser = new DirectoryChooser();
// Set the Dialog title
directoryChooser.setTitle("Select a Directory");
File selectedDirectory = directoryChooser.showDialog(stage);
if (selectedDirectory != null) {
label.setText("Selected Directory: " + selectedDirectory.getAbsolutePath());
} else {
label.setText("No directory selected.");
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX DirectoryChooser");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we create an instance of DirectoryChooser and set its title. The showDialog method displays the directory selection dialog to the user. The selected directory’s path is then obtained using the getAbsolutePath method of the returned File object.
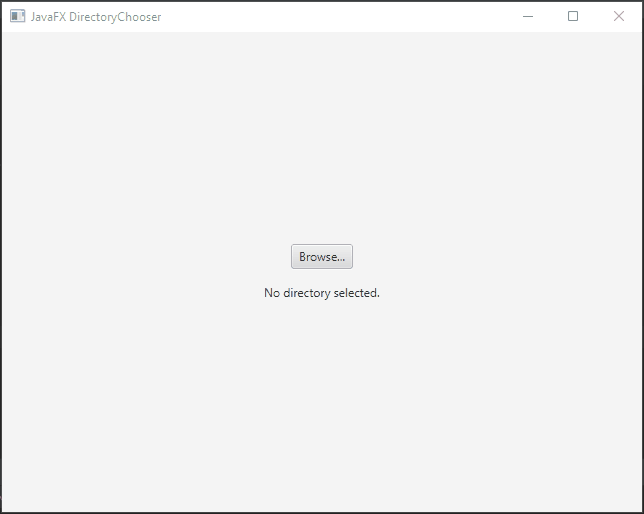
Setting the Initial Directory
The DirectoryChooser class allows for additional configuration to enhance the user experience. You can set an initial directory that the dialog will open to. Here’s an example of how to set an initial directory:
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.DirectoryChooser;
import javafx.stage.Stage;
import java.io.File;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
Button button = new Button("Browse...");
Label label = new Label("No directory selected.");
button.setOnAction(actionEvent -> this.onBrowse(actionEvent, label));
// Add the Button and the Label to the VBox container
VBox container = new VBox(15, button, label);
container.setAlignment(Pos.CENTER);
// Add the VBox container to the BorderPane layout manager
this.parent.setCenter(container);
}
private void onBrowse(ActionEvent actionEvent, Label label) {
Stage stage = (Stage) ((Button) actionEvent.getSource()).getScene().getWindow();
DirectoryChooser directoryChooser = new DirectoryChooser();
// Set the Dialog title
directoryChooser.setTitle("Select a Directory");
// Set initial directory
File initialDirectory = new File(System.getProperty("user.home"));
directoryChooser.setInitialDirectory(initialDirectory);
File selectedDirectory = directoryChooser.showDialog(stage);
if (selectedDirectory != null) {
label.setText("Selected Directory: " + selectedDirectory.getAbsolutePath());
} else {
label.setText("No directory selected.");
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX DirectoryChooser");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we set the initial directory to the user’s home directory using System.getProperty(“user.home”).
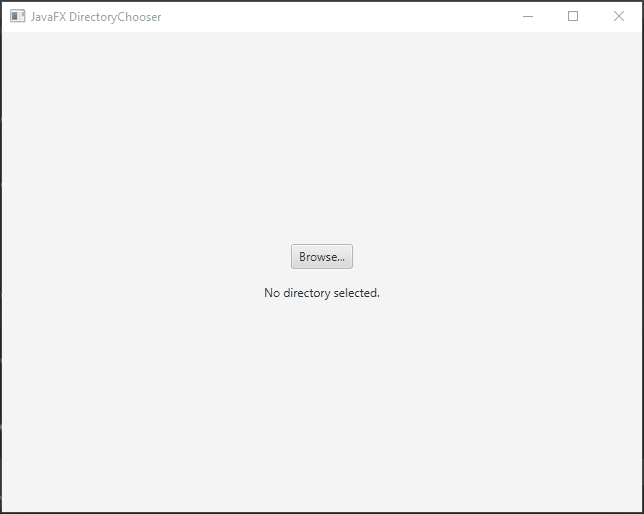
Conclusion
The JavaFX DirectoryChooser class simplifies the process of allowing users to select directories within your desktop applications. By integrating this class into your JavaFX application, you can provide a familiar and user-friendly experience for users when choosing directories. This article has provided a comprehensive overview of the DirectoryChooser class along with code examples, enabling you to seamlessly incorporate directory selection functionality into your JavaFX projects.
Sources:
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!