JavaFX is a powerful framework for building cross-platform desktop applications. One of the essential components in any application is a date picker, which allows users to select dates conveniently. In this article, we will explore the JavaFX DatePicker, its features, and demonstrate how to use it with concise code examples.
Understanding the DatePicker
The DatePicker is a JavaFX control that enables users to select dates from a graphical calendar interface. It provides an intuitive way to pick a date without the need for manual input or parsing. The DatePicker class is part of the javafx.scene.control package and provides several methods and properties to customize its behavior.
Creating a Simple DatePicker
Let’s start by creating a basic DatePicker in JavaFX. Here’s the code snippet that demonstrates how to create and display a DatePicker in a JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
DatePicker datePicker = new DatePicker();
this.parent.getChildren().addAll(datePicker);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX DatePicker");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
In the above code, we create an instance of DatePicker and add it to a StackPane layout container. Then, we create a Scene and set it as the primary scene of the application window. Finally, we set the title, show the window, and launch the application.
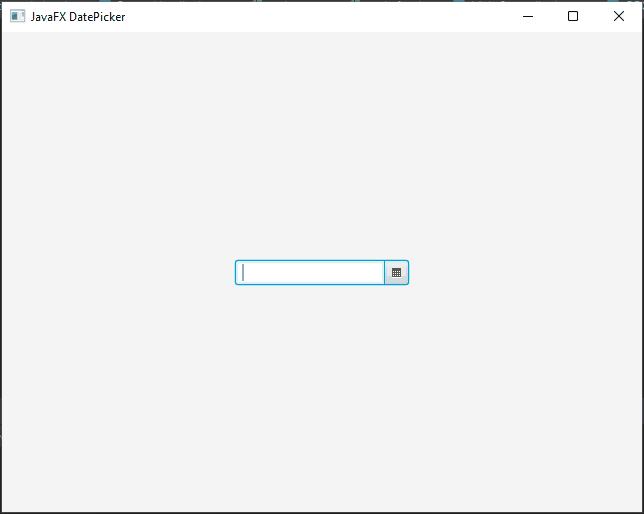
Setting an Initial Date
To set an initial date in the DatePicker, you can use the setValue() method. Here’s an example that sets the initial date to July 1, 2023:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import java.time.LocalDate;
import java.time.Month;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
DatePicker datePicker = new DatePicker();
datePicker.setValue(LocalDate.of(2023, Month.JULY, 1));
this.parent.getChildren().addAll(datePicker);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX DatePicker");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
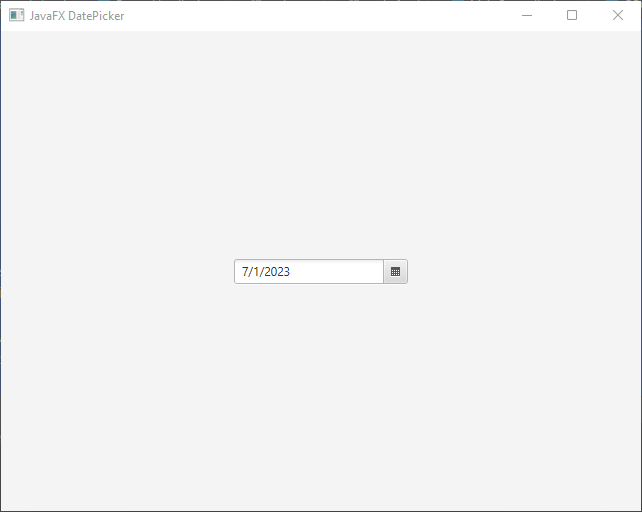
Handling Date Selection
To respond to date selection events, you can add an event handler to the DatePicker using the setOnAction() method or you can add an event listener using the valueProperty(). The event handler will be triggered whenever the user selects a new date.
Using setOnAction Method
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
DatePicker datePicker = new DatePicker();
datePicker.setOnAction(event -> {
LocalDate selectedDate = datePicker.getValue();
System.out.println("Selected Date: " + selectedDate);
});
this.parent.getChildren().addAll(datePicker);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX DatePicker");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
Using The valueProperty
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
DatePicker datePicker = new DatePicker();
datePicker.valueProperty().addListener((observable, oldValue, newValue) -> {
