In programming, user input means asking the person using your program to type something in—like their name, age, or favorite animal. Instead of the program doing everything on its own, it lets the user join in and give answers.
In Python, collecting user input is super helpful when you want your program to react to people. For example, you can ask the user for their name and then greet them, or ask for two numbers and add them together. With input, your program becomes more interactive and fun.
Using the input()
Function
In Python, the main way to get information from the user is by using the input()
function. When Python sees input()
, it pauses and waits for the user to type something and press Enter. Whatever they type is saved as a string (which just means text).
Here’s a simple example that asks the user for their name and says hello:
name = input("What's your name? ")
print("Hello, " + name + "!")
When you run this, the program will show the message "What's your name?"
, wait for the user to type something, and then greet them using whatever they typed.
Prompting the User with a Message
The input()
function becomes more helpful when you include a message that tells the user what to type. This message goes inside the parentheses and should be a string—usually written inside quotes.
For example:
name = input("What's your name? ")
print("Nice to meet you, " + name + "!")
This line tells the user exactly what the program is asking for. Clear prompts make your programs easier and more fun to use.

Converting Input to Other Data Types
By default, the input()
function always gives you a string—even if the user types a number. That means if you want to do math or logic with the input, you need to change it into the right data type.
Convert to Integer
Use int()
to change the input into a whole number:
age = int(input("How old are you? "))
print("Next year, you’ll be", age + 1)
Convert to Float
Use float()
if the number can have a decimal point:
height = float(input("Enter your height in meters: "))
print("You are", height, "meters tall.")
Use Input for Yes/No Questions
For simple true/false questions, you can use conditions like this:
answer = input("Do you like pizza? (yes/no): ")
if answer.lower() == "yes":
print("Yum! Pizza is great.")
else:
print("That's okay. More for me!")
Here, lower()
helps catch answers like “Yes”, “YES”, or “yes” by making everything lowercase first.
Using input()
with Math or Logic
You can use input()
to ask the user for numbers, but remember: input always gives you a string. If you want to do math, you must convert the input first.
Here’s an example where the program asks for two numbers and adds them:
num1 = int(input("Enter the first number: "))
num2 = int(input("Enter the second number: "))
total = num1 + num2
print("The total is:", total)
In this example, both inputs are turned into integers using int()
before the addition. If you forget to convert, Python will try to add strings instead of numbers, which won’t work the way you expect.
Getting Multiple Inputs
Sometimes you want the user to enter more than one value at once. You can do this by using the split()
function, which breaks the input into parts.
Here’s how to ask for two numbers in one line:
a, b = input("Enter two numbers: ").split()
print("You entered:", a, "and", b)
By default, split()
separates the input by spaces. So if the user types 3 5
, a
becomes "3"
and b
becomes "5"
.

If you want to do math with those values, convert them first. You can use map()
with int()
or float()
to do that quickly:
a, b = map(int, input("Enter two numbers: ").split())
print("Their sum is:", a + b)
This version turns both inputs into integers right away, so you can use them in calculations.

Using input()
in Loops
You can use input()
inside a loop to keep asking the user for something until they give the right answer or type something specific. This makes your program more interactive and flexible.
Here’s an example where the program keeps asking until the user types "exit"
:
command = ""
while command != "exit":
command = input("Type something (or 'exit' to quit): ")
print("You typed:", command)
In this code, the while
loop runs again and again until the user types "exit"
. Inside the loop, input()
asks the user for a new command each time.
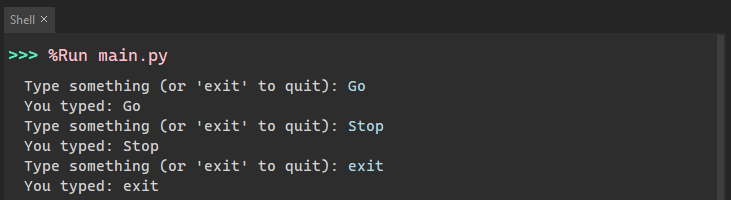
Using input()
in Functions
You can also use input()
inside a function. This is useful when you want to reuse the same input logic in different parts of your program.
Here’s an example of a function that asks the user for their favorite animal:
def ask_favorite_animal():
animal = input("What's your favorite animal? ")
return animal
chosen_animal = ask_favorite_animal()
print("Wow! I like", chosen_animal, "too!")
The ask_favorite_animal()
function uses input()
to get the user’s answer and then returns it. Later, you can use the result in other parts of your program. Functions like this help keep your code clean and organized.
Basic Input Validation
Sometimes, users might type something you don’t expect. To make your program safer and more reliable, you can check the input before using it. This is called input validation.
Here’s a simple example that checks if the user entered a number greater than 0:
number = int(input("Enter a number greater than 0: "))
if number > 0:
print("Thanks! That’s a good number.")
else:
print("Oops! Please enter a number greater than 0.")
This helps your program respond kindly when the input isn’t what you want. While input validation can get more advanced, even a small check like this can make a big difference.
Adding Exception Handling
But wait — what if someone types a word instead of a number? That would cause an error and might crash the program! To stop that from happening, we can use something called exception handling. It catches errors and lets you respond nicely.
Let’s improve the previous example by adding exception handling using try
and except
:
try:
number = int(input("Enter a number greater than 0: "))
if number > 0:
print("Thanks! That’s a good number.")
else:
print("Oops! Please enter a number greater than 0.")
except ValueError:
print("Uh-oh! That doesn’t look like a number. Please try again.")
Now, if someone types “cat” or “pizza” instead of a number, the program won’t crash. Instead, it shows a friendly message. This makes your program even more helpful and strong!
Conclusion
In this article, we learned how to use Python’s input()
function to get information from users. We covered the basics of asking for input, converting that input into different data types like integers and floats, and even handling multiple inputs at once. We also saw how input()
can be used in loops and functions to create more interactive and reusable programs.