In the world of modern software development, creating user interfaces that are both visually appealing and responsive across various devices is of paramount importance. JavaFX, a powerful framework for building desktop and mobile applications, offers a variety of layout managers to help developers achieve this goal. Among them, the JavaFX FlowPane stands out as an excellent choice for designing flexible and responsive user interfaces.
In this article, we will delve into the features of the JavaFX FlowPane layout manager and explore how it can be used to create dynamic and adaptive interfaces. We’ll provide comprehensive code examples to illustrate the concepts discussed.
Introducing FlowPane
The FlowPane is a layout manager in JavaFX that arranges its child nodes in a flow-like manner, either horizontally or vertically. This layout dynamically adjusts the positioning and sizing of its child nodes based on available space. This makes it ideal for scenarios where you want the UI elements to adapt gracefully to changes in window size or screen orientation.
Key Features of FlowPane
- Automatic Wrapping: One of the standout features of the FlowPane is its ability to automatically wrap child nodes to the next line or column when the available space is insufficient. This allows you to design interfaces that gracefully adjust to various screen sizes and orientations.
- Alignment and Margins: You can specify the alignment of child nodes within the FlowPane, both vertically and horizontally. Additionally, you can set margins around each child node to control spacing.
- Resizability: As the parent container (window or another layout) is resized, the FlowPane dynamically redistributes the child nodes, maintaining the flow-like arrangement.
Creating a Basic FlowPane
Let’s start with a simple example to demonstrate the usage of FlowPane. We’ll create a JavaFX application with a FlowPane containing a set of buttons that will adapt their positions as the window is resized.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final FlowPane parent = new FlowPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Horizontal gap between nodes
this.parent.setHgap(10);
// Vertical gap between nodes
this.parent.setVgap(10);
// Create and add UI elements to the FlowPane
for (int i = 1; i <= 10; i++) {
Button button = new Button("Button " + i);
this.parent.getChildren().add(button);
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FlowPane: Designing Responsive Interfaces");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
In this example, we’ve created a simple JavaFX application with a FlowPane containing ten buttons. The setHgap and setVgap methods set the horizontal and vertical gaps between the buttons. As you resize the window, you’ll notice that the buttons adjust their positions to fit the available space while maintaining the defined gaps.
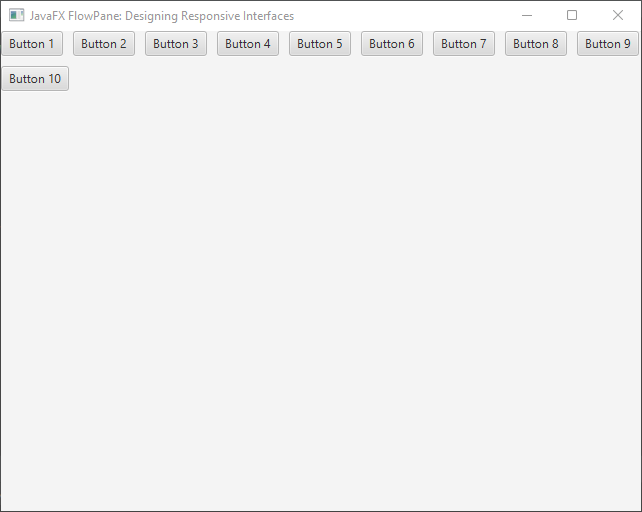
Customizing FlowPane Behavior
The FlowPane offers various customization options to control its behavior and appearance. Here are a few examples:
Orientation and Wrapping
You can set the flow direction using the setOrientation method:
import javafx.application.Application;
import javafx.geometry.Orientation;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final FlowPane parent = new FlowPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Horizontal gap between nodes
this.parent.setHgap(10);
// Vertical gap between nodes
this.parent.setVgap(10);
