If you’re working on a JavaFX application and need to export data from a TableView to an Excel spreadsheet, Apache POI (Poor Obfuscation Implementation) is an excellent library to help you achieve this task. Apache POI allows you to create, modify, and extract Microsoft Office files, making it perfect for exporting data to Excel. In this article, we will guide you through the process of exporting data from a JavaFX TableView to an Excel file.
What is Apache POI?
Apache POI is a widely used open-source Java library provided by the Apache Software Foundation. It allows Java developers to create, modify, and extract data from Microsoft Office files, including Word documents, PowerPoint presentations, and Excel spreadsheets. In our case, we will focus on exporting data to Excel files, also known as XLSX files.
Installation of Apache POI
To get started with Apache POI, you’ll need to include its libraries in your JavaFX project. You can do this using Maven by adding the following dependencies to your pom.xml file:
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.2.4</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.4</version>
</dependency>
Or you can download the JAR files directly from the Apache POI website and include them in your project manually.
User Record Class
To export JavaFX table data to Excel, we first need a class that represents the data we want to export. Let’s create a simple User record class to represent user records.
public record User(int id, String name, int age, String gender, String language, String country) {
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getGender() {
return gender;
}
public String getLanguage() {
return language;
}
public String getCountry() {
return country;
}
}
In this example, we’ve created a simple User record class to represent user data. The class contains fields for id, name, age, gender, language and country. You can extend this class as needed to match the structure of your data.
Creating a Table
To display the user data in a JavaFX table, you’ll need to create a UI component to hold the data. In this example, we’ll use a TableView to achieve this. Let’s create a simple JavaFX application that displays user data in a table:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.scene.Scene;
import java.io.IOException;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() throws IOException {
ObservableList<User> users = UserData.getUsers();
// Create a UserTableView to display the data
UserTableView userTableView = new UserTableView(users);
// Add the TableView to the parent layout
this.parent.getChildren().add(userTableView);
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Exporting JavaFX Table Data to Excel");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
class UserTableView extends TableView<User> {
public UserTableView(ObservableList<User> users) {
super(users);
// Create TableColumn instances for each attribute
TableColumn<User, Integer> idColumn = this.createColumn("ID", "id");
TableColumn<User, String> nameColumn = this.createColumn("Name", "name");
TableColumn<User, Integer> ageColumn = this.createColumn("Age", "age");
TableColumn<User, String> genderColumn = this.createColumn("Gender", "gender");
TableColumn<User, String> languageColumn = this.createColumn("Language", "language");
TableColumn<User, String> countryColumn = this.createColumn("Country", "country");
// Add the TableColumn instances to the TableView
this.getColumns().addAll(idColumn, nameColumn, ageColumn, genderColumn, languageColumn, countryColumn);
}
private <T> TableColumn<User, T> createColumn(String title, String property) {
TableColumn<User, T> column = new TableColumn<>(title);
column.setCellValueFactory(new PropertyValueFactory<>(property));
return column;
}
}
class UserData {
public static ObservableList<User> getUsers() {
ObservableList<User> userList = FXCollections.observableArrayList();
// Add 20 dummy user data
userList.add(new User(1, "Alice", 25, "Female", "English", "USA"));
userList.add(new User(2, "Bob", 30, "Male", "Spanish", "Spain"));
userList.add(new User(3, "Charlie", 22, "Male", "French", "France"));
userList.add(new User(4, "David", 28, "Male", "German", "Germany"));
userList.add(new User(5, "Eve", 33, "Female", "Italian", "Italy"));
userList.add(new User(6, "Frank", 29, "Male", "Dutch", "Netherlands"));
userList.add(new User(7, "Grace", 27, "Female", "Japanese", "Japan"));
userList.add(new User(8, "Hannah", 24, "Female", "Korean", "South Korea"));
userList.add(new User(9, "Ivan", 35, "Male", "Russian", "Russia"));
userList.add(new User(10, "Jasmine", 23, "Female", "Arabic", "UAE"));
userList.add(new User(11, "Kevin", 31, "Male", "Chinese", "China"));
userList.add(new User(12, "Lily", 26, "Female", "Portuguese", "Portugal"));
userList.add(new User(13, "Michael", 32, "Male", "Swedish", "Sweden"));
userList.add(new User(14, "Nora", 21, "Female", "Danish", "Denmark"));
userList.add(new User(15, "Oliver", 34, "Male", "Greek", "Greece"));
userList.add(new User(16, "Penny", 28, "Female", "Turkish", "Turkey"));
userList.add(new User(17, "Quincy", 29, "Male", "Finnish", "Finland"));
userList.add(new User(18, "Rachel", 25, "Female", "Norwegian", "Norway"));
userList.add(new User(19, "Sam", 27, "Male", "Polish", "Poland"));
userList.add(new User(20, "Tina", 26, "Female", "Hungarian", "Hungary"));
return userList;
}
}
In the above code, we’ve created a simple JavaFX application that displays user data in a table. Each column corresponds to a property of the User class. The sample data is added to the table for demonstration purposes.