System.out.println("Selected Date: " + newValue);
});
this.parent.getChildren().addAll(datePicker);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX DatePicker");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
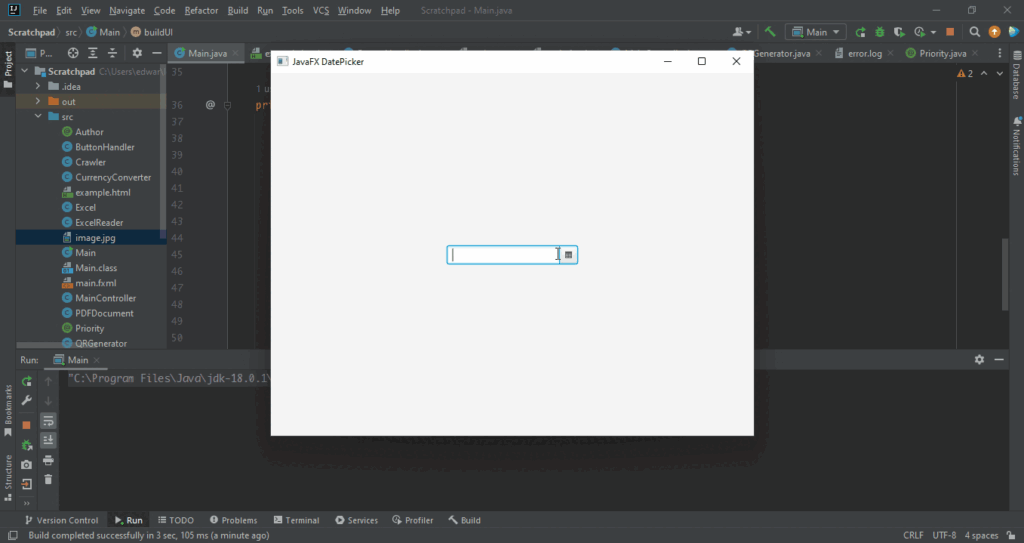
Restricting Date Range
You can also set a specific date range to restrict the selectable dates in the DatePicker. Use the setDayCellFactory method to customize the date cells and apply your logic for date range validation.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DateCell;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import java.time.LocalDate;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
DatePicker datePicker = new DatePicker();
datePicker.setDayCellFactory(picker -> new DateCell() {
@Override
public void updateItem(LocalDate date, boolean empty) {
super.updateItem(date, empty);
LocalDate minDate = LocalDate.now().minusDays(5);
LocalDate maxDate = LocalDate.now().plusDays(5);
setDisable(date.isBefore(minDate) || date.isAfter(maxDate));
}
});
this.parent.getChildren().addAll(datePicker);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX DatePicker");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
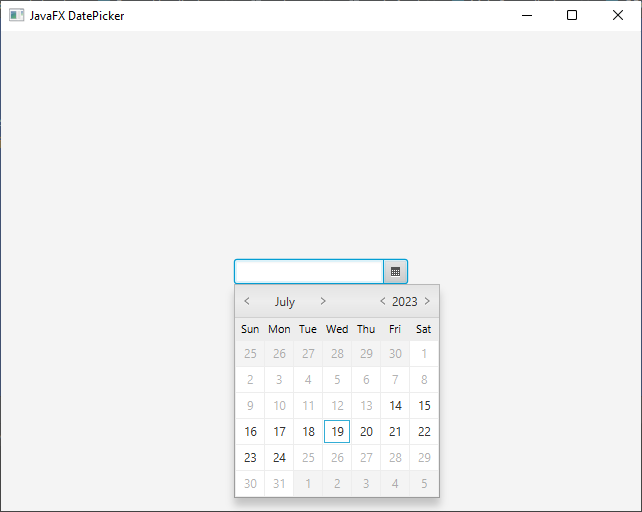
Customizing the DatePicker
The DatePicker provides several properties and methods to customize its appearance and behavior. Some of the commonly used ones include:
The setEditable(boolean editable) Method
Enables or disables manual input of dates.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
DatePicker datePicker = new DatePicker();
datePicker.setEditable(false);
this.parent.getChildren().addAll(datePicker);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX DatePicker");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
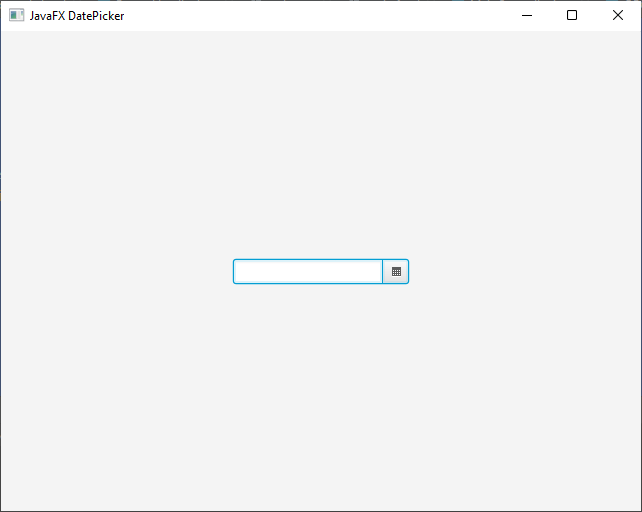
The setShowWeekNumbers(boolean showWeekNumbers) Method
Displays week numbers in the calendar.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final StackPane parent = new StackPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
DatePicker datePicker = new DatePicker();
datePicker.setShowWeekNumbers(true);
this.parent.getChildren().addAll(datePicker);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX DatePicker");
// Set the stage scene
stage.setScene(scene);
// Center stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
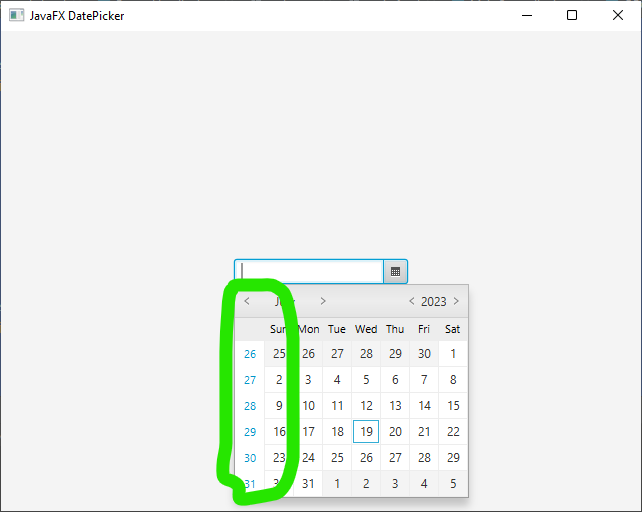
Conclusion
The JavaFX DatePicker is a versatile control that simplifies date selection in desktop applications. By utilizing its features and methods, you can enhance user experience and improve the functionality of your JavaFX application. In this article, we explored the basics of using the DatePicker, setting initial dates, handling date selection events, and customizing its appearance.
Remember to check out the official JavaFX documentation for further details and advanced usage of the DatePicker.
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!