JavaFX is a powerful framework for building desktop applications with Java. It provides a wide range of UI controls that enable developers to create user-friendly and feature-rich applications. This article explores one such control called the ColorPicker, which allows users to choose colors interactively. We’ll cover everything you need to know about JavaFX ColorPicker, its usage, customization, and handling of color change events.
What is a ColorPicker?
A ColorPicker is a JavaFX control that provides a user-friendly way to select colors. It displays a combination of a color preview box and a drop-down color palette or color chooser dialog, depending on the user’s operating system.
Creating a Simple ColorPicker
Let’s start by creating a basic JavaFX application with a ColorPicker to see how it works. We will build a simple program that changes the background color of the application based on the color selected by the user.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ColorPicker;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create a new ColorPicker
ColorPicker colorPicker = new ColorPicker();
parent.getChildren().add(colorPicker);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX ColorPicker");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
We create a ColorPicker instance and add it to a StackPane, which is then displayed in the application’s main scene.
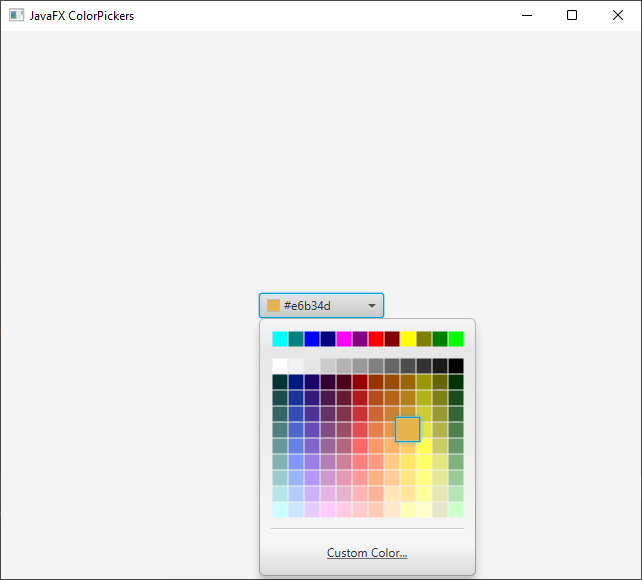
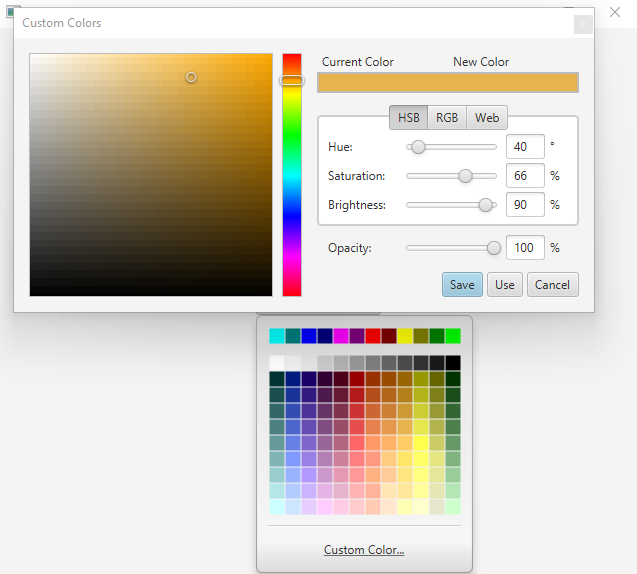
Handling Color Selection
Now that we have a basic ColorPicker, let’s enhance our application by adding a functionality that changes the background color of the scene when a new color is selected.