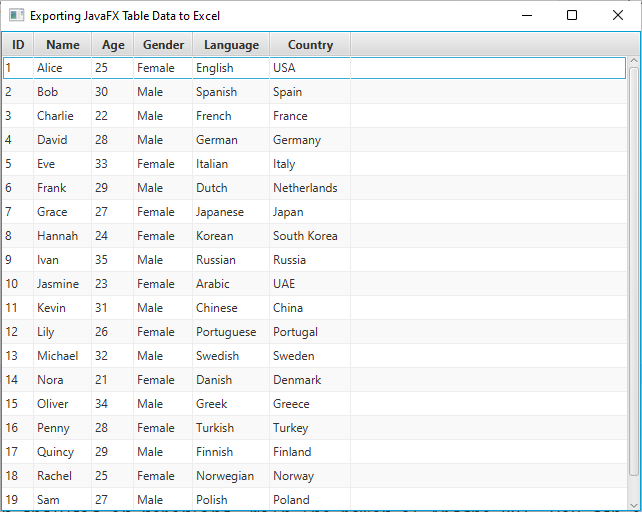
Adding Export Functionality
Now, let’s create a utility class ExcelExporter that handles exporting data from the TableView to an Excel file. We’ll use Apache POI for this purpose.
import javafx.collections.ObservableList;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelExporter {
public static <T> void exportToExcel(TableView<T> tableView, String sheetName, String filePath) throws IOException {
try (Workbook workbook = new XSSFWorkbook()) {
Sheet sheet = workbook.createSheet(sheetName);
ObservableList<TableColumn<T, ?>> columns = tableView.getColumns();
// Create header row
Row headerRow = sheet.createRow(0);
for (int i = 0; i < columns.size(); i++) {
headerRow.createCell(i).setCellValue(columns.get(i).getText());
}
// Fill data
ObservableList<T> items = tableView.getItems();
for (int rowIdx = 0; rowIdx < items.size(); rowIdx++) {
Row row = sheet.createRow(rowIdx + 1);
T item = items.get(rowIdx);
for (int colIdx = 0; colIdx < columns.size(); colIdx++) {
Object cellValue = columns.get(colIdx).getCellData(item);
if (cellValue != null) {
row.createCell(colIdx).setCellValue(cellValue.toString());
}
}
}
// Save the workbook to a file
try (FileOutputStream fileOut = new FileOutputStream(filePath)) {
workbook.write(fileOut);
}
}
}
}
To use the ExcelExporter class, you can call the exportToExcel method, passing in your TableView, the desired sheet name, and the file path where you want to save the Excel file. Here’s an example of how to use it:
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.control.Button;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.StackPane;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import javafx.scene.Scene;
import java.io.File;
import java.io.IOException;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() throws IOException {
ObservableList<User> users = UserData.getUsers();
// Create a UserTableView to display the data
UserTableView userTableView = new UserTableView(users);
// Create a BorderPane to arrange the TableView and export button
BorderPane borderPane = new BorderPane(userTableView);
// Create an "Export to Excel" button
Button exportButton = new Button("Export to Excel");
// Set an action for the export button
exportButton.setOnAction(e -> {
try {
exportTable(userTableView);
} catch (IOException ex) {
// Handle any exceptions that occur during the export process
System.out.println("Couldn't export table data.");
throw new RuntimeException(ex);
}
});
// Add the export button to the bottom of the BorderPane
borderPane.setBottom(exportButton);
BorderPane.setAlignment(exportButton, Pos.CENTER);
BorderPane.setMargin(exportButton, new Insets(10));
// Add the BorderPane to the parent layout
this.parent.getChildren().add(borderPane);
}
private void exportTable(UserTableView tableView) throws IOException {
FileChooser fileChooser = new FileChooser();
fileChooser.getExtensionFilters().add(new FileChooser.ExtensionFilter("Excel Files", "*.xlsx"));
File file = fileChooser.showSaveDialog(tableView.getScene().getWindow());
if (file != null) {
ExcelExporter.exportToExcel(tableView, "FXUserData", file.getAbsolutePath());
}
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Exporting JavaFX Table Data to Excel");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
class UserTableView extends TableView<User> {
public UserTableView(ObservableList<User> users) {
super(users);
// Create TableColumn instances for each attribute
TableColumn<User, Integer> idColumn = this.createColumn("ID", "id");
TableColumn<User, String> nameColumn = this.createColumn("Name", "name");
