Icons are an essential element in modern user interfaces, aiding in conveying meaning, enhancing user experience, and making applications more visually appealing. FontAwesome is a popular icon library that provides a wide range of scalable vector icons that can be easily integrated into web and desktop applications. In this article, we will explore how to use FontAwesome icons in JavaFX applications using the FontAwesomeFX library, providing you with the tools to create dynamic and engaging user interfaces.
What is FontAwesomeFX?
FontAwesomeFX is a JavaFX-specific library that acts as a bridge between the FontAwesome icon library and JavaFX applications. It simplifies the process of integrating FontAwesome icons into JavaFX applications by providing an API to access and display these icons as regular JavaFX nodes.
Adding FontAwesomeFX to Your Project
The first step is to include the FontAwesomeFX library in your JavaFX project. You can do this by adding the Maven dependency to your project’s build configuration:
<dependency>
<groupId>de.jensd</groupId>
<artifactId>fontawesomefx</artifactId>
<version>8.9</version>
</dependency>
Alternatively, if you’re not using Maven, you can download the FontAwesomeFX JAR file from JARDownload and add it to your project’s classpath.
Using FontAwesome Icons in JavaFX
Once you have added the FontAwesomeFX library to your project, you can start using FontAwesome icons in your JavaFX application. The FontAwesomeFX library provides a wide range of icons, each represented by a unique icon code.
You can create FontAwesome icons using the FontAwesomeIconView class. Here’s an example of creating FontAwesome icons and displaying them in a JavaFX application:
import de.jensd.fx.glyphs.fontawesome.FontAwesomeIcon;
import de.jensd.fx.glyphs.fontawesome.FontAwesomeIconView;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create FontAwesome icons
FontAwesomeIconView androidIcon = new FontAwesomeIconView(FontAwesomeIcon.ANDROID);
FontAwesomeIconView googleIcon = new FontAwesomeIconView(FontAwesomeIcon.GOOGLE);
FontAwesomeIconView windowsIcon = new FontAwesomeIconView(FontAwesomeIcon.WINDOWS);
FontAwesomeIconView appleIcon = new FontAwesomeIconView(FontAwesomeIcon.APPLE);
FontAwesomeIconView amazonIcon = new FontAwesomeIconView(FontAwesomeIcon.AMAZON);
// Set the icon size
String iconSize = "50px";
androidIcon.setSize(iconSize);
googleIcon.setSize(iconSize);
windowsIcon.setSize(iconSize);
appleIcon.setSize(iconSize);
amazonIcon.setSize(iconSize);
// Create a VBox container to hold the icons with spacing
VBox iconContainer = new VBox(10, androidIcon, googleIcon, windowsIcon, appleIcon, amazonIcon);
iconContainer.setAlignment(Pos.CENTER);
// Add the VBox icon container to the center of the BorderPane
this.parent.setCenter(iconContainer);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("FontAwesome Icons in JavaFX with FontAwesomeFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we have created icons representing Android, Google, Windows, Apple, and Amazon logos. These icons are displayed with a size of 50 pixels each and are arranged vertically within a centrally aligned VBox container. This VBox is subsequently positioned at the center of a BorderPane layout.
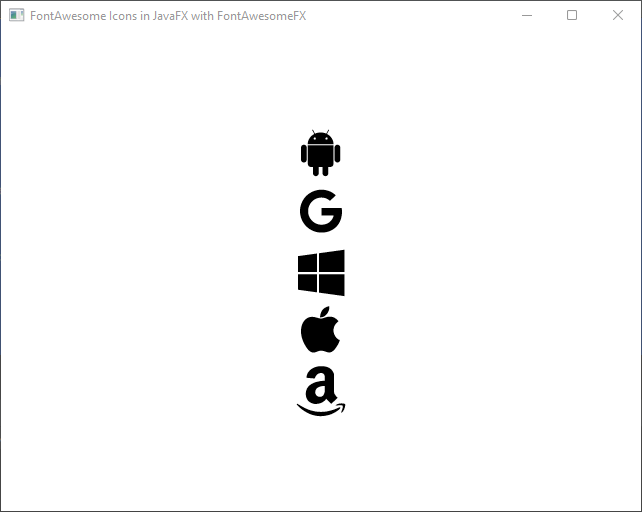
Adding FontAwesome Icons to JavaFX Nodes
You can easily add FontAwesome icons to your JavaFX nodes, such as labels and buttons. Here’s an example of how to set a FontAwesome icon to a Label:
import de.jensd.fx.glyphs.fontawesome.FontAwesomeIcon;
import de.jensd.fx.glyphs.fontawesome.FontAwesomeIconView;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create FontAwesome icon
FontAwesomeIconView androidIcon = new FontAwesomeIconView(FontAwesomeIcon.ANDROID);
// Create the Label
Label label = new Label("Android");
// Set the icon as the graphic
label.setGraphic(androidIcon);
// Increase the Label font size
label.setStyle("-fx-font-size: 16px");
// Add the Label to the center of the BorderPane
this.parent.setCenter(label);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("FontAwesome Icons in JavaFX with FontAwesomeFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we have created a label that displays the text “Android” and positions it at the center of the application window. The application utilizes FontAwesomeFX to generate the Android icon, which is subsequently included in the label as a graphic. To enhance visibility, the label’s font size is increased, and the overall user interface is structured using a BorderPane layout.
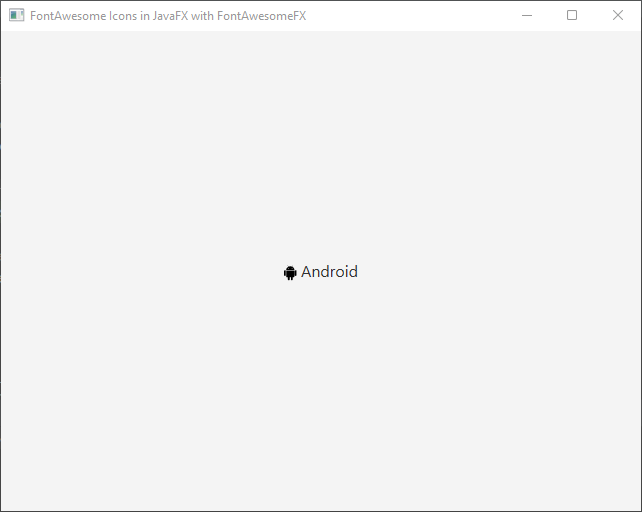
Conclusion
Integrating FontAwesome icons into your JavaFX application using the FontAwesomeFX library is a straightforward process that can significantly enhance the visual appeal and usability of your user interface. With just a few lines of code, you can seamlessly incorporate a wide variety of icons into your application, adding an extra layer of interactivity and engagement for your users. So go ahead and experiment with FontAwesomeFX to create more dynamic and visually pleasing JavaFX applications.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!