WebSockets have revolutionized web development by enabling real-time, bidirectional communication between clients and servers. With the power of JavaScript, developers can harness this technology to create interactive and dynamic web applications. In this blog post, we will explore how to use WebSockets with JavaScript.
Firstly, you will need a WebSocket server. However, in this blog post, we will be writing our own using the ws (WebSocket) library. Node.js is required for this task, so if you don’t have it installed on your computer, I highly recommend installing it globally.
Now that we have Node.js installed, we need to install the ws library using npm. You can do this by running the following command in your terminal:
npm install ws
This command will download and install the latest version of the ws library in your project directory, along with its dependencies.
Now it’s time to write our WebSocket server. The code below demonstrates how to write a simple WebSocket server. The server doesn’t do much, it simply sends the current time to the client every second:
// import the WebSocket library
const { Server } = require('ws');
// create a new WebSocket server instance
const wss = new Server({ port: 8080 });
// listen for connections to the WebSocket server
wss.on('connection', (client) => {
console.log('Client connected!');
// send a message to the client every second
const interval = setInterval(() => {
// get the current time as a string
const timeString = (new Date()).toLocaleTimeString();
// send the time string to the client
client.send(timeString);
}, 1000);
// listen for the client to close the connection
client.on('close', () => {
console.log('Client disconnected!');
// stop sending messages to the client
clearInterval(interval);
});
});
Now that the server is implemented and serving data correctly, we need a client to receive the data. After all, what good is a server without anyone or anything to serve the data to? Typically, servers act as bridges between two entities, such as a database and a client.
Here is an HTML file with JavaScript that connects to the WebSocket server we just created. It listens for messages sent by the server and displays them in a div element with an id of “time”.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>How to Use WebSockets with JavaScript</title>
<style>
html * {
padding: 0;
margin: 0;
}
body {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
}
#time {
font-size: 2em;
font-weight: bold
}
</style>
</head>
<body>
<div id="time"></div>
<script>
// establish a WebSocket connection to a server at ws://localhost:8080
const ws = new WebSocket("ws://localhost:8080");
// event listener for when the connection is successfully opened
ws.addEventListener("open", () => {
console.log('WebSocket connection has been established');
});
// event listener for when a message is received from the server
ws.addEventListener("message", ({ data }) => {
// update the text content of an HTML element with an ID of "time" with the received message data
const timeEl = document.querySelector("#time");
timeEl.textContent = data;
});
</script>
</body>
</html>
We have created the WebSocket server and the client. Now it’s time to test our implementation. To run the server, all you need to do is enter the following command in the terminal:
node server.js
This will start the server on port 8080, which is the same port that the client is configured to connect to. Once the server is running, you can open the HTML file in a web browser to test the WebSocket connection. If everything is working correctly, the current time should be displayed in the div element with the id “time”, and it should update every second.
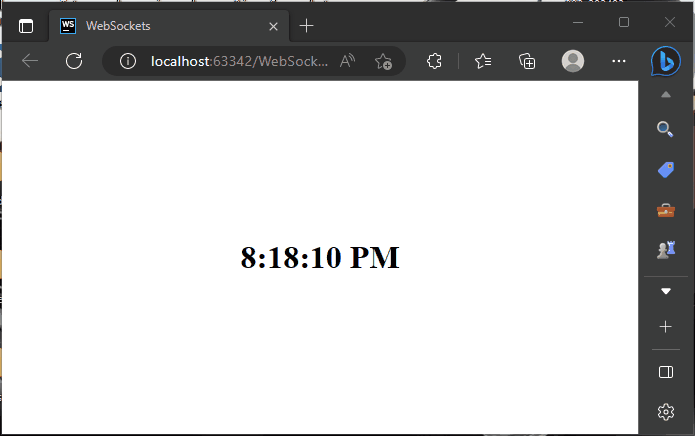
That’s it! We have successfully created a basic WebSocket server and client. I hope you find the code helpful. If you wish to learn more about JavaScript, please subscribe to our newsletter today and continue your JavaScript learning journey with us!