Accordions are a popular user interface (UI) element that helps organize content in a collapsible and interactive manner. In JavaFX, accordions provide a sleek and efficient way to display information within expandable sections. Whether you need to present FAQs, menu options, or categorized content, accordions offer a user-friendly solution.
Creating Accordions
In this code snippet, we explore how to use the JavaFX Accordion control to create a window with titled panes representing frequently asked questions (FAQs). Each titled pane acts as a header for a specific question, and when clicked, it expands to reveal the corresponding answer. This allows users to easily navigate through the FAQs and find the information they need. The Accordion control is highly customizable, allowing developers to style it according to their application’s design. By utilizing the Accordion control, JavaFX empowers developers to create user-friendly and informative applications with ease.
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
private Scene scene;
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// create the main content pane
VBox mainContent = new VBox(10);
mainContent.setPadding(new Insets(15));
// create the header label
Label header = new Label("FAQs");
header.setStyle("-fx-font-size: 20px; -fx-font-weight: bold");
// create the Accordion container
Accordion accordion = new Accordion();
// create nodes for the content sections
Label content1 = new Label("Content 1");
Label content2 = new Label("Content 2");
Label content3 = new Label("Content 3");
Label content4 = new Label("Content 4");
// create titled panes for the questions
TitledPane question1 = new TitledPane("Is JavaFX still used today?", content1);
TitledPane question2 = new TitledPane("What is JavaFX used for?", content2);
TitledPane question3 = new TitledPane("Should I use JavaFX or Swing?", content3);
TitledPane question4 = new TitledPane("Is JavaFX available for Android?", content4);
// add the titled panes to the accordion
accordion.getPanes().addAll(question1, question2, question3, question4);
// add the header and accordion to the main content pane
mainContent.getChildren().addAll(header, accordion);
// Create the layout manager using BorderPane
BorderPane layoutManager = new BorderPane(mainContent);
// create the scene with specified dimensions
this.scene = new Scene(layoutManager, 640, 480);
}
@Override
public void start(Stage stage) throws Exception {
// set the scene for the stage
stage.setScene(this.scene);
stage.setTitle("Getting Started with Accordions in JavaFX for Beginners");
stage.centerOnScreen();
stage.show();
}
}
When executed, the above program produces a window with an accordion, as depicted in the image below:
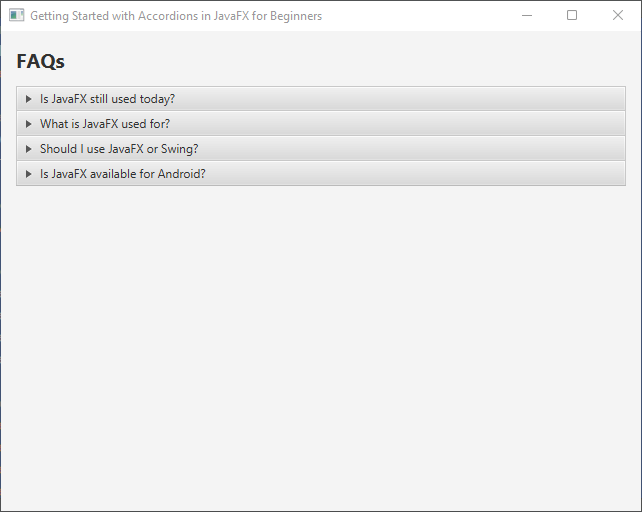
I hope you found this code informative and helpful in enhancing your JavaFX application. If you like this and would like to see more like it, make sure to subscribe to stay updated with my latest code snippets. 😊