JavaFX is a popular framework for creating rich, interactive graphical user interfaces in Java applications. One of its key features is the Canvas API, which allows developers to draw shapes, lines, and images directly onto a canvas. In this article, we will focus on drawing lines in JavaFX Canvas and provide you with full code examples to get you started.
Drawing Lines on the Canvas
To draw lines on the Canvas, you’ll need to get the GraphicsContext from the canvas and use it to draw lines. Here’s an example of how to draw a straight line:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set a stroke color, and width
gc.setStroke(Color.BLUE);
gc.setLineWidth(2.0);
// Draw a line from (50, 50) to (350, 350)
gc.strokeLine(50, 50, 350, 350);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Lines in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this code snippet, we first obtain the GraphicsContext for the canvas. We set the line color to blue and the line width to 2.0 pixels. Then, we use the strokeLine method to draw a line from point (50, 50) to point (350, 350) on the canvas.
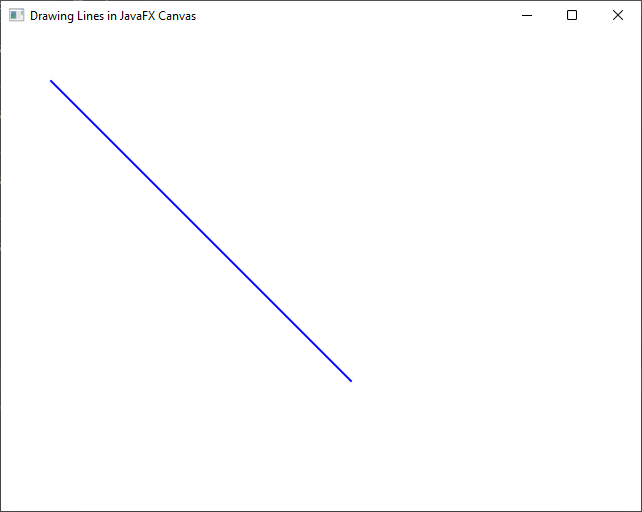
Drawing Multiple Lines
Drawing a single line is useful, but most applications require drawing multiple lines or more complex shapes. You can use loops and arrays to achieve this. Here’s an example of drawing multiple lines using a loop:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set a stroke color, and width
gc.setStroke(Color.RED);
gc.setLineWidth(1.0);
int numLines = 10;
double spacing = 30.0;
// Draw lines
for (int i = 0; i < numLines; i++) {
double startX = 20.0;
double startY = 20.0 + (i * spacing);
double endX = 380.0;
double endY = 20.0 + (i * spacing);
// Draw a line
gc.strokeLine(startX, startY, endX, endY);
}
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Lines in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we use a loop to draw ten horizontal lines with a spacing of 30 pixels between each line.