TableColumn<User, Integer> ageColumn = this.createColumn("Age", "age");
TableColumn<User, String> genderColumn = this.createColumn("Gender", "gender");
TableColumn<User, String> languageColumn = this.createColumn("Language", "language");
TableColumn<User, String> countryColumn = this.createColumn("Country", "country");
// Add the TableColumn instances to the TableView
this.getColumns().addAll(idColumn, nameColumn, ageColumn, genderColumn, languageColumn, countryColumn);
}
private <T> TableColumn<User, T> createColumn(String title, String property) {
TableColumn<User, T> column = new TableColumn<>(title);
column.setCellValueFactory(new PropertyValueFactory<>(property));
return column;
}
}
class UserData {
public static ObservableList<User> getUsers() {
ObservableList<User> userList = FXCollections.observableArrayList();
// Add 20 dummy user data
userList.add(new User(1, "Alice", 25, "Female", "English", "USA"));
userList.add(new User(2, "Bob", 30, "Male", "Spanish", "Spain"));
userList.add(new User(3, "Charlie", 22, "Male", "French", "France"));
userList.add(new User(4, "David", 28, "Male", "German", "Germany"));
userList.add(new User(5, "Eve", 33, "Female", "Italian", "Italy"));
userList.add(new User(6, "Frank", 29, "Male", "Dutch", "Netherlands"));
userList.add(new User(7, "Grace", 27, "Female", "Japanese", "Japan"));
userList.add(new User(8, "Hannah", 24, "Female", "Korean", "South Korea"));
userList.add(new User(9, "Ivan", 35, "Male", "Russian", "Russia"));
userList.add(new User(10, "Jasmine", 23, "Female", "Arabic", "UAE"));
userList.add(new User(11, "Kevin", 31, "Male", "Chinese", "China"));
userList.add(new User(12, "Lily", 26, "Female", "Portuguese", "Portugal"));
userList.add(new User(13, "Michael", 32, "Male", "Swedish", "Sweden"));
userList.add(new User(14, "Nora", 21, "Female", "Danish", "Denmark"));
userList.add(new User(15, "Oliver", 34, "Male", "Greek", "Greece"));
userList.add(new User(16, "Penny", 28, "Female", "Turkish", "Turkey"));
userList.add(new User(17, "Quincy", 29, "Male", "Finnish", "Finland"));
userList.add(new User(18, "Rachel", 25, "Female", "Norwegian", "Norway"));
userList.add(new User(19, "Sam", 27, "Male", "Polish", "Poland"));
userList.add(new User(20, "Tina", 26, "Female", "Hungarian", "Hungary"));
return userList;
}
}
In this example, we’ve added an “Export to Excel” button that, when clicked, opens a file chooser dialog, allowing the user to select the location and name for the Excel file to be exported. It then calls the exportToExcel method to export the data to the selected file. Error handling is also included to deal with any exceptions that may occur during the export process.
With this code, you can easily export data from your JavaFX TableView to an Excel file using Apache POI. This can be a valuable feature for applications that need to generate reports or share data with users who prefer working with Excel.
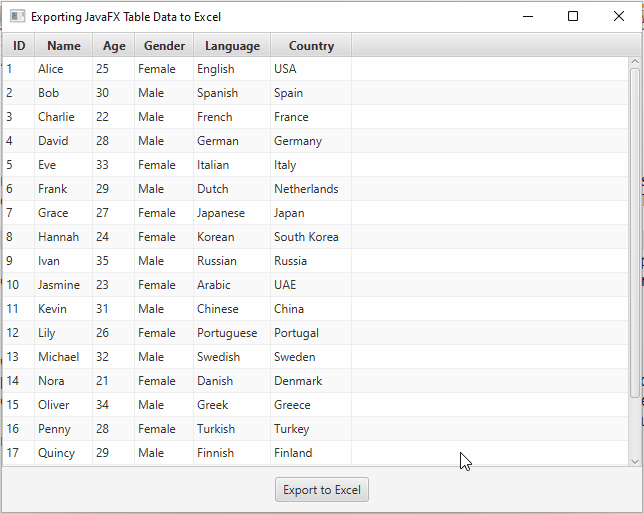
Conclusion
In this article, we explored how to export JavaFX table data to an Excel spreadsheet using the Apache POI library. We learned about Apache POI, how to set up the library in your project, and how to create an Excel workbook, add a sheet, populate it with data, and save the workbook to a file. This is a useful skill for JavaFX developers who need to provide their users with the ability to export data for further analysis or reporting. With the power of Apache POI, you can easily bridge the gap between your JavaFX application and Excel, making data export a breeze.
Related:
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.