JavaFX is a powerful framework for creating rich, interactive graphical user interfaces in Java applications. One of its key features is the Canvas class, which allows you to draw shapes, lines, and images directly onto a graphical surface. In this article, we will explore how to draw ovals in a JavaFX Canvas.
Drawing Ovals
Drawing ovals in JavaFX Canvas is straightforward. You can use the fillOval and strokeOval methods of the GraphicsContext class to draw filled and outlined ovals, respectively.
Filled Ovals
Here’s how you can draw a filled oval on the canvas:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the fill color
gc.setFill(Color.CORNFLOWERBLUE);
// Draw a filled oval
gc.fillOval(100, 100, 300, 150);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Ovals in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
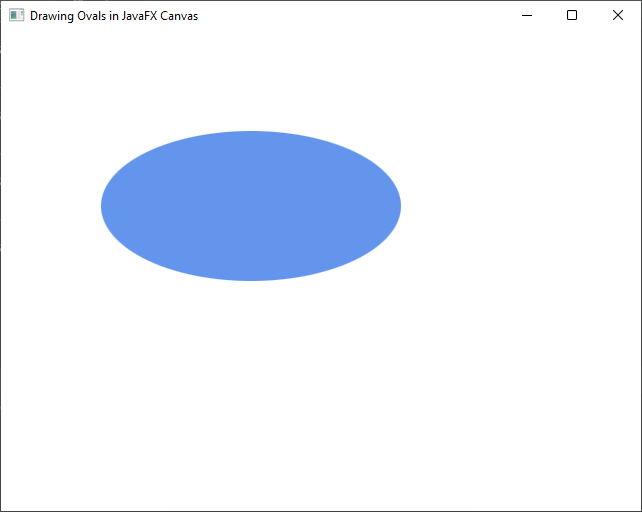
Stroked (Outlined) Ovals
To draw a stroked (outlined) oval, you can use the strokeOval() method:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(640, 480);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set the stroke color and width
gc.setStroke(Color.RED);
gc.setLineWidth(5);
// Draw an outlined oval
gc.strokeOval(100, 100, 300, 150);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Drawing Ovals in JavaFX Canvas");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
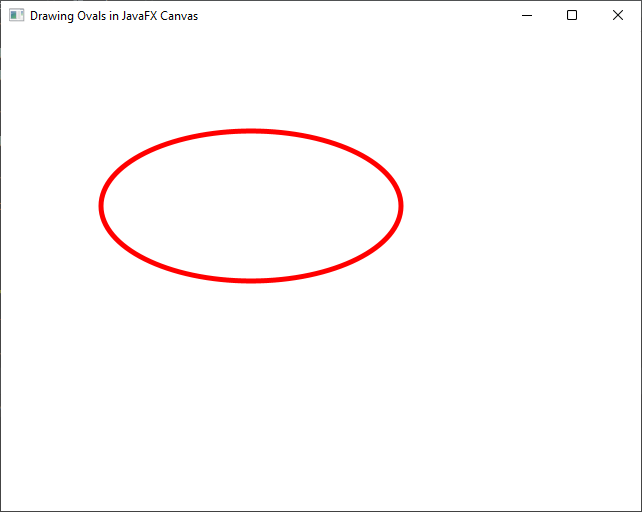
This code sets the stroke color to red, stroke width to 5px, and draws an oval with the same dimensions as the previous example.
Conclusion
Drawing ovals in JavaFX Canvas is straightforward and allows you to create visually appealing graphics in your Java applications. By utilizing the GraphicsContext class and its methods, you can easily customize the appearance of ovals to meet your specific design requirements. This article provided you with the essential knowledge and code examples to get started with oval drawing in JavaFX Canvas. Remember to check the JavaFX Canvas Documentation for more.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!