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.scene.Scene;
import javafx.scene.control.ColorPicker;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create a new ColorPicker
ColorPicker colorPicker = new ColorPicker();
colorPicker.setOnAction(this::onColorPicked);
parent.getChildren().add(colorPicker);
}
private void onColorPicked(ActionEvent actionEvent) {
// Gets the color picker
ColorPicker colorPicker = (ColorPicker) actionEvent.getSource();
// Gets the selected color
Color selectedColor = colorPicker.getValue();
// Convert the selected color to its RGB hexadecimal representation
String RGB = toRGBCode(selectedColor);
this.parent.setStyle(String.format("-fx-background-color: %s;", RGB));
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ColorPickers");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
private String toRGBCode(Color color) {
return String.format(
"#%02X%02X%02X",
(int) (color.getRed() * 255),
(int) (color.getGreen() * 255),
(int) (color.getBlue() * 255)
);
}
}
In the modified code, we set an onAction event handler on the ColorPicker, which is triggered when the user selects a new color. We extract the selected color using colorPicker.getValue(), convert it to its RGB hexadecimal representation using the toRGBCode() method, and then set it as the background color of the scene parent pane.
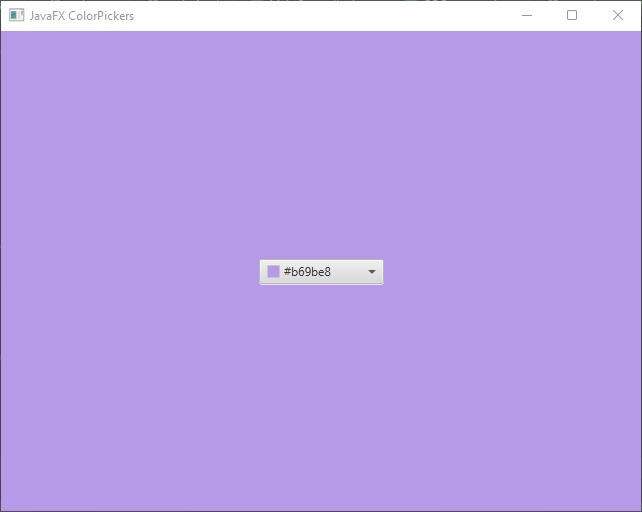
Customizing the ColorPicker
JavaFX allows you to customize the appearance and behavior of the ColorPicker to match the theme of your application. You can change the default colors, customize the text, change the prompt text, and much more. Here’s a quick example of how to customize the ColorPicker:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ColorPicker;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create a new ColorPicker
ColorPicker colorPicker = new ColorPicker();
// Set a custom width
colorPicker.setPrefWidth(300);
// Set a default color
colorPicker.setValue(Color.DARKGREEN);
parent.getChildren().add(colorPicker);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Set the stage title
stage.setTitle("JavaFX ColorPickers");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
In this example, we set a preferred width (300px) for the ColorPicker and set the default color (darkgreen) to be displayed when the application starts.
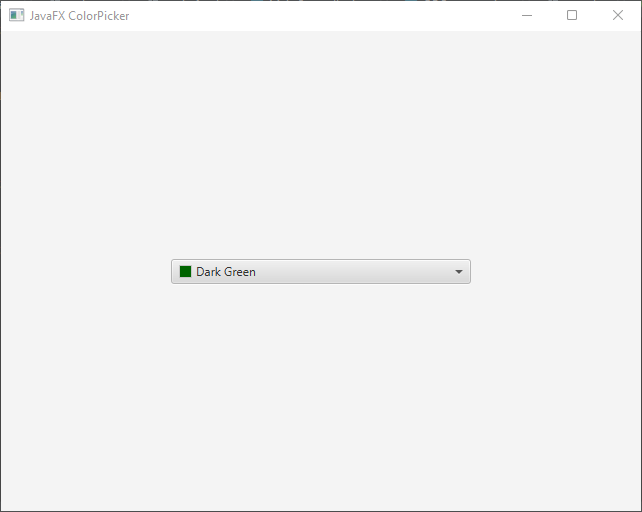
Conclusion
The ColorPicker is a valuable JavaFX control that allows users to select colors with ease. In this article, we explored the usage of the ColorPicker and learned how to handle color selection events. Additionally, we saw how to customize the ColorPicker to fit the requirements of your JavaFX application.
JavaFX’s ColorPicker is just one of the many exciting features this powerful library offers. Experiment with it, combine it with other controls, and unleash your creativity to build fantastic desktop applications with JavaFX.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!