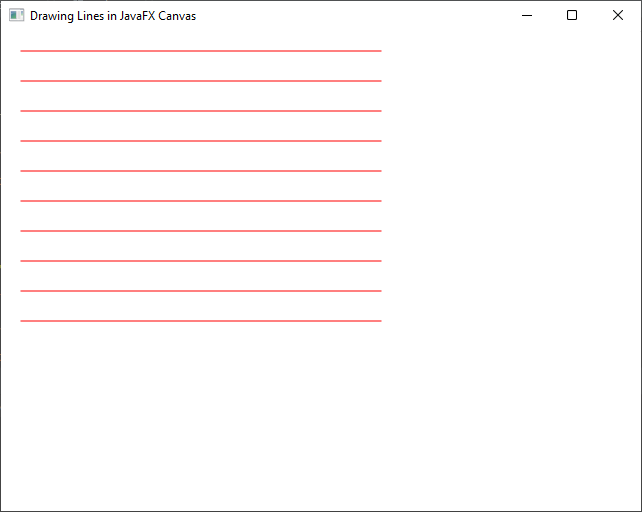
Line Styles
In JavaFX, you can create various line styles by customizing the stroke and stroke-dash-array properties of the GraphicsContext used to draw lines on a canvas. Here are some common line styles you can achieve:
Solid Line
A solid line is the default line style. To create a solid line, you don’t need to set any special properties since it’s the default behavior.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the line color
gc.setStroke(Color.BLACK);
// Set the line width
gc.setLineWidth(2.0);
gc.strokeLine(50, 50, 350, 50);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Lines in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
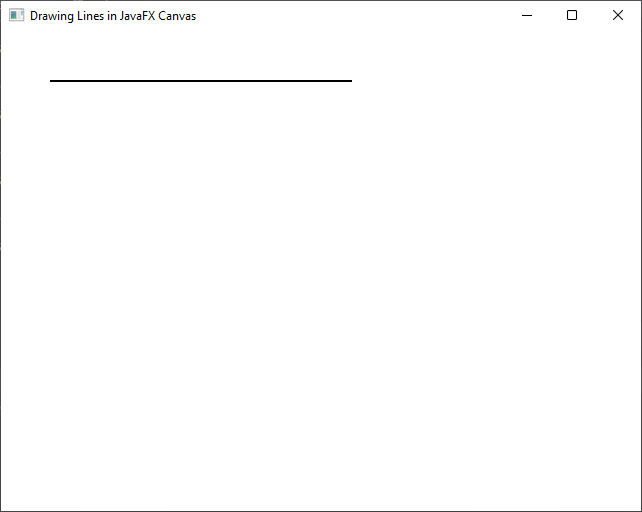
Dashed Line
Dashed lines consist of alternating segments of equal length. You can achieve this by setting the stroke-dash-array property with an array of values that specify the length of dashes and gaps.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the line color
gc.setStroke(Color.BLACK);
// Set the line width
gc.setLineWidth(2.0);
// 10 units of dash, 5 units of gap
gc.setLineDashes(10, 5);
gc.strokeLine(50, 100, 350, 100);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Lines in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
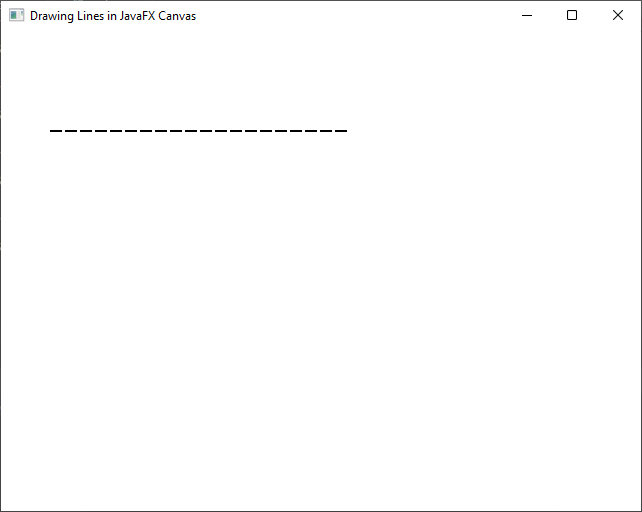
Dotted Line
Dotted lines consist of closely spaced dots. To create a dotted line, set the stroke-dash-array property with a small value for the dash length and an even smaller value for the gap.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the line color
gc.setStroke(Color.BLACK);
// Set the line width
gc.setLineWidth(2.0);
// 2 units of dash, effectively creating dots
gc.setLineDashes(2);
gc.strokeLine(50, 150, 350, 150);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Lines in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
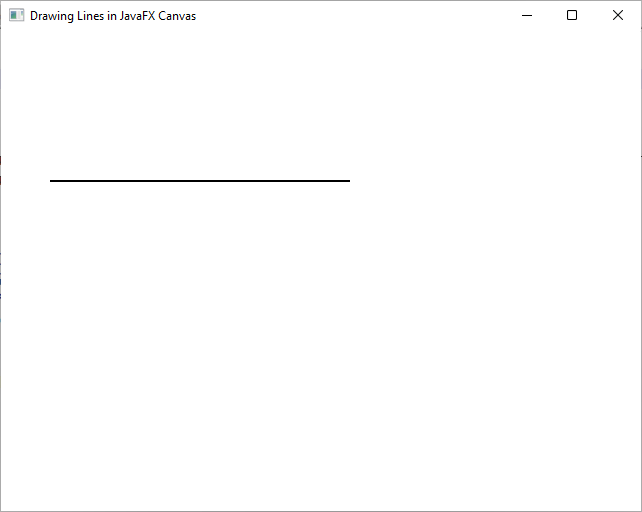
Custom Dash Patterns
