Python has gained immense popularity as a versatile and powerful programming language, and its range of libraries and frameworks contributes to its widespread adoption. When it comes to building graphical user interfaces (GUIs) for desktop and mobile applications, PyQt emerges as an excellent choice.
In this article, we will introduce PyQt, a set of Python bindings for the Qt application framework. We’ll explore what PyQt is, why it’s a compelling option for GUI development, and how to get started with it.
What is PyQt?
PyQt is a set of Python bindings for the Qt application framework. Qt is a popular cross-platform application development framework, widely used for building graphical user interfaces (GUIs) and applications that can run on various platforms such as Windows, macOS, Linux, and mobile devices.
PyQt allows Python developers to utilize the powerful features of Qt and create GUI applications with ease. It provides a wide range of modules that cover GUI programming, multimedia, network communication, database access, and more.
There are several versions of PyQt, the most notable ones being PyQt4 and PyQt5. The latter is the latest version and is compatible with both Python 2 and Python 3, while PyQt4 is mostly for Python 2 and has been deprecated in favor of PyQt5.
Additionally, there is also PyQt6, which is designed to work with Python 3 and provides enhancements over PyQt5. PyQt6 is mostly API-compatible with PyQt5, with some minor differences and improvements.
Why Choose PyQt?
Cross-Platform Compatibility
PyQt allows you to develop applications that can seamlessly run on various operating systems, such as Windows, macOS, and Linux, without significant code modifications.
Rich Set of Widgets
PyQt offers an extensive collection of widgets that serve as building blocks for your GUIs. These widgets include buttons, text boxes, sliders, tables, and more, enabling you to create complex interfaces effortlessly.
Event Handling
PyQt’s event-driven architecture lets you create responsive applications. You can easily handle user interactions, such as clicks and keystrokes, by connecting events to specific functions in your code.
Customization and Styling
PyQt allows you to customize the appearance of your applications by applying stylesheets. This feature ensures that your application not only functions well but also looks visually appealing.
Multimedia and Graphics
You can incorporate multimedia elements, such as images, audio, and video, into your PyQt applications. The framework also supports advanced graphics rendering for data visualization.
Internationalization (i18n) and Localization (l10n)
PyQt simplifies the process of making your application accessible to a global audience by providing tools for language translation and localization.
PyQT Installation
Before you start building PyQt applications, you need to have PyQt installed. To install PyQt, use the following pip command, replacing the version number with the specific version of PyQt you desire:
For PyQt6:
pip install PyQt6
For any other PyQt version, substitute the version number in place of “6” in the command above.
Make sure you have Python and pip installed on your system before running these commands. If you’re working with virtual environments, make sure to activate the virtual environment before installing PyQt.
Once you have PyQt installed, you can start using it to create GUI applications in Python.
Creating Your First PyQt Application
Let’s create a simple “Hello PyQt” application to demonstrate how easy it is to get started with PyQt.
import sys
from PyQt6.QtCore import Qt
from PyQt6.QtWidgets import QApplication, QMainWindow, QLabel
class AppWindow(QMainWindow):
def __init__(self, title, width, height, left=100, top=100):
super().__init__()
self.setWindowTitle(title)
# Set the window location and size
self.setGeometry(left, top, width, height)
self.initUI()
def initUI(self):
# Create a QLabel widget
label = QLabel("Hello, PyQt!")
# Set alignment
label.setAlignment(Qt.AlignmentFlag.AlignCenter)
# Add the QLabel widget to the main window
self.setCentralWidget(label)
def center(self):
# Get the geometry of the screen
screen = QApplication.primaryScreen().size()
# Calculate the center point of the screen
x = (screen.width() - self.width()) // 2
y = (screen.height() - self.height()) // 2
# Move the window to the center
self.move(x, y)
if __name__ == "__main__":
app = QApplication(sys.argv)
# Create new app window with title, and dimensions
window = AppWindow("Introduction to PyQT", 640, 480)
# Center app window on screen
window.center()
# show app window on screen
window.show()
sys.exit(app.exec())
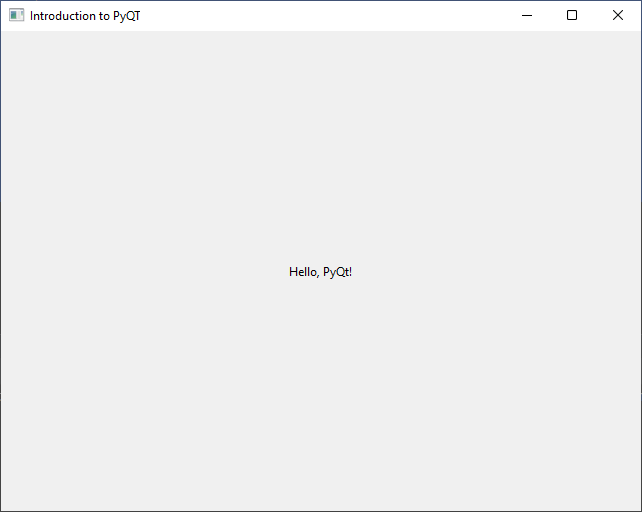
In this example, we import the necessary PyQt modules, create an application instance, set up a main window, add a label with text, and then start the application event loop.
Conclusion
PyQt is a fantastic tool for developing feature-rich and visually appealing GUI applications using Python. Its integration with the Qt framework empowers developers to create cross-platform applications that cater to a wide audience. In this article, we’ve only scratched the surface of what PyQt can do. As you delve deeper, you’ll discover its versatility and flexibility, enabling you to create applications that meet your unique requirements.
Whether you’re a beginner in GUI programming or an experienced developer looking to streamline your application development process, PyQt is a valuable asset that can significantly enhance your productivity and create engaging user experiences. So, why not dive into the world of PyQt and unlock a new realm of possibilities for your Python applications?
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!