// Create and add UI elements to the FlowPane
for (int i = 1; i <= 10; i++) {
Button button = new Button("Button " + i);
this.parent.getChildren().add(button);
}
// Flow vertically
this.parent.setOrientation(Orientation.VERTICAL);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FlowPane: Designing Responsive Interfaces");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
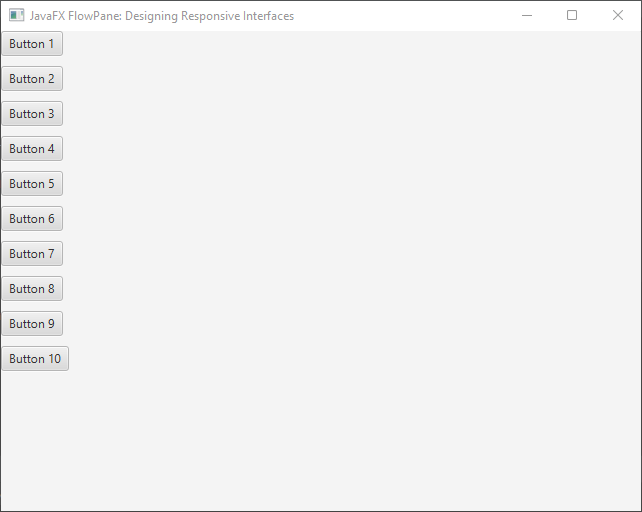
Additionally, you can enable wrapping to create multiple rows or columns:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final FlowPane parent = new FlowPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Horizontal gap between nodes
this.parent.setHgap(10);
// Vertical gap between nodes
this.parent.setVgap(10);
// Create and add UI elements to the FlowPane
for (int i = 1; i <= 10; i++) {
Button button = new Button("Button " + i);
this.parent.getChildren().add(button);
}
// Preferred width for wrapping
this.parent.setPrefWrapLength(200);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FlowPane: Designing Responsive Interfaces");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
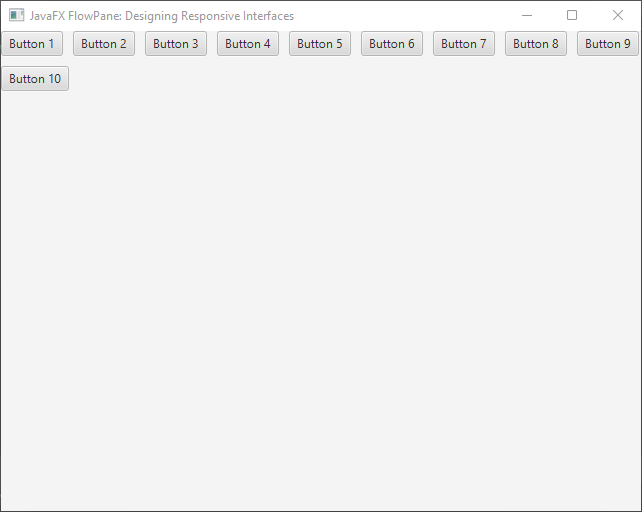
Alignment and Margins
You can align child nodes within the FlowPane using alignment properties:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final FlowPane parent = new FlowPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Horizontal gap between nodes
this.parent.setHgap(10);
// Vertical gap between nodes
this.parent.setVgap(10);
// Create and add UI elements to the FlowPane
for (int i = 1; i <= 10; i++) {
Button button = new Button("Button " + i);
this.parent.getChildren().add(button);
}
// Align children at the center
this.parent.setAlignment(Pos.CENTER);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FlowPane: Designing Responsive Interfaces");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
Specify the horizontal and vertical alignment of individual columns and rows, using the setColumnHalignment(HPos value) and setRowValignment(VPos value) methods, respectively.
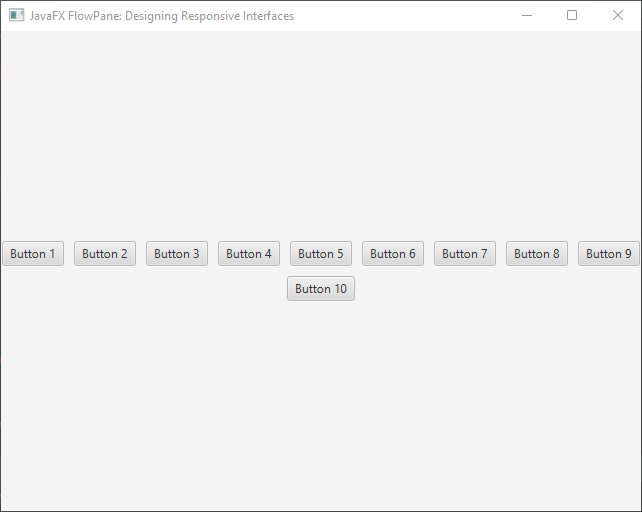
To add margins around child nodes, use the setMargin method:
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private final FlowPane parent = new FlowPane();
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Horizontal gap between nodes
this.parent.setHgap(10);
// Vertical gap between nodes
this.parent.setVgap(10);
// Create and add UI elements to the FlowPane
for (int i = 1; i <= 10; i++) {
Button button = new Button("Button " + i);
this.parent.getChildren().add(button);
// Set a margin of 10 pixels
FlowPane.setMargin(button, new Insets(10));
}
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("JavaFX FlowPane: Designing Responsive Interfaces");
// Set the stage scene
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
}
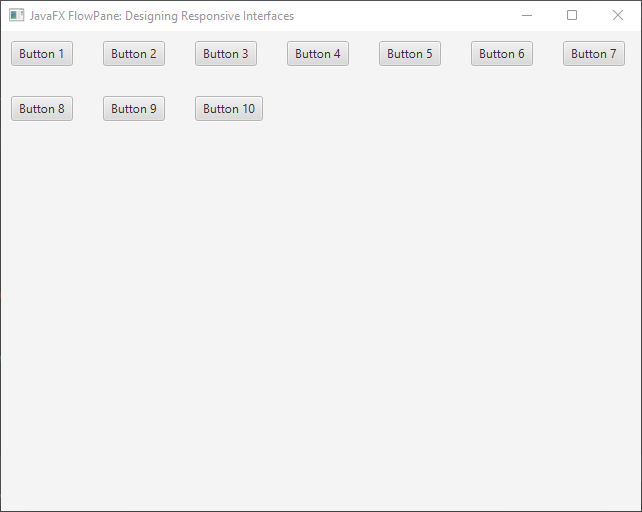
Conclusion
The FlowPane layout manager in JavaFX offers a flexible and responsive way to arrange UI elements, making it suitable for various design scenarios. Its ability to automatically wrap and reflow child nodes based on available space ensures that your application’s UI will adapt gracefully to different screen sizes and orientations. By leveraging the power of FlowPane, developers can create visually appealing and user-friendly interfaces that enhance the overall user experience.
Sources:
I hope you found this code informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!