You can create custom dash patterns by specifying multiple values in the stroke-dash-array property. For example, to create a line with alternating long and short dashes:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the line color
gc.setStroke(Color.BLACK);
// Set the line width
gc.setLineWidth(2.0);
// 15 units dash, 5 units gap, 5 units dash, 5 units gap, and so on
gc.setLineDashes(15, 5, 5, 5);
gc.strokeLine(50, 200, 350, 200);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Lines in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
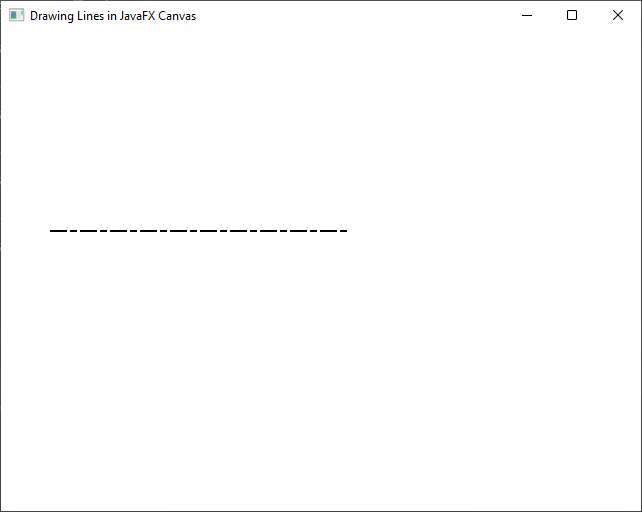
Changing Line Cap Style
You can also customize the line cap style using the setLineCap method of the GraphicsContext. The default is SQUARE, but you can set it to BUTT or ROUND for different cap styles.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.StrokeLineCap;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the line color
gc.setStroke(Color.BLACK);
// Set the line width
gc.setLineWidth(10.0);
// BUTT, ROUND, or SQUARE
gc.setLineCap(StrokeLineCap.ROUND);
gc.strokeLine(50, 250, 350, 250);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Lines in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
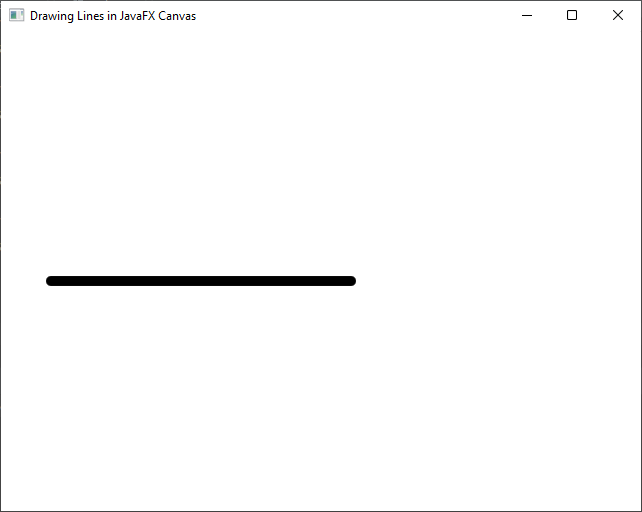
Experiment with these line styles and properties to create the visual effects you desire in your JavaFX applications. By combining different stroke colors, widths, dash patterns, and cap styles, you can achieve a wide range of line styles for your graphics.
Conclusion
Drawing lines in JavaFX Canvas is a fundamental skill for creating custom graphics and interactive applications. With the provided code examples, you can start exploring the capabilities of the JavaFX Canvas API and create your own graphical masterpieces. Feel free to experiment with different colors, line styles, and shapes to enhance your JavaFX applications. Remember to check the JavaFX Canvas Documentation for